Question
In the provided file SudokuChecker.java, complete the following methods: public static boolean checkRow(int row, byte[][] grid) public static boolean checkColumn(int column, byte[][] grid) public static
In the provided file SudokuChecker.java, complete the following methods:
public static boolean checkRow(int row, byte[][] grid) public static boolean checkColumn(int column, byte[][] grid) public static boolean checkSubregion(int region, byte[][] grid)
These methods are used in the provided check method that takes a 2-dimensional (99) array of numbers and determines if it represents a valid (solved) sudoku grid of numbers. A valid sudoku grid has the following properties:
each row contains all of the digits from 1 to 9, each column contains all of the digits from 1 to 9, each of the nine 2-dimensional 33 subgregions contain all of the digits 1 to 9.
For example, the following is a valid sudoku grid (with n = 3). The nine subregions are shown shaded.
5 | 3 | 4 | 6 | 7 | 8 | 9 | 1 | 2 |
6 | 7 | 2 | 1 | 9 | 5 | 3 | 4 | 8 |
1 | 9 | 8 | 3 | 4 | 2 | 5 | 6 | 7 |
8 | 5 | 9 | 7 | 6 | 1 | 4 | 2 | 3 |
4 | 2 | 6 | 8 | 5 | 3 | 7 | 9 | 1 |
7 | 1 | 3 | 9 | 2 | 4 | 8 | 5 | 6 |
9 | 6 | 1 | 5 | 3 | 7 | 2 | 8 | 4 |
2 | 8 | 7 | 4 | 1 | 9 | 6 | 3 | 5 |
3 | 4 | 5 | 2 | 8 | 6 | 1 | 7 | 9 |
The rows are labelled 0 to 8 (from top to bottom), the columns are labelled 0 to 8 (from left to right), and the subregions are labelled 0 to 8 as follows:
0 | 1 | 2 |
3 | 4 | 5 |
6 | 7 | 8 |
SUDOKU CHECKER
public class SudokuChecker{ /** sample valid game */ public static byte[][] example1 = new byte[][]{ {5,3,4,6,7,8,9,1,2}, {6,7,2,1,9,5,3,4,8}, {1,9,8,3,4,2,5,6,7}, {8,5,9,7,6,1,4,2,3}, {4,2,6,8,5,3,7,9,1}, {7,1,3,9,2,4,8,5,6}, {9,6,1,5,3,7,2,8,4}, {2,8,7,4,1,9,6,3,5}, {3,4,5,2,8,6,1,7,9}}; /** sample invalid game */ public static byte[][] example2 = new byte[][]{ {5,3,4,6,7,8,9,1,2}, {6,7,2,1,9,5,3,4,8}, {1,9,8,3,4,2,5,6,7}, {8,5,9,7,6,1,4,2,3}, {4,2,6,8,5,3,7,9,1}, {7,1,3,9,2,4,8,5,6}, {9,6,1,5,3,7,2,8,3}, {2,8,7,4,1,9,6,2,6}, {3,4,5,2,8,6,1,8,8}}; /** checks if row 'row' is OK in the grid */ public static boolean checkRow(int row, byte[][] grid){ return true; } /** checks if column 'col' is OK in the grid */ public static boolean checkColumn(int col, byte[][] grid){ return true; } /** checks if the subregion 'region' is OK in the grid */ public static boolean checkSubregion(int region, byte[][] grid){ return true; } public static boolean check(byte[][] grid){ for(int row=0; row<9; row+=1){ // check the rows if( !checkRow(row, grid) ){ return false; } } for(int col=0; col<9; col+=1){ // check the rows if( !checkColumn(col, grid) ){ return false; } } for(int subregion=0; subregion<9; subregion+=1){ // check the subregions if( !checkSubregion(subregion, grid) ){ return false; } } // if we get this far then we conclude that the grid // must be valid (because if it was not, we would have // returned false somewhere above) return true; } public static void main(String[] args){ System.out.print("exmample1 | expected output is true | actual output is "); System.out.println(check(example1)); System.out.print("exmample2 | expected output is false | actual output is "); System.out.println(check(example2)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
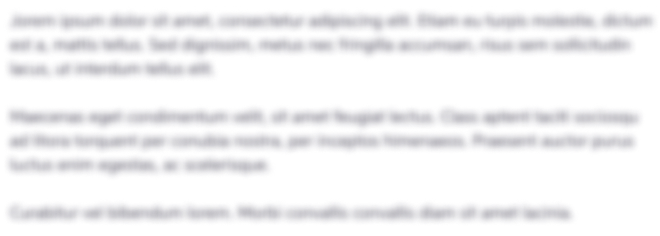
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started