Question
In java please, You will be implementing the Iterator Design Pattern to display a simple song list. Song Class: Holds the details of a song
In java please,
You will be implementing the Iterator Design Pattern to display a simple song list.
Song Class:
Holds the details of a song
toString method returns a string representation of the the name, artist, genre, and length of a song, as shown in the output.txt
Note: the Genre is implemented as an enum.
Album Class:
Holds an array of max 20 songs
Has a name associated with the Album
addSong -> Accepts the song details, creates a song and adds it to the array, returns true if the song was successfully added, and false otherwise
createIterator -> returns a new AlbumIterator using the Song array
AlbumIterator Class:
Implements the java.util Iterator
Constructor -> accepts an array of songs
next(): Returns the next song, make sure to error check
hasNext(): returns true if their is more songs to iterate over, and false otherwise
SongDriver.java:
package iterator;
import java.util.Iterator;
public class SongDriver {
public static void main(String[] args) {
Album myHits = new Album("Surfer Girl");
myHits.addSong("Surfer Girl", "The Beach Boys", 2.26, Genre.Pop);
myHits.addSong("Catch a Wave", "The Beach Boys", 2.07, Genre.Pop);
myHits.addSong("The Surfer Moon", "The Beach Boys", 2.11, Genre.Pop);
myHits.addSong("South Bay Surfer", "The Beach Boys", 1.45, Genre.Pop);
myHits.addSong("The Rocking Surfer", "The Beach Boys", 2, Genre.Pop);
myHits.addSong("Little Deuce Coupe", "The Beach Boys", 1.38, Genre.Pop);
myHits.addSong("In My Rooom", "The Beach Boys", 2.11, Genre.Pop);
myHits.addSong("Hawaii", "The Beach Boys", 1.59, Genre.Pop);
myHits.addSong("Surfers Rule", "The Beach Boys", 1.54, Genre.Pop);
myHits.addSong("Our Car Club", "The Beach Boys", 2.22, Genre.Pop);
myHits.addSong("Your Summer Dream", "The Beach Boys", 2.27, Genre.Pop);
myHits.addSong("Boogie Woodie", "The Beach Boys", 1.56, Genre.Pop);
AlbumIterator songIterator = myHits.createIterator();
System.out.println(" " + myHits.getName() + " Song List:");
while (songIterator.hasNext()) {
Song song = songIterator.next();
System.out.println("- " + song);
}
}
}
output.txt:
Surfer Girl Song List: - Surfer Girl by The Beach Boys category: Pop length: 2.26 min - Catch a Wave by The Beach Boys category: Pop length: 2.07 min - The Surfer Moon by The Beach Boys category: Pop length: 2.11 min - South Bay Surfer by The Beach Boys category: Pop length: 1.45 min - The Rocking Surfer by The Beach Boys category: Pop length: 2.0 min - Little Deuce Coupe by The Beach Boys category: Pop length: 1.38 min - In My Rooom by The Beach Boys category: Pop length: 2.11 min - Hawaii by The Beach Boys category: Pop length: 1.59 min - Surfers Rule by The Beach Boys category: Pop length: 1.54 min - Our Car Club by The Beach Boys category: Pop length: 2.22 min - Your Summer Dream by The Beach Boys category: Pop length: 2.27 min - Boogie Woodie by The Beach Boys category: Pop length: 1.56 min
UML:
THANK YOU!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
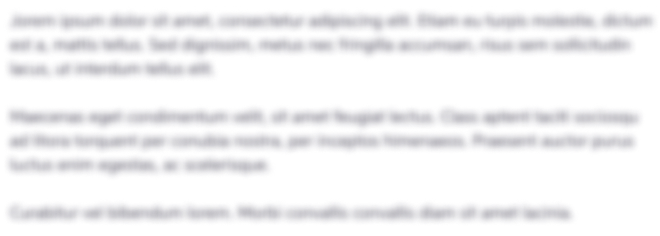
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started