Question
*** IN JAVA **** Write a program that will sort a basket of clothes into their proper drawers. If you were not aware you are
*** IN JAVA ****
Write a program that will sort a basket of clothes into their proper drawers. If you were not aware you are sort clothes by their type in this order:
Top Drawer Undergarments
Next Drawer Socks or Stockings
The Following Drawer Tops
The Subsequent Drawer Bottoms
The Cape Drawer Capes
Write a class called Clothing
Instance Variables
Type This can only be Undergarment, Socks, Stockings, Top, Bottom, and Cape
Color This can only be Brown, Red, Pink, Orange, Green, Blue, Purple, and Grey
Constructors
Default
Parameterized
Accessors and Mutators for the instance variables
Make sure to check for valid values in the mutator
Methods
toString: Takes in no parameters and returns a string with the Type and Color of the garment
equals: Takes an instance of Clothing as a parameters and returns true only if the parameters are equal
Next write a class called Dresser
Instance Variables
Clothes a 2D array where there are only 5 drawers, and each drawer can hold 10 items of clothing
Constructors
Just default that creates the 2D array
No Accessors or Mutators
Methods
add: Takes in an instance of Clothing as a parameter and returns nothing. The parameter is then sorted in their proper drawers by its type as mentioned above. If a drawer is full make sure to tell the user.
remove: Takes in an instance of Clothing as a parameter and returns nothing. This method searches for a piece of clothing, and if it exists it is removed (by setting that value to null).
print: This prints out every piece of clothing in the dresser
Finally write a class called DresserFrontEnd
Contains the main method
Prompts the user to add clothing, remove clothing, check what is in the dresser, or quit
Adding an item should prompt the user to enter the type and color
Removing should prompt the user to again enter the type and color they wish to remove
Printing shows the user what is in the dresser
Quit immediately halts the program
Example Output:
Welcome to the dresser!
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
1
Enter the type
It may be undergarment, socks, stockings, top, bottom, or cape
top
Enter a color
It may be brown, pink, orange, green, blue, purple, or grey
red
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
1
Enter the type
It may be undergarment, socks, stockings, top, bottom, or cape
cape
Enter a color
It may be brown, pink, orange, green, blue, purple, or grey
purple
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
1
Enter the type
It may be undergarment, socks, stockings, top, bottom, or cape
socks
Enter a color
It may be brown, pink, orange, green, blue, purple, or grey
grey
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
1
Enter the type
It may be undergarment, socks, stockings, top, bottom, or cape
cape
Enter a color
It may be brown, pink, orange, green, blue, purple, or grey
blue
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
1
Enter the type
It may be undergarment, socks, stockings, top, bottom, or cape
undergarment
Enter a color
It may be brown, pink, orange, green, blue, purple, or grey
pink
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
3
Drawer 0
undergarment pink
Drawer 1
socks grey
Drawer 2
top red
Drawer 3
Drawer 4
cape purple
cape blue
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
2
Enter the type
It may be undergarment, socks, stockings, top, bottom, or cape
socks
Enter a color
It may be brown, pink, orange, green, blue, purple, or grey
grey
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
3
Drawer 0
undergarment pink
Drawer 1
Drawer 2
top red
Drawer 3
Drawer 4
cape purple
cape blue
Enter 1: to add an item
Enter 2: to remove an item
Enter 3: to print out the dresser contents
Enter 9: to quit
9
Goodbye
Step by Step Solution
There are 3 Steps involved in it
Step: 1
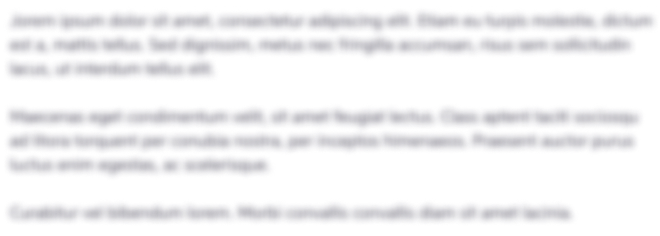
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started