Question
In this lab section, you are going to learn how to use loop statements. You are going to write an application that has two classes.
In this lab section, you are going to learn how to use loop statements. You are going to
write an application that has two classes. Each of these classes may have multiple
methods. One method may call another method within the same class.
This application will calculate the average score for a test taken by the students. It will
ask user to input the a number of entries. For each entry, the user will input a test score
(see Test Case 1 for detailed input values). Then the application will calculate the
average score and output the result. You need to use the following test data when you do
a screen capture.
Test Case 1
Input:
Welcome to ICS 141 Lab 2.
Number of entries: 3
Score1: 70
Score2: 90
Score3: 100
Output:
The average score for your inputs is:
// you figure out
The Design
This is a relatively simple application. Thus we are going to use two classes to
implement this application. The worker class is named GradeAverage and the
application class is named TestGradeAverage. The member information of the two
classes is:
1
TestGradeAverage
main()
GradeAverage
double: averageScore
GradeAverage() // constructor
static int readInt()
void calculateGradeAverage()
double getGradeAverage()
The relationship of the two classes is: TestGradeAverage uses GradeAverage. The
readInt() method is a static method. This method reads an integer from the standard input
(keyboard).
SCORE YOUR POINTS BY DOING THE FOLLOWING
According to the information given above, draw a class diagram in the space below (using
UML notation) (4 pts).
Programming work
In this section, you are asked to implement the above design and do some screen captures of the
outputs. You are given partial code and you need to finish the missing code according to the
requirements.
Specifically, you are given the following code:
class GradeAverage
Application class TestGradeAverage
And you need to supply the missing code for the following class methods:
calculateGradeAverade() in GradeAverage
getGradeAverage() in GradeAverage
The following is the partial code for this application:
2
import javax.swing.*;
public class GradeAverage {
private double averageScore;
public GradeAverage() {
averageScore = 0;
}
private static int readInt(String prompt) {
String numStr = JOptionPane.showInputDialog(prompt);
return Integer.parseInt(numStr);
}
public void calculateGradeAverage() {
int numberEntries = readInt(Number of entries: );
int score;
// supply the body of this method
// This method uses for-loop to receive
// n integers from the user by calling
// readInt() n times. Here, n equals to the
// numberEntries. This method also calculates
// and sets the instance variable averageScore
// to the appropriate value.
}
public double getGradeAverage() {
// supply the body of this method
// This method returns the instance variable
// averageScore.
}
}
Public class TestGradeAverage {
public static void main(String[] args) {
GradeAverage gradeAverage = new GradeAverage();
System.out.println(Welcome to ICS 141 Lab 2.);
gradeAverage.calculateGradeAverage();
System.out.println(The average score for your inputs is: + gradeAverage.getGradeAverage());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
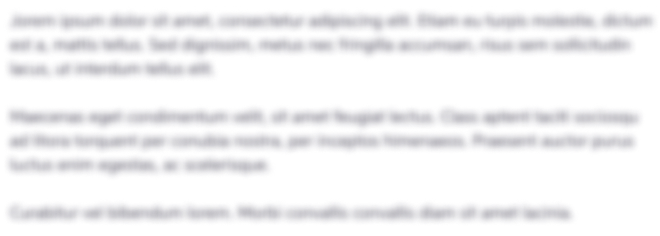
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started