Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In Python 3 Write a LinkedList class that has recursive implementations of the display , remove , contains , insert , and normal_list methods. You
In Python 3 Write a LinkedList class that has recursive implementations of the display, remove, contains, insert, and normal_list methods. You may use default arguments and/or helper functions.
The file must be named: LinkedList.py
Here is what I have for my code so far. The methods I need the recursive implementations for will be bolded:
class Node: """ Represents a node in a linked list (parent class) """ def __init__(self, data): self.data = data self.next = None class LinkedList: """ A linked list implementation of the List ADT (child class) """ def __init__(self): self.head = None def add(self, val): """ Adds a node containing val to the linked list """ current = Node(val) if self.head is None: # If the list is empty self.head = Node(val) # Inheritance function - takes functionality from the parent class Node and allows us # to add the value variable return elif current.next is None: # Reaches the end of the list current.next = Node(val) return else: self.add(current.next) # Adds the value to the linked list return # NEEDS RECURSIVE IMPLEMENTATION def insert(self, val, pos): """ Inserts a value into the linked list based on the number of the position """ if pos == 0: # If the position is zero, insert the new value as the new first element temp = self.head print("val:", self.head.data) self.head = Node(val) # Inheritance function - takes functionality from the parent class Node and allows us # to add the value variable self.head.next = temp else: # If the position is greater than zero, keep incrementing current position and insert it there or at the # end of the list if position > length of list current = self.head for _ in range(pos - 1): # _ is a throwaway variable - it just indicates that the loop variable is not # actually used if current.next is None: current.next = Node(val) return current = current.next temp = current.next current.next = Node(val) current.next.next = temp # NEEDS RECURSIVE IMPLEMENTATION def display(self): """ Prints out the values in the linked list """ current = self.head while current is not None: print(current.data, end=" ") current = current.next print() # NEEDS RECURSIVE IMPLEMENTATION def remove(self, val): """ Removes the node containing val from the linked list """ if self.head is None: # If the list is empty return if self.head.data == val: # If the node to remove is the head self.head = self.head.next else: current = self.head while current is not None and current.data != val: previous = current current = current.next if current is not None: # If we found the value in the list previous.next = current.next def is_empty(self): """ Returns True if the linked list is empty, returns False otherwise """ return self.head is None # NEEDS RECURSIVE IMPLEMENTATION def normal_list(self): """ Returns a list of results based on the linked list """ result = [] current = self.head while current is not None: result += [current.data] current = current.next return result # NEEDS RECURSIVE IMPLEMENTATION def contains(self, key): """ Returns True if that value is in the linked list, returns False otherwise """ if self.head is None: # If the list is empty return False current = self.head while current is not None: if current.data == key: return True current = current.next if current is None: return False def reverse(self): """ Reverses the order of the nodes in the linked list """ def reverse_recursive(cur, prev): """ Recursive helper function """ if not cur: # If the base case is met return prev # The return is the previous node # Otherwise nxt = cur.next cur.next = prev prev = cur cur = nxt return reverse_recursive(cur, prev) # Every time we call this function, it will update the state of current and previous as we go along in the # recursive calls to this function self.head = reverse_recursive(cur=self.head, prev=None)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
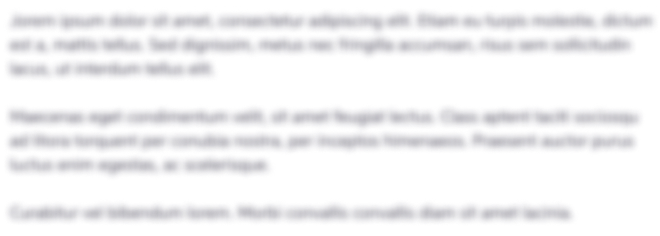
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started