Question
In this assignment, you will execute and manipulate a simple program written in MIPS assembly language. The code of this program that calculates the sum
In this assignment, you will execute and manipulate a simple program written in MIPS assembly language. The code of this program that calculates the sum of cubes of integers from one to a given non-negative integer can be found in the following .asm file:
.text main: # Prompt user to input non-negative number la $a0,prompt li $v0,4 syscall li $v0,5 #Read the number(n) syscall move $s2,$v0 # moves n to $s2. # Call function to get cube_sum #n move $a0,$s2 #alternatively, addi $a0, $s2, 0 jal cube_sum #call cube_sum (n) move $s3,$v0 #result is in $s3 # Output message and n la $a0,result #Print C_ li $v0,4 syscall move $a0,$s2 #Print n li $v0,1 syscall la $a0,result2 #Print = li $v0,4 syscall move $a0,$s3 #Print the answer li $v0,1 syscall la $a0,endl #Print ' ' li $v0,4 syscall # End program li $v0,10 syscall #int cube_sum(int n){int sum = 0; #for(int i = 0; i <= n;i++)sum+=cube(i); #return sum;} cube_sum: # Compute and return cube_sum number #Push $ra $s0, and $s1 to stack addi $sp,$sp,-16 sw $ra,0($sp) #storing return address in stack sw $s0,4($sp) #storing first saved register in stack sw $s1,8($sp) #storing second saved register in stack sw $s2,12($sp) #storing third saved register in stack move $s0, $a0 #n is now stored in $s0 move $s2, $zero #sum is now stored in $s2 and initialized to zero addi $s1, $zero, 1 #i is stored in $s1 and initialized to 1 for: slt $t0, $s0, $s1 bne $t0, $zero, exit #body of for loop move $a0, $s1 jal cube add $s2, $s2, $v0 #sum += cube(i) #end of for loop addi $s1, $s1, 1 j for exit: move $v0, $s2 #returning sum lw $ra,0($sp) #restoring return address from stack lw $s0,4($sp) #restoring first saved register from stack lw $s1,8($sp) #restoring second saved register from stack lw $s2,12($sp) #restoring third saved register from stack addi $sp,$sp,16 jr $ra #int cube(int n){ # return n*n*n; #} cube: #compute the cube of a number mul $t0, $a0, $a0 mul $t0, $t0, $a0 move $v0, $t0 jr $ra .data prompt: .asciiz "This program calculates the sum of cube sequence. Enter a non-negative number less than 100: " result: .asciiz "C_" result2: .asciiz " = " endl: .asciiz " "
In this program, function with label cube gets an input and returns. Also, function with label cube_sum gets an input and returns the following sum with the help of cube function: ni = 1 i 3
To execute this file, you can use this online service, or alternatively, install and use the QtSpim application.
Tasks
Here are the tasks that you need to do:
(20 points) Execute the given program, input number 9 through console, and find the program output printed on console. Take a snapshot of the value of all the 32 MIPS registers before and after executing the program. The values are visible on the main window of the online assembler or the QtSpim program.
(20 points) Manipulate the program assembly code so that it implements and prints out the return value of this function (instead of cube_sum):
int sum_odd_factorial(int n){ int sum = 0; for(int i = 1; i <= n;i+=2) sum += factorial(i); return sum; } |
where factorial is another function that you need to implement:
int factorial(int n) { int rv = 1; for(int i = 1; i <= n;i++) rv *= i; return rv; } |
3. (20 points) Execute the program that you wrote in step 2 for input 11. Take a snapshot of the value of all the 32 MIPS registers before and after executing the program.
4. (20 points) Manipulate the program assembly code so that it it implements and prints out the return value of this function:
int nested_loop_test(int n){ int rv = 1; for(int i = 1; i < n;i*=2) for(int j = i; j >=0; j--) if(j % 2 == 0) rv += (i + j); else rv += (2*i-3*j); return rv; } |
5. (20 points) Execute the program that you wrote in step 4 for input 20. Take a snapshot of the value of all the 32 MIPS registers before and after executing the program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
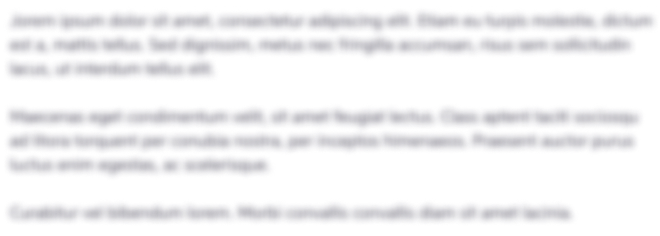
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started