Question
***********IN JAVA************* Write a method that sums the areas of all the geometric objects in an array. The method signature is: public static double totalArea(GeometricObject[]
***********IN JAVA*************
Write a method that sums the areas of all the geometric objects in an array. The method signature is:
public static double totalArea(GeometricObject[] list)
The test program will create an array of four geometicObjects (two Rectangle and two Circle ) and call the totalArea method to compute the sum of the areas.
Note:
The radius, width and height are positive values and default value is 1.
Input format:
The first number is the width of the first rectangle follow by
the height of the first rectangle follow by
the width of the second rectangle
the height of the second rectangle
the radius of the first circle
the radius of the second circle
*******Use the following class driver with no modifications to it!********
import java.util.*; import java.lang.*; import java.io.*;
abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated;
/** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; }
/** Return color */ public String getColor() { return color; }
/** Set a new color */ public void setColor(String color) { this.color = color; }
/** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; }
/** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; }
/** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; }
@Override public String toString() { return "created on " + dateCreated + " color: " + color + " and filled: " + filled; }
/** Abstract method getArea */ public abstract double getArea();
}
class Circle extends GeometricObject{ private double radius = 1; public Circle(){ } public Circle(double radius){ if(radius > 0) this.setRadius(radius); } /** Return radius */ public double getRadius() { return radius; }
/** Set a new radius */ public void setRadius(double radius) { if(radius > 0) this.radius = radius; } public double getArea(){ return this.radius * this.radius * Math.PI; } }
class Rectangle extends GeometricObject{ private double width = 1; private double height = 1; public Rectangle() { }
public Rectangle(double width, double height) { if(width>0 && height>0){ this.width = width; this.height = height; } }
/** Return width */ public double getWidth() { return width; }
/** Set a new width */ public void setWidth(double width) { this.width = width; }
/** Return height */ public double getHeight() { return height; } /** Set a new height */ public void setHeight(double height) { this.height = height; } public double getArea(){ return this.width * this.height; } }
**********HERE'S THE MAIN DRIVER, NO MODIFCATIONS HERE EITHER***********
class DriverTest{
public static void main(String args[]){
Scanner input = new Scanner(System.in);
double w1 = input.nextDouble();
double h1 = input.nextDouble();
double w2 = input.nextDouble();
double h2 = input.nextDouble();
double r1 = input.nextDouble();
double r2 = input.nextDouble();
GeometricObject[] list = new GeometricObject[4];
list[0] = new Rectangle(w1, h1);
list[1] = new Rectangle(w2, h2);;
list[2] = new Circle(r1);
list[3] = new Circle(r2);
System.out.printf("%.2f",HW5_P2.totalArea(list));
}
}
***********************************************************************************
---And use the following class that was refered to in main----
class HW5_P2{
public static double totalArea(GeometricObject[] list){
}
}
***********************************************************************************************************************
Leaving comments in the code would be much appreciated to help me understand the code more!
PLEASE follow assignment instructions as I am having trouble understanding everything completely!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
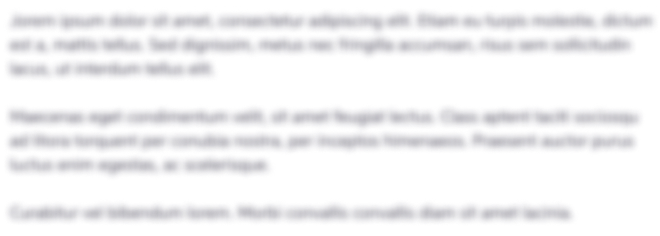
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started