Question
In this week's lesson, the algorithms quicksort and bubblesort are described. In Sorting Algorithms (Links to an external site.)Links to an external site. you can
In this week's lesson, the algorithms quicksort and bubblesort are described. In Sorting Algorithms (Links to an external site.)Links to an external site. you can find the class ArrayList, where these sorting algorithms are implemented. Write a program that times both of them for various list lengths, filling the array lists with random numbers. Use at least 10 different list lengths, and be sure to include both small values and large values for the list lengths (it might be convenient to add a parameterized constructor to the class ArrayList so the size of the list can be set at the moment an ArrayList object is declared).
Create a table to record the times as follows.
List Length | Bubblesort Time (seconds) | Quicksort Time (seconds) |
Regarding the efficiency of both sorting methods, what are your conclusions? In addition to the source code and a screenshot of the execution window, please submit a separate document with the table and your conclusions about the experiment. Note: To time a section of your source code, you can do this.
#include using namespace std; int main() { start = chrono::steady_clock::now(); //add code to time here end = chrono::steady_clock::now(); chrono::duration timeElapsed = chrono::duration_cast>(end-start); cout << "Code duration: " << timeElapsed.count() << " seconds" << endl; }
Main.cpp
/******************************************** * Week 4 lesson: * * ArrayList class with sorting algorithms * *********************************************/
#include
using namespace std;
/* * Program to test the ArrayList class. */ int main() { srand((unsigned)time(0));
//creating a list of integers ArrayList numbersCopy1, numbersCopy2;
//filling the list with random integers for (int i = 0; i<10; i++) { int number = rand()%100; numbersCopy1.add(number); numbersCopy2.add(number); }
//printing the list cout << "Original list of numbers:" << endl <<"\t"; numbersCopy1.display();
//testing bubblesort cout << endl << "Bubble-sorted list of numbers:" << endl <<"\t"; numbersCopy1.bubbleSort(); numbersCopy1.display();
//testing quicksort cout << endl << "Quick-sorted list of numbers:" << endl <<"\t"; numbersCopy2.quicksort(); numbersCopy2.display();
return 0; }
ArrayList.h
/******************************************** * Week 4 lesson: * * ArrayList class with sorting algorithms * *********************************************/
/* * Class implementing an array based list. Bubblesort and quicksort algorithms * are implemented also. */ class ArrayList { public: ArrayList (); ~ArrayList(); bool isEmpty(); void display(); void add(int); void removeAt(int); void bubbleSort(); void quicksort(); private: void quicksort(int, int); int findPivotLocation(int, int); int SIZE; //size of the array that stores the list items int *list; //array to store the list items int length; //amount of elements in the list };
ArrayList.cpp
/******************************************** * Week 4 lesson: * * ArrayList class with sorting algorithms * *********************************************/
#include
using namespace std;
/* * Default constructor. Sets length to 0, initializing the list as an empty * list. Default size of array is 20. */ ArrayList::ArrayList() { SIZE = 20; list = new int[SIZE]; length = 0; }
/* * Destructor. Deallocates the dynamic array list. */ ArrayList::~ArrayList() { delete [] list; list = NULL; }
/* * Determines whether the list is empty. * * Returns true if the list is empty, false otherwise. */ bool ArrayList::isEmpty() { return length == 0; }
/* * Prints the list elements. */ void ArrayList::display() { for (int i=0; i < length; i++) cout << list[i] << " "; cout << endl; }
/* * Adds the element x to the end of the list. List length is increased by 1. * * x: element to be added to the list */ void ArrayList::add(int x) { if (length == SIZE) { cout << "Insertion Error: list is full" << endl; } else { list[length] = x; length++; } }
/* * Removes the element at the given location from the list. List length is * decreased by 1. * * pos: location of the item to be removed */ void ArrayList::removeAt(int pos) { if (pos < 0 || pos >= length) { cout << "Removal Error: invalid position" << endl; } else { for ( int i = pos; i < length - 1; i++ ) list[i] = list[i+1]; length--; } }
/* * Bubble-sorts this ArrayList */ void ArrayList::bubbleSort() { for (int i = 0; i < length - 1; i++) for (int j = 0; j < length - i - 1; j++) if (list[j] > list[j + 1]) { //swap list[j] and list[j+1] int temp = list[j]; list[j] = list[j + 1]; list[j + 1] = temp; } }
/* * Quick-sorts this ArrayList. */ void ArrayList::quicksort() { quicksort(0, length - 1); }
/* * Recursive quicksort algorithm. * * begin: initial index of sublist to be quick-sorted. * end: last index of sublist to be quick-sorted. */ void ArrayList::quicksort(int begin, int end) { int temp; int pivot = findPivotLocation(begin, end);
// swap list[pivot] and list[end] temp = list[pivot]; list[pivot] = list[end]; list[end] = temp;
pivot = end;
int i = begin, j = end - 1;
bool iterationCompleted = false; while (!iterationCompleted) { while (list[i] < list[pivot]) i++; while ((j >= 0) && (list[pivot] < list[j])) j--;
if (i < j) { //swap list[i] and list[j] temp = list[i]; list[i] = list[j]; list[j] = temp;
i++; j--; } else iterationCompleted = true; }
//swap list[i] and list[pivot] temp = list[i]; list[i] = list[pivot]; list[pivot] = temp;
if (begin < i - 1) quicksort(begin, i - 1); if (i + 1 < end) quicksort(i + 1, end); }
/* * Computes the pivot location. */ int ArrayList::findPivotLocation(int b, int e) { return (b + e) / 2; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
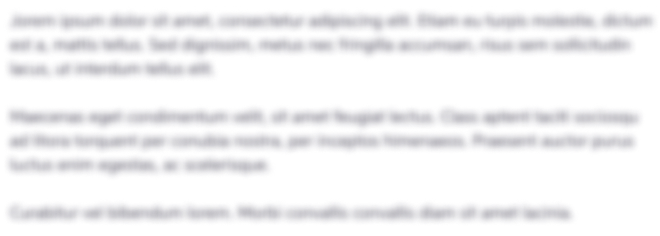
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started