Question
In Java, you will write a postfix calculator program. A postfix calculator uses postfix notation, in which the operator follows the operands. For example: 3
In Java, you will write a postfix calculator program. A postfix calculator uses postfix notation, in which the operator follows the operands.
For example:
3 4 * 4 5 + *
is the posfix notation for (3 * 4) * (4 + 5).
This is a program, not an ADT. If you want, all the code can be in the main() function.
Your program needs to store operands (which are just floating point numbers for this lab) in some appropriate collection type (a stack, queue, or bag). When it reads an operator (like '+' or '\') it must take the two most recent operands, perform the operation, and keep the result as the most recent operand.
(So as not to give it away, I will refer here to the stack, queue, or bag that you use as "the collection".)
Besides the usual arithmetic operators (+, -, *, /), your program needs to recognize two special operators: "sqrt" should take the most recent operand, compute its square root (using Math.sqrt() from the Java library), and add the result back to the collection. "print" should take the most recent operand from the collection, and print it using StdOut.println().
For the print function, just use StdOut.println(). That way, your output will be consistent with mine.
Your program should read strings from standard input and take the appropriate action until the input is exhausted.
So, for example, if the input is:
3 4 * 2 * 1 + sqrt print
your program should print:
5
Let's follow that in detail. Here is what your program should do with each string it reads in the above example:
string | action | |
3 | add to the collection | |
4 | add to the collection | |
* |
| |
2 | add to the collection | |
* |
| |
1 | add to the collection | |
+ | remove the 1 and 24, add them, add the result (25) to the collection | |
sqrt | remove the 25, compute the square root, and add the result (5) to the collection | |
remove the 5, and print it |
HERE is the program I have done so far :
public class PostfixCalc {
public static boolean isNumber(String s) {
try {
Double.parseDouble(s);
return true;
} catch (NumberFormatException e) {
return false;
}
}
public static void main(String[] args) {
/// CODE in here
{
Step by Step Solution
There are 3 Steps involved in it
Step: 1
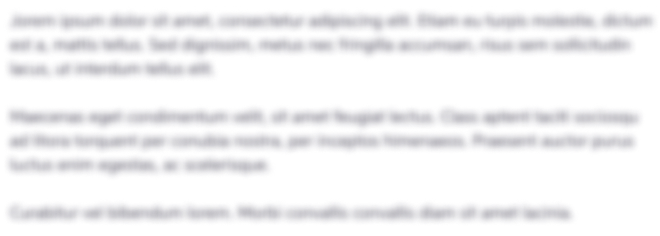
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started