Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this assignment, you will create two decorators. I suggest you follow 2 steps. Step 1 Refer to the trace decorator from Professor Allison's Chapter
In this assignment, you will create two decorators. I suggest you follow steps.
Step
Refer to the trace decorator from Professor Allison's Chapter slide set. It counts the number of times a decorated function is executed. Modify trace and rename it as track so that it
caches the result of all function calls, so that the decorated function is never called twice with the same arguments,
def trackf:
cache
def wrapperargskwargs:
counts the total number of function calls. Tracking the count of calls is accomplished by using a function attribute. To illustrate how this works, consider the recursive function that computes the nth Fibonacci number.
def fibn:
return n if n in else fibn fibn
This implementation makes many repeated function calls on the same argument. If we decorate it as follows:
@track
def fibn:
return n if n in else fibn fibn
then calling printfib 'count fib.count yields the following:
calls
Very wasteful! Your modified track decorator should print the following:
found in cache
found in cache
found in cache
found in cache
found in cache
found in cache
found in cache
found in cache
The eight trace statements are printed whenever a repeated call is attempted on the arguments shown. Note that both positional and keyword arguments function as the cache dictionary key. This is done with the following statement:
key strargs strkwargs
using the customary parameter names for decorators.
Step
Write a separate logging decorator that saves all function calls and their results in the file "log.txt This requires opening the file for appending:
logfile openlogtxta
You will need the wrapper function to open the file for each function call, write the information, and close the file.
When you decorate fib with only the log decorator, logtxt should contain the output below:
@log
def fibn:
return n if n in else fibn fibn
printfib # Prints
Now decorate fib with both track and log:
@track
@log
def fibn:
return n if n in else fibn fibn
printfib 'calls fib.count
The output should then be
found in cache
found in cache
found in cache
found in cache
found in cache
found in cache
found in cache
found in cache
calls
from the trace decorator, and logtxt should contain:
fib
fib
fib
fib
fib
fib
fib
fib
fib
fib
fib
Step by Step Solution
There are 3 Steps involved in it
Step: 1
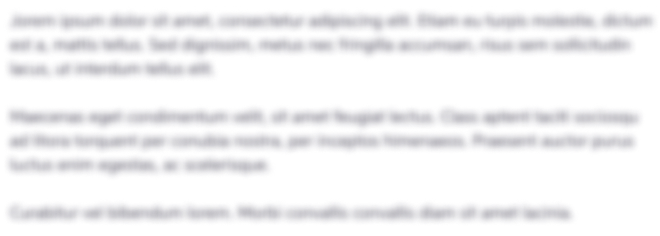
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started