Question
In this problem, you will write part of a program to grade students. Suppose we have a String[] names array of student names, and a
In this problem, you will write part of a program to grade students. Suppose we have a String[] names array of student names, and a double[] grades array of student grades. We know that the grades array is the same length as the names array, and the value at grades[index] is the grade for the student named names[index].
Also suppose that a passing grade is 65.0 -- any grade less than this indicates fail, while greater than or equal to 65.0 indicates passing.
We want to write a method printPassOrFail which prints out all of the students names, and next to each, whether that student passed or failed.
For instance, if we declare:
String[] names = { "Peter", "Christine", "Glenn" }; double[] grades = { 90.0, 90.0, 50.0 };
then printPassOrFail(names, grades) prints out:
Peter: pass
Christine: pass
Glenn: fail
If we declare
String[] names = { "Bill", "Michael", "Rudi" }; double[] grades = { 65.0, 64.9, 0.0 };
then printPassOrFail(names, grades) prints out:
Bill: pass
Michael: fail
Rudi: fail
Your method should not return any values, and you must use System.out.print and/or System.out.println in your solution. Write your solution on the following page.
// Prints out student names along with "pass" or "fail" (depending on // whether the corresponding grade is less than or greater than 65). // Precondition: names.length == grades.length
public static void printPassOrFail(String[] names, double[] grades) {
Step by Step Solution
There are 3 Steps involved in it
Step: 1
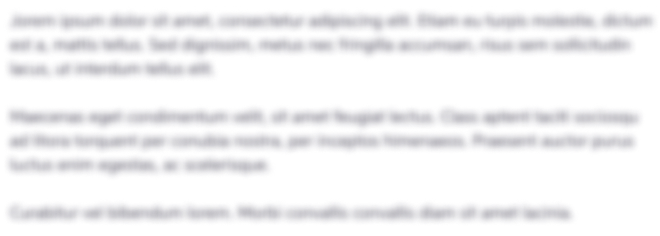
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started