Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this assignment you are to write a Python program to implement heapsort algorithm & apply it on a provided list. You are provided with
In this assignment you are to write a Python program to implement heapsort algorithm & apply it on a provided list.
You are provided with the template in which you must:
implement Phase II of a heapsort algorithm:
function that sifts down the value of the heap root to a correct position, and
function that finds the largest child of a given heap node.
Your program must:
take a file containing input list of values as a command line argument and print the original list,
create a heap form a given list of values and print created heap,
sort given list using heapsort algorithm and print sorted list.
Following is the output of the program run applied on a provided test file:
Together with your source code include a separate file
Namingformat.py with your information as the values of the
following variables:
myName 'firstname lastname'
myTechID
myTechEmail 'abc #only your email id omit @latech.edu
You must properly cite the sources if you use any help from peers or any codeideas from online resources. Failure to do
so will be treated as a plagiarism. Properly citing the sources should be done as a comment in your code. Some examples:
# found this code at:
# used idea from:
#
##### HAVE TO USE MY TEMPLATE #########
import sys
DEBUG False
class Heap:
def initself:
self.size
self.capacity
self.data None
def getDataself:
return self.data:self.size
def getSizeself:
return self.size
def isEmptyself:
return self.size
def siftUpself child:
parent child
while child and selfdatachild self.dataparent:
temp self.datachild
self.datachild self.dataparent
self.dataparent temp
if DEBUG:
printfselfdataparent swapped with selfdatachild
child parent
parent child
def buildFromself seq:
self.data Nonelenseq
self.size self.capacity lenseq
for x in rangeselfsize:
self.datax seqx
index self.data.indexseq
while index lenseq:
self.siftUpindex
index
def addToHeapself newVal:
if selfsize self.capacity:
self.capacity
temp None self.capacity
for i in rangeselfsize:
tempi self.datai
self.data temp
self.dataselfsize newVal
self.siftUpselfsize
self.size
return newVal
def largestChildself index, lastIndex:
Inputs:
index index of the current node
lastIndex index of the last node in the heap
Output:
index of the largest child if it exists, None otherwise
# find indexes of left and right child of the current index node
# return None if left child index is past last index
# otherwise return the index of the largest child
### WRITE YOUR CODE HERE###
def siftDownFromToself fromIndex, last:
Inputs:
fromIndex index of the node where to start sifting from
last index of the last node in the heap
Output:
the node sifted down as far as necessary to maintain heap conditions
# repeat until node is in the right position
# find index of a largest child
# if index of the largest child is not found then finish
# otherwise, if value of the largest child is larger than parent then swap
### WRITE YOUR CODE HERE###
def sortseq:
h Heap
hbuildFromseq
for i in rangelenseq:
hdata hdatahsize hdatahsize hdata
hsize
hsiftDownFromTo hsize
return hdata
def strself:
st ftHeap size: selfsize
st ftHeap capacity: selfcapacity
st ftElements of heap:
for x in rangeselfsize:
st fttValue: selfdatax at index: x
return st
def reprself:
return self.str
def main:
file opensysargvr
for line in file:
values intx for x in line.split
printfOriginal list: values
### WRITE YOUR CODE HERE###
printfHeapified list:
h
sortedlist Heap.sortvalues
print

Step by Step Solution
There are 3 Steps involved in it
Step: 1
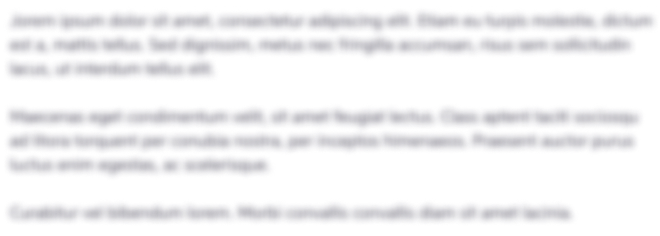
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started