Question
In this case I want you to attempt to write assembly code using ARM V Instruction Set based on the knowledge you have from writing
In this case I want you to attempt to write assembly code using ARM V Instruction Set based on the knowledge you have from writing assembly code using the MIPS 1 ISA.
There are a few differences in the way you write the code as the underlying hardware varies.
Assume assembler directives and labels are the same as they were in MIPS assembly code.
There are only 16 registers. (Look below for a list of the registers) Note: The first input argument and return value use the same register $a1.
When user programs need to communicate with the operating system they use (svc #CallCodeNumber) to cause an exception. Where as in MIPS we first input the call code into $vo then use the syscall instruction. Additionally if there is an input for the exception use the input argument register, $a1. Assume it will return any information we requested to the return register, $a1.
Finally assume we have the same operating system. THUS USE THE SAME SYSCALL CODES THAT YOU WERE USING FOR MIPS
Recall in ARM all instructions can be executed conditionally not just branch statements as in MIPS. Additionally, when branching in MIPS the instruction checks the condition(by calculating it) then branches if the condition is met(All using one instruction). In ARM we first check the condition then use the branch statement to branch based on the condition we want to check. NOTE: The branch statement only looks at a status register to see if one of the four conditions has been met by a previous instruction. HENCE IT DOES NOT ACTUALLY CALCULATE IF THE CONDITION HAS BEEN MET. IT MUST BE CALCULATED BEFORE THE BRANCH STATEMENT!
ARM ISA
Table 6.1. APCS registers
Register | APCS name | APCS role |
---|---|---|
r0 | a1 | argument 1/scratch register/result |
r1 | a2 | argument 2/scratch register/result |
r2 | a3 | argument 3/scratch register/result |
r3 | a4 | argument 4/scratch register/result |
r4 | v1 | register variable |
r5 | v2 | register variable |
r6 | v3 | register variable |
r7 | v4 | register variable |
r8 | v5 | register variable |
r9 | sb/v6 | static base/register variable |
r10 | sl/v7 | stack limit/stack chunk handle/register variable |
r11 | fp/v8 | frame pointer/register variable |
r12 | ip | scratch register/new -sb in inter-link-unit calls |
r13 | sp | lower end of the current stack frame |
r14 | lr | link register/scratch register |
r15 | pc | program counter |
Table 6.2. APCS floating-point registers
Name | Number | APCS Role |
---|---|---|
f0 | 0 | FP argument 1/FP result/FP scratch register |
f1 | 1 | FP argument 2/FP scratch register |
f2 | 2 | FP argument 3/FP scratch register |
f3 | 3 | FP argument 4/FP scratch register |
f4 | 4 | floating-point register variable |
f5 | 5 | floating-point register variable |
f6 | 6 | floating-point register variable |
f7 | 7 | floating-point register variable |
To summarize:
a1-a4, [f0-f3]
These are used to pass arguments to functions. a1 is also used to return integer results, and f0 to return FP results. These registers can be corrupted by a called function.
v1-v8, [f4-f7]
These are used as register variables. They must be preserved by called functions.
sb, sl, fp, ip, sp, lr, pc
These have a dedicated role in some APCS variants, though certain registers may be used for other purposes even when strictly conforming to the APCS. In some variants of the APCS some of these registers are available as additional variable registers. Refer to A more detailed look at APCS register usage for more information.
Convert the source code to ARM Assembly Code
int a = 5;
int const one = 1;
void main()
int b = 30;
int c = 75;
int d;
int array[1] = {7};
d= a + b + c + one;
array = new int [10]; //dynamically allocating size
array[4] = d;
array[8] = a;
array[2] = c;
a = a + 15;
if( a>0 )
{
a=1;
}
else
{
c = factorial( b );
}
for( int f=0; f<4; f=f+1; )
{
a = a+1;
d = b + c;
}
d = factorial (a);
int factorial(int n)
{
if(n<1)
return 1;
else
return ( n * factorial(n-1) );
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
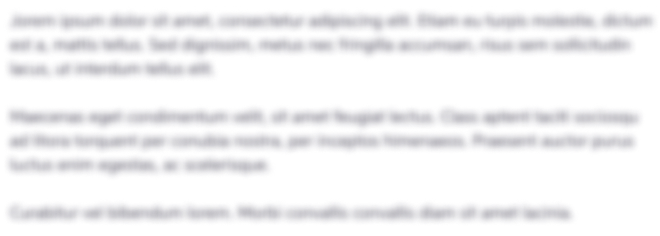
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started