Question
In this assignment, you will be writing your own vector class. Implement class vector consisting of an array of a generic type. This class will
In this assignment, you will be writing your own vector class. Implement class vector consisting of an array of a generic type. This class will require the following fields: mData: An array of items of a generic type. mCapacity: An integer representing the capacity of the vector, which is the number of items the array can contain. Hereafter known as the capacity. mSize: An integer representing the size of the vector, which is the number of items actually stored in the array. Hereafter known as the size. The capacity is always greater than or equal to the size. Your vector class must also support the following public member functions. (Please define everything in a vector.hpp file!): vector(): A default constructor which should call the initialization constructor with a value of 10. vector(const vector&): A copy constructor that takes a vector object and copies its contents into a dynamically allocated array with the same capacity. ~vector(): A destructor that deletes the data array. operator=(const vector&): vector& An overloaded assignment operator that deletes the current array and replaces it with a dynamically allocated array with the same capacity and contents as the provided vector object. size(): int Returns the size of the vector. push_back(const T): void Adds an item in the next open position if there is room. If there is no room, the application should allocate a new array that is twice the size of the current capacity and then copies all existing elements from the existing array to the new array. Then we delete the existing array and point our array field to the new array. This method should update the assignment count each time it performs and assigment. (This behavior of resizing the array should be its own private helper method called resizeArray(int) (where the passed int is the size of the new array). operator[]: T Returns the element stored at the specified index. Historically, if the index is out-of-bounds (either for being negative or greater than/equal the size of the vector), this function does nothing to warn the user that this is the bad idea. You can do that, or you can throw invalid\_argument("Index out of bounds.");. Im okay with either. See a better description below
Step by Step Solution
There are 3 Steps involved in it
Step: 1
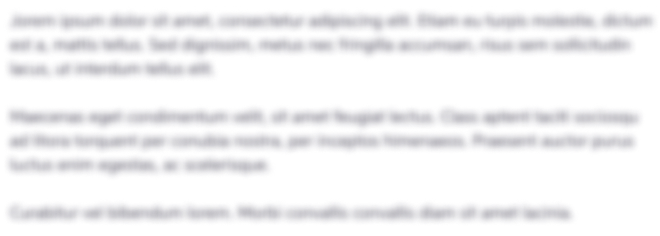
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started