Question
In this homework, you will implement a single linked list to store a list of computer science textbooks. Every book has a title, author, and
In this homework, you will implement a single linked list to store a list of computer science textbooks. Every book has a title, author, and an ISBN number. You will create 2 classes: Textbook and Library. Textbook class should have all above attributes and also a next pointer.
Library class should have a head node as an attribute to keep the list of the books. Also, following member functions should be implemented in this class:
Library Type Attribute Textbook* head Return Type Function constructor void addBook(string title, string author, string ISBN) void removeBook(string ISBN) void print() void print(char startingLetter) void print(string author) Textbook at(int index) int getSize() bool isEmpty()
addBook(): Adds a new book to the list. The new book is placed based on the ALPHABETICAL ORDER of the titles. You shouldnt add the textbook directly to the end or front of the list. The new book has to be inserted to the correct position. For example, if you have following three books:
A new book, Applied Cryptography will be placed in alphabetical order between the first and second book.
removeBook(): This functions will remove a book using the given ISBN number. If the given ISBN number is not in the list, it will give an error.
print(): Print all books in (alphabetical) order. Title, author, and the ISBN should be printed for each book.
print(char startingLetter): Prints all books whose title starts with the input character, startingLetter.
print(string author): Prints all books of an author.
at(int index): Returns the book at given index
getSize(): returns the number of books in the list
isEmpty(): returns true if list is empty, returns false otherwise.
The main program (main.cpp) is provided for you. So you will only implement the Textbook and library classes. I expect you to have 2 files: Library.h and Library.cpp. Textbook class definition will be in the Library.h file.
main.cpp includes all necessary functions to read the dataset file (dataset.txt). Also, several test cases are prepared for you to see whether your code is running or not. You do not need to change any code in the main.cpp file. Whenever you include your library class and main cpp to your project, they must run properly together.
**********main.cpp**********
Library myLib;
//Read the dataset file and loads myLib list
void loadDataset(string fileName){
ifstream inFile;
inFile.open(fileName.c_str());
string line;
while(getline(inFile,line)){
int ind1 = line.find("#");
int ind2 = line.find("#",ind1+1);
string title = line.substr(0,ind1);
string author = line.substr(ind1+1, ind2-ind1-1);
string ISBN = line.substr(ind2+1);
//Here we add the new book. Please make sure your function name matches
myLib.addBook(title,author,ISBN);
}
}
//Just for convenience...
void continueMessage(string message){
cout
cout
}
int main() {
string fileName = "dataset.txt";
loadDataset(fileName);
continueMessage("Dataset file is loaded to the program!");
//---------------------------------------------------------------------------
myLib.print();
continueMessage("All books in the library are listed!");
//---------------------------------------------------------------------------
string title = "Total Recall: How the E-Memory Revolution Will Change Everything";
string author = "C. Gordon Bell";
string ISBN = "592968760-9";
myLib.addBook(title,author,ISBN);
continueMessage("New book, with " + ISBN + " ISBN is added to the library.");
//---------------------------------------------------------------------------
myLib.print(title[0]); string s(1, title[0]);
continueMessage("The books, whose title starts with "+s+", are listed.");
//---------------------------------------------------------------------------
author = "W. Richard Stevens";
myLib.print(author);
continueMessage("The books, whose author is "+author+", are listed.");
//---------------------------------------------------------------------------
myLib.removeBook(ISBN);
continueMessage("The book, with " + ISBN + " ISBN is removed from the library.");
//---------------------------------------------------------------------------
Textbook tb = myLib.at(5);
cout
//---------------------------------------------------------------------------
int size = myLib.getSize();
cout
return 0;
}
**********dataset.txt**********
Introduction to Algorithms#Thomas H. Cormen#878134263-2 Structure and Interpretation of Computer Programs#Harold Abelson#336190405-6 The C Programming Language#Brian W. Kernighan#699022731-1 The Pragmatic Programmer: From Journeyman to Master#Andy Hunt#888099490-5 The Art of Computer Programming, Volumes 1-3 Boxed Set#Donald Ervin Knuth#625268126-1 Design Patterns: Elements of Reusable Object-Oriented Software#Erich Gamma#604854500-2 Introduction to the Theory of Computation#Michael Sipser#615228728-6 Code: The Hidden Language of Computer Hardware and Software#Charles Petzold#822491420-8 The Mythical Man-Month: Essays on Software Engineering#Frederick P. Brooks Jr.#987411743-5 Artificial Intelligence: A Modern Approach#Stuart Russell#704058716-5 Code Complete#Steve McConnell#465609758-6 The Protocols (TCP/IP Illustrated, Volume 1)#W. Richard Stevens#126456048-6 Algorithms#Robert Sedgewick#873746894-4 Advanced Programming in the UNIX Environment#W. Richard Stevens#598255768-4 A Discipline of Programming#Edsger W. Dijkstra#412861075-5 Introduction to Automata Theory, Languages, and Computation#John E. Hopcroft#952883775-1 Compilers: Principles, Techniques, and Tools#Alfred V. Aho#557104773-9 Joel on Software#Joel Spolsky#394368902-6 Learn You a Haskell for Great Good!: A Beginner's Guide#Miran Lipova a#260995077-2 The Society of Mind#Marvin Minsky#539224508-0
Textbook Type Attribute String String String Textbook* title author ISBN nextStep by Step Solution
There are 3 Steps involved in it
Step: 1
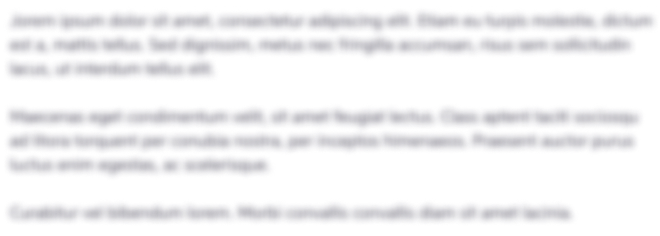
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started