Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In Python, define two functions called calc _ cirumference and calc _ area, which will calculate the circumference and the area of a circle, respectively,
In Python, define two functions called calccirumference and calcarea, which will calculate the circumference and the area of a circle, respectively, based on a radius passed in as an argument. Heres some model code that would calculate a diameter based on a radius argument:
def calcdiameterradius:
return radius;
And heres how we would call the calcdiameter function from main passing a radius of :
diam calcdiameter #variable diam will take on the value of
The circumference of a circle is x PI x the radius pi r The area of a circle is PI x the radius squared pi r For PI you can either hardcode or plug in math.pi To square the radius, you can use radius radius or math.powradius
Task : Define a Main Function
Define a main function using the def keyword. While youre at it call your main function using the recommended way discussed in this weeks reading and lecture materials ie using an if statement to test the files name variable Example:
if namemain:
main
Task : Calculate the Number of Pizzas
In order to calculate the number of pizzas to make, follow these steps below. You may create any variables you need at any time in your program.
Ask the user for the diameter of the pizza and the number of expected guests see sample output next page
Divide the diameter in half to get the radius, and then get the pizza area by passing the radius to your calcarea function.
Calculate the slices per one pizza by dividing the area by
Calculate the number of slices needed for the party by multiplying the number of guests by
Finally, calculate the number of pizzas by dividing the number of slices needed by the number of slices per pizza. Since the caterer does not make fractional pizzas, use the math.ceil function to round up to the next highest integer, to ensure they make enough for the party. Example:
numPizzas math.ceilslicesNeeded slicesPerPizza
Task : Calculate Cups of Cheese Needed for the Crust
To calculate the cups needed to sufficiently stuff the crust:
Get the pizzas circumference by passing the radius to your calccircumference function.
Calculate the cups of cheese by dividing the circumference by because there are ounces in a cup, and one ounce of cheese will stuff linear inches of crust and then multiply that by the number of pizzas. As in task we have to use math.ceil to round up to make sure we have enough. Example:
cupsCheese math.ceilpizzaCirc numPizzas
Step by Step Solution
There are 3 Steps involved in it
Step: 1
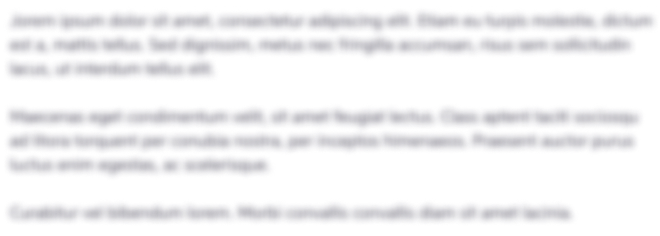
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started