Question
In this assignment, you are to explore the use and programming of some system calls through the creation of a simple shell program (you are
In this assignment, you are to explore the use and programming of some system calls through the creation of a simple shell program (you are writing a simple user-interface to the operating system).
In several cases, your shell program is implementing built-in commands. In others, it is to use exec to execute external commands. The list of built-in commands that your shell must support includes date, cd, mkdir, rmdir, pwd, kill, echo, hostname, and exit. This means that your program must implement these commands directly by using the system calls that implement them, or otherwise (echo doesn't require a system call). For all of the listed commands, do not use exec to implement them. Additionally, the exit and cd commands are special cases described below.
For all other commands (not built-in), your program must implement them using exec (using some version of exec). Because your program is to implement a simple shell, it should operate in a fashion similar to that as illustrated in the book, in Figure 1-19:
while (true) { print a command prompt get a line of user input if (they didn't type "exit" or "cd") { create a child process if (I am the parent) wait for the child else (I am the child) directly execute the sys call - OR - execute the command using a version of exec. } }
This specifically means the following: when the user types a command, your main program should create a child process (using fork) and then wait for that child process to terminate (using wait or waitpid). Meanwhile, the child process should parse the command and act accordingly - by executing a system call (the command is one of the required built-ins) or by using some version of exec to execute the command.
System Calls
This list may not represent all possible options, especially for the time information and the host name.
Time information: gettimeofday, localtime, strftime
Getting the host name: uname, gethostname
Creating (making) a directory: mkdir
Removing a directory: rmdir
Printing working directory: getcwd
Changing directory: chdir
Kill: kill
Creating a child process: fork
Waiting for a child process: waitpid, wait
As far as exec'd commands are concerned, I will try to only type commands having a maximum of three command-line parameters, but I will give some extra credit if it can handle more than that.
Child or Not
There are two situations in which your program should not create a child process as in the pseudocode and description above. The first is that your program should terminate operation when the user types exit. There is no need for the parent to create a child using fork (the "true" condition above should really be read as "the user hasn't typed exit"). In addition to the originating parent process, the exit command should cause any remaining child processes to terminate. If you have written your program correctly, there will be no child processes to terminate.
The second situation is when you use the cd command. The reason for not using fork here is the behavior of processes: once a child is created, it has a separate address space, environment, etc. Things a child does to itself do not affect the parent. Therefore, if a child is created using fork and then changes its directory and then terminates, then all is well with the child, but it will have had absolutely no effect on the parent process. The parent, which (for lack of a better term) is your main program, will not "observe" the effects of cd in that case.
Here is the shell:
#various include directives using namespace std; extern int errno; // you may want these. extern char **environ; // Possible function definitions omitted here //*************************************************************** // Print a prompt, get user input, then depending on what // they typed, either use a built-in with a syscall, or // call exec. //*************************************************************** int main(void) { char cmdline[100]; // An input line. char *token; // Used for parsing input lines // using strtok etc. char outbuf[100]; // Generic print buffer char *argv[256]; // The argument vector. User-typed. int argc; // The argument count. pid_t pid; // PID from fork. int stat; // Used by waidpid. // You may wish to have some sort of "initialize argv" loop here. while (!done) { // Until the user types exit... // Similarly, you may wish to have some sort of "clean up argv" // loop through here. Reset pointers to null. Call delete[] on any // that are not null. cout << "% "; // Print a prompt to the user cin.getline(cmdline,100); if (strcmp(cmdline,"exit") == 0) // They typed exit, so do that. done = true; else { // They didn't type exit. // Start using strtok to parse the string into component // parts. These get "placed into" (pointed-at by) argv[i]. // This is where we check to see what it is they typed. // If it's one of the built-ins, then fork a child to do // it (unless it was cd). // stuff the parent does, including fork. if (fork() == 0) { // I am the child // do various child stuff involving implementing the // built-ins, OR using exec to execute a command I do // not have built-in. } // end if (I am child) else { // Wait for child to terminate // (call wait or waitpid here) } } // End of kill processing } // End while (!done) exit(0);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
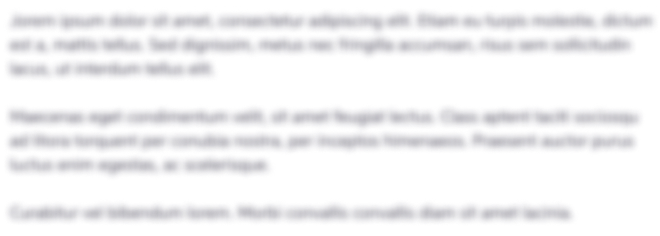
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started