Question
#include //STEP 1 - Write a statement that creates a symbolic constant for the value of 5 here void bubbleSort(int * const array, const size_t
#include
void bubbleSort(int * const array, const size_t size);
int main(void) {
// STEP 2 - create an integer array using the symbolic constant from step 1 // You DO NOT need to use an initializer list
// STEP 3 - create a pointer to the above array
// STEP 4 - Using POINTER OFFSET NOTATION within a loop, fill the array with values that are 2 * the index of the element // So, the value at index 0 will be 0, the value at index 1 will be 2, the value at index 2 will be 4, etc. for (size_t i=0; i < ; i++) //loop body goes here
puts("Data items in original order");
// STEP 5 - Update this loop as necessary to print the data in your array for (size_t i = 0; i < ; ++i) { printf("%4d", ); }
// STEP 6 - Update this call to the bubblesort function, using the information needed for your array bubbleSort( ); // sort the array
puts(" Data items after sorting in descending order"); // STEP 7 - Update this loop as necessary to print the data in your array. Hint - just copy what you have // for step 5. for (size_t i = 0; i < ; ++i) { printf("%4d", ); }
puts(""); // STEP 9 - AFTER you get this project working for an array of size 5, change the code where necessary to use an array of size 10 // Submit both versions!! }
// sort an array of integers using bubble sort algorithm void bubbleSort(int * const array, const size_t size) { void swap(int *element1Ptr, int *element2Ptr); // prototype // loop to control passes for (unsigned int pass = 0; pass < size - 1; ++pass) {
// loop to control comparisons during each pass for (size_t j = 0; j < size - 1; ++j) {
// STEP 8 - Update this if statement so the array will be sorted in DESCENDING order
// swap adjacent elements if theyre out of order if (array[j] > array[j + 1]) { swap(&array[j], &array[j + 1]); } } } }
// swap values at memory locations to which element1Ptr and // element2Ptr point void swap(int *element1Ptr, int *element2Ptr) { int hold = *element1Ptr; *element1Ptr = *element2Ptr; *element2Ptr = hold; }
Read the comments(Steps)....im stumped thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
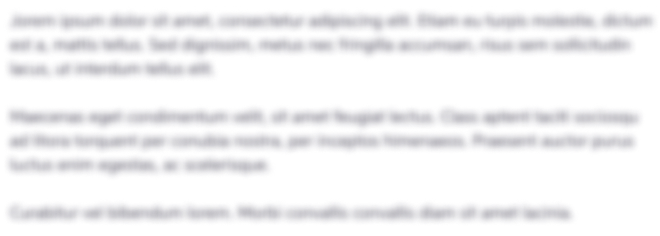
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started