Question
#include #include #include llist.h /* Private node ADT. */ typedef struct _node node; struct _node { node * next; void * e; }; static node
#include
#include "llist.h"
/* Private node ADT. */
typedef struct _node node;
struct _node { node * next; void * e; };
static node * newNode(void * e) { node * n = malloc(sizeof(node)); if (!n) return NULL; n->next = NULL; n->e = e; return n; }
static void deleteNode(node * n) { free(n); }
/* Linked list library. */
struct _llist { node * head; };
llist * newLList(void) { llist * ll = malloc(sizeof(llist)); if (!ll) return NULL; ll->head = NULL; return ll; }
void deleteLList(llist * ll) { if (!ll) return; node * n = ll->head; while (n) { node * next = n->next; deleteNode(n); n = next; } free(ll); }
int isEmptyLList(llist const * ll) { if (!ll) return 0; return(ll->head == NULL); }
int putLList(llist * ll, void * e) { node * n; if (!ll) return -1; n = newNode(e); if (!n) return -1; n->next = ll->head; ll->head = n; return 0; }
int getLList(llist * ll, void ** e) { node * n; if (!ll || !e) return -1; if (ll->head == NULL) { /* nothing to get */ *e = NULL; return -2; } n = ll->head; *e = n->e; /* write element */ ll->head = n->next; deleteNode(n); return 0; }
int peekLList(llist const * ll, void ** e) { if (!ll || !e) return -1; if (ll->head == NULL) { /* Nothing to get. */ *e = NULL; return -2; } *e = ll->head->e; /* write element */ return 0; }
int printLList(llist const * ll, printFn f) { node * n; int cnt; if (!ll || !f) return -1;
cnt = 0; for (n = ll->head; n != NULL; n = n->next) { /* Print the index of the element. */ cnt++; printf(" %d:", cnt); /* Call user-provided f to print the element. */ f(n->e);}
printf(" "); return 0;}
int zip(llist * ll1, llist * ll2, llist * ll3) { /* Your code goes here. */ return 0; }
Exercise 8.18. Using the llist type declared above, implement a function to "zip" together two linked lists: /Zips together the lists 1 and 112. For ezample, if 1L1 is [o, 1, 21 and l112 is [3, 4. 5, 6, 77, then after running, 113 will be o, 3. 1. 4. 2, 5, 6, 77. and both *l1 and l12 will be empty void zip (llist 111, llist 112, llist 113); This is Exercise 8.18 from our book, with the following difference: Instead of having a return type of void, zip should return an int: 0 if successful and -1 otherwise. It is an error to call zip with a nonempty list as third argument. It is also an error to call zip with one of the input lists the same as the output list. (For instance, zip(I1,112,11). Can you see the problem such a call would cause?). Your implementation should check for such errors. Of course, passing NULL pointers for some of the lists in not allowed either. On the other hand, it's OK to call zipwith empty I1 or I12 (or both). The test program actually checks that the correct result is produced in that case. Exercise 8.18. Using the llist type declared above, implement a function to "zip" together two linked lists: /Zips together the lists 1 and 112. For ezample, if 1L1 is [o, 1, 21 and l112 is [3, 4. 5, 6, 77, then after running, 113 will be o, 3. 1. 4. 2, 5, 6, 77. and both *l1 and l12 will be empty void zip (llist 111, llist 112, llist 113); This is Exercise 8.18 from our book, with the following difference: Instead of having a return type of void, zip should return an int: 0 if successful and -1 otherwise. It is an error to call zip with a nonempty list as third argument. It is also an error to call zip with one of the input lists the same as the output list. (For instance, zip(I1,112,11). Can you see the problem such a call would cause?). Your implementation should check for such errors. Of course, passing NULL pointers for some of the lists in not allowed either. On the other hand, it's OK to call zipwith empty I1 or I12 (or both). The test program actually checks that the correct result is produced in that caseStep by Step Solution
There are 3 Steps involved in it
Step: 1
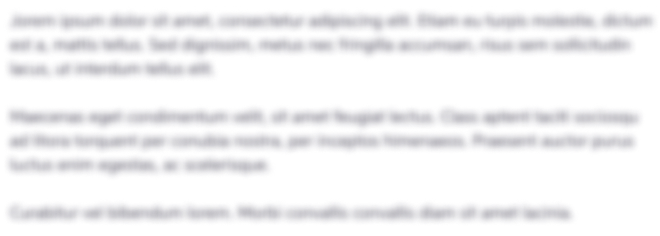
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started