Question
In this project, you will implement the matrix multiplication-based solution we saw in class to determine the number of walks of length l between any
In this project, you will implement the matrix multiplication-based solution we saw in class to determine the number of walks of length l between any two vertices. The walk length is 4 for all students. The graph on which your code has to be tested is assigned below. You are given a startup code (in C++/Java) that reads in the list of edges and sets up the adjacency matrix as a two-dimensional array. Your task would be to extend the code such that the procedure to compute the number of walks of length l is implemented. For ease of implementation, vertex ID starts with 0. Below, I show the list of edges (stored as a text file) and a screenshot of the expected output for a sample graph.
C++ code
#include
#include
#include
#include
#include
#include
#include
#include
#include
using namespace std;
void printMatrix(int** matrix, int numNodes){
for (int uNodeID = 0; uNodeID
for (int vNodeID = 0; vNodeID
cout
cout
}
}
int main(){
string graphEdgesFilename;
cout
cin >> graphEdgesFilename;
int numNodes;
cout
cin >> numNodes;
int walkLength;
cout
cin >> walkLength;
int **adjacencyMatrix; // pointer to a pointer
adjacencyMatrix = new int* [numNodes]; // creating an array of pointers
for (int rowID = 0; rowID
adjacencyMatrix[rowID] = new int[numNodes]; // each pointer points to an array of elements in a row
}
for (int uNodeID = 0; uNodeID
for (int vNodeID = 0; vNodeID
adjacencyMatrix[uNodeID][vNodeID] = 0;
}
}
ifstream graphEdgeFileReader(graphEdgesFilename);
if (!graphEdgeFileReader){
cout
return 0;
}
int numCharsPerLine = 25;
char *line = new char[numCharsPerLine];
// '25' is the maximum number of characters per line
graphEdgeFileReader.getline(line, numCharsPerLine, ' ');
// ' ' is the delimiting character to stop reading the line
while (graphEdgeFileReader){
char* cptr = strtok(line, " \t");
string uNodeToken(cptr);
int uNodeID = stoi(uNodeToken);
cptr = strtok(NULL, " \t");
string vNodeToken(cptr);
int vNodeID = stoi(vNodeToken);
adjacencyMatrix[uNodeID][vNodeID] = 1;
adjacencyMatrix[vNodeID][uNodeID] = 1;
graphEdgeFileReader.getline(line, numCharsPerLine, ' ');
}
cout
printMatrix(adjacencyMatrix, numNodes);
return 0;
}
2 2 3) 2 Sample Graph edgelnfo.txt Enter the file name for the edges of the graph: edgeInfo.txt Enter number of nodes 6 Enter the walk length: 4 Initial Adjacency Matrix 0 1 0 0 0 1 1 0 1 1 1 1 0 1 0 1 0 0 0 1 1 1 0 0 1 0 1 0 1 1 1 0 1 0 Final Walk Length Matrix (LengthStep by Step Solution
There are 3 Steps involved in it
Step: 1
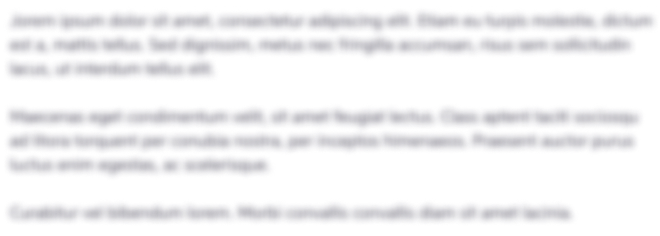
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started