Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this exercise we will work with graphs where the vertices are laid out in a grid like this: I have written the name of
In this exercise we will work with graphs where the vertices are laid out in a grid like this:
I have written the name of the vertex above itit is labeled by its row and column number, where rows count how many steps the vertex is down from the top, and column counts how many steps the vertex is to the right. We will only consider grid graphs where a vertex is possibly adjacent to a vertex immediately to its left, its right, above it or below it For example, the possible neighbours of vertex are and
We will represent a grid graph in a fun way, as a "maze" with walls and corridors like this:
#####
# # #
## #
# ##
#####
Here a # represents a wall and a is a corridor. This picture corresponds to the following graph:
Given two positions in the maze, they are adjacent in the graph if and only if
they are both valid positions in the maze
one can be reached from the other by a single step left, right, up or down
at both positions in the maze there is a space
The task for this week is given a maze and two positions, determine if they are adjacent.
Specifics
In more detail a maze will be given as a vector of strings, like this:
std::vector maze
#####
# #
# #
#####;
We promise that every string in the vector has the same length, and the only characters in the strings are and # It does not have to be the case that there is a border of walls around the maze as in this example.
To specify positions in the maze, we provide a simple struct called Point:
simple struct to hold a maze position
struct Point
int row ;
int col ;
;
We provide functions to add two Points together and tell if two Points are equal or not.
Your task is to write a function with the signature
bool isEdgeconst std::vector& const Point& const Point&;
Given a maze as above, and two points x and y this should return true if x and y are adjacent in the graph and false otherwise.
Examples
std::vector maze
## ##
# #
#
## ##;
EXPECTFALSEisEdgemaze; points must be valid positions in maze
EXPECTFALSEisEdgemaze; no self loops
EXPECTFALSEisEdgemaze; no diagonal moves
EXPECTFALSEisEdgemaze; no edge with a wall
EXPECTFALSEisEdgemaze; not reachable in a single step
EXPECTTRUEisEdgemaze; valid edge
EXPECTTRUEisEdgemaze; valid edge
EXPECTTRUEisEdgemaze; the graph is undirected
Complexity
Your solution should work in constant time.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
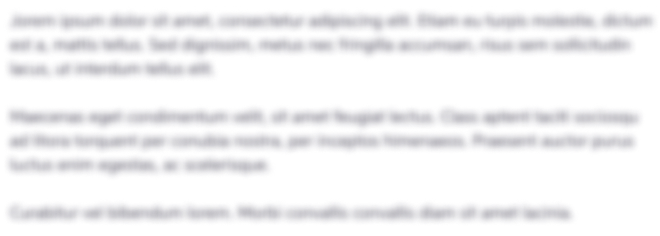
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started