Question
IN SCALA , please complete help me finish the following code. object storage { // EXERCISE 1: Complete the following definition, so that constant5 is
IN SCALA , please complete help me finish the following code.
object storage {
// EXERCISE 1: Complete the following definition, so that "constant5" is a function that returns 5
// whenever it is invoked.
val constant5 : () => Int = {
// TODO: Complete the definition.
null
}
// EXERCISE 2: Complete the following definition, so that "constant" is a function that when
// invoked with integer n returns a function that returns n whenever it is invoked.
val constant : Int => () => Int = {
// TODO: Complete the definition.
null
}
// EXERCISE 3: Complete the following definition, so that "counter0" is a (stateful) function that
// returns 0 when it is first invoked, then 1, then 2, etc.
// REMEMBER: you can use "var" but everything you add has to be inside the "{...}" body of "counter0".
// This rule applies throughout this assignment.
val counter0 : () => Int = {
// TODO: Complete the definition.
null
}
// EXERCISE 4: Complete the following definition, so that "counter" is a (stateless) function that
// when invoked with integer n returns a (stateful) function that returns n when it is first
// invoked, then n+1, then n+2, etc. The counters must be independent, i.e., running "counter (0)"
// twice should yield two functions that do not interfere with one another's state.
val counter : Int => () => Int = {
// TODO: Complete the definition.
null
}
// EXERCISE 5: Complete the following definition, so that "getAndSet" is a (stateless) function
// that when invoked with integer n returns a pair of functions (that share state) that allow
// reading and writing a var that is initialized with integer n. The first function in the pair
// should be the reader. The second function in the pair should be the writer. For example, the
// following expression should return 10: { val (get, set) = getAndSet (5); set (10); get () }
// Multiple calls to "getAndSet" should yield independent pairs, i.e., the first pair returned
// should not share any state with the second pair returned.
val getAndSet : Int => (() => Int, Int => Unit) = {
// TODO: Complete the definition.
null
}
// EXERCISE 6: Complete the following definition, so that "getAndSetSpy" is a (stateful) function
// that when invoked it returns a pair. The second component of the pair should behave like
// "getAndSet" above (with the exception noted next). The first component of the pair is a
// function that, when invoked, returns the total number of times that a "set" call has been made.
// That number should cover all calls to "set" made in all pairs created via "getAndSetSpy". That is,
// the total number is a piece of state shared all "set" functions created via "getAndSetSpy".
val getAndSetSpy : () => (() => Int, Int => (() => Int, Int => Unit)) = {
// TODO: Complete the definition.
null
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
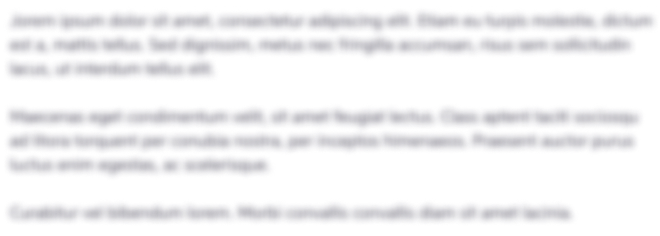
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started