Question
#include #include #include #include using namespace std; void f(int x, int &y); int main(){ char s1[] = zoey; char s2[] = zoey; string s3 =
#include
#include
#include
#include
using namespace std;
void f(int x, int &y);
int main(){
char s1[] = "zoey";
char s2[] = "zoey";
string s3 = s1;
string s4 = s2;
cout << "1. sizeof(s1) = " << sizeof(s1) << endl;
if(s1 == s2) cout << "2. s1 == s2 is true" << endl;
else cout << "2. s1 == s2 is false" << endl;
if(s3 == s4) cout << "3. s3 == s4 is true" << endl;
else cout << "3. s3 == s4 is false" << endl;
array
vector
for(size_t i = 0; i < 4; i++){
v.push_back((unsigned long) &d[i+1] - (unsigned long) &d[i]);
}
cout << "4. The values stored in v are ";
for(int value : v){
cout << value << " ";
}
cout << endl;
char * p1 = &s4[0];
*p1 = 'j';
*(&s4[3]) = 's';
cout << "5. s4 = " << s4 << endl;
s4 = s1;
string s5("zoey\0 is awesome");
cout << "6. s5 = " << s5 << endl;
int q[5];
int * p2 = q;
for(int i = 1; i < 6; i++) *(p2 + i - 1) = i * 5;
f(*(q + 1), *(q + 2));
cout << "7. What is the value of (q[1] + q[2])?" << endl;
cout << "8. What is the value of q?" << endl;
cout << "9. p2 = " << (unsigned long) p2 << ", q = " << (unsigned long) q << endl;
cout << "10. p2 = " << p2 << ", q = " << q << endl;
return 0;
}
void f(int x, int &y){
x = -1;
y = -2;
}
An output for the C++ (version 14) program executed above is:
1. sizeof(s1) = 5
2. s1 == s2 is false
3. s3 == s4 is true
4. The values stored in v are 8 8 8 8
5. s4 = joes
6. s5 = zoey
7. What is the value of (q[1] + q[2])?
8. What is the value of q?
9. p2 = 140726181901504, q = 140726181901504
10. p2 = 0x7ffd5e1534c0, q = 0x7ffd5e1534c0
Answer the following 10 questions based on the C++ program and its
output above.
1. In the output for 1 above, why is sizeof(s1) = 5 even though s1 contains only four letters? What is character is being
stored in the fifth byte of s1?
2. In the output for 2 above, why does s1 == s2 result in false when both s1 and s2 contain the same character sequence?
What values are being compared in s1 == s2 to yield a result of false?
3. In the output for 3 above, why does s3 == s4 result in true? What values are being compared in s3 == s4? What
types of strings are s3 and s4, and how are they different than the types of string used for s1 and s2?
4. In the output for 4 above, explain why the values stored in v are all 8? Explain your answers in terms of how the
memory addresses are being stored for d. Assume the starting address of d is 500, then what are the memory addresses
for d[0], d[1], d[2], d[3], and d[4]?
5. Why is joes in the output for 5 above? Explain how the first character in s4 was changed to j. Explain how the last
character in s4 was changed to s.
6. Why did the output for 6 above print s5 = zoey instead of s5 = zoey is awesome?
7. As asked in the output for 7 above, what is the value of (q[1] + q[2])? Is it correct syntax to use *(q[1] + q[2]) to
compute the value of (q[1] + q[1])? Explain why this syntax is correct or why it isnt correct.
8. As asked in the output for 8 above, what is the value of q? Explain what this value represents in terms of what is going
on in the programs memory at runtime.
9. Consider the output for 9 and 10 above. Why do these memory addresses typically change each time this program is
run?
10. Consider the output for 9 and 10 above. The memory addresses that are being display to standard output for 9 above
are using which number system: binary, octal, base-10, or hexadecimal? The memory addresses that are being display
to standard output for 10 above are using which number system: binary, octal, base-10, or hexadecimal?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
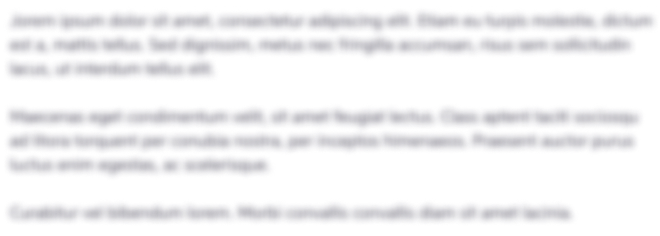
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started