Question
Description: In this final evaluation, you will be responsible for analyzing and improving your assignment one using your understanding of object orientation. The goal of
Description: In this final evaluation, you will be responsible for analyzing and improving your assignment one using your understanding of object orientation. The goal of this evaluation is to determine how much you have learned over the semester. 1) Perform a code critique on your first assignment: identify ways that the program could be improved and enhanced through object oriented techniques. Comment the code with your suggestions. Once you're finished, change the file extension to .txt to avoid compiler issues.
2) Implement the changes that you have suggested in part 1. Be sure to comment your code.
My code:
Character class:
import java.util.Random;
//Characters class public class Characters { //Immutable attributes private final String FIRST; private final String LAST; private final char SEX; private final String RACE; private final String CLASS; //Mutable atrributes private int armorClass; private int hp; private int strength; private int dexterity; private int constitution; private int intelligence; private int wisdom; private int charisma; private Random rand; private String[] actionMessages; private String[] encouragementMessages; private String[] badHappeningMessages; private String[] sadMessages; //Parameterized constructor. public Characters (String FIRST, String LAST, char SEX, String RACE, String CLASS) { this.FIRST = FIRST; this.LAST = LAST; this.SEX = SEX; this.RACE = RACE; this.CLASS = CLASS; rand = new Random(); armorClass = generator(); hp = generator(); strength = generator(); dexterity = generator(); constitution = generator(); intelligence = generator(); wisdom = generator(); charisma = generator(); actionMessages = new String[] { this.getFirst() + " dodges a flurry of daggers that rain down all around " + (this.getSex() == 'F' ? "her" : " him!") + "... ", this.getFirst() + " walks through and trips over trap wire, triggering boulders from above to fall all around " + (this.getSex() == 'F' ? "her" : " him!") + "... ", this.getFirst() + " rides through the swamp, startling a tribe of acquadocs who beging firing darts at " + (this.getSex() == 'F' ? "her" : " him!") + "... ", this.getFirst() + "'s horse becomes spooked and throws " + (this.getSex() == 'F' ? "her" : " him!") + " off into quick sand... " }; encouragementMessages = new String[] { "\"Never fret, indeed,\" sighs " + this.getFirst() + "... ", "\"Awh, that was a close one\" " + this.getFirst() + " says with relief... ", "\"Jack be nibble, Jack be quick, " + this.getFirst() + " jump over the candle stick... \"" + " chants " + this.getFirst() + " after escaping the danger... ", "\"It's a good thing I'm quick on my feet, otherwise I'd be a goner\" " + this.getFirst() + " let's out with relief... " }; badHappeningMessages = new String[] { this.getFirst() + " is suddenly struck by a flurry of arrows!... ", this.getFirst() + " did not make it through the entrapment untouched... ", this.getFirst() + " takes on heavy damage... ", this.getFirst() + " was unlucky, as " + (this.getSex() == 'F' ? "she" : " he") + " took damage from the swamp... " }; sadMessages = new String[] { " \"Boy was I a fool in school for cutting gym....\"" + this.getFirst() + " sighs with " + (this.getSex() == 'F' ? "her" : " his") + " last breath... ", "\"I should have ran faster to avoid danger....\"" + " says " + this.getFirst() + " to " + (this.getSex() == 'F' ? "her" : " his") + " companions... ", "\"I should have be bobbing when I was weaving...\"" + " says " + this.getFirst() + " as " + (this.getSex() == 'F' ? "she" : " he") + " lays there helpless... " , "\"It's sad " + this.getFirst() + " left us this way\"... the group agreed... " }; } //random stat generator private int generator() { return rand.nextInt(90) + 10; } //to show Characters attributes public String show_stats() { return "[ " + FIRST + " " + LAST + ": armorClass=" + armorClass + ", hp=" + hp + ", strength=" + strength + ", dexterity=" + dexterity + ", constitution=" + constitution + ", intelligence=" + intelligence + ", wisdom=" + wisdom + ", charisma=" + charisma + " ]"; } //Accessors for immutable variables public String getFirst() { return FIRST; }
public void setFirst(String first) { } public String getLast() { return LAST; }
public void setLast(String last) { } public char getSex() { return SEX; }
public void setSex(char sex) { } public String getRace() { return RACE; }
public void setRace(String race) { }
public String getClazz() { return CLASS; }
public void setClass(String Class) { } //Accessors for mutable variables public int getArmorClass() { return armorClass; } public int getHp() { return hp; } public int getStrength() { return strength; } public int getDexterity() { return dexterity; } public int getConstitution() { return constitution; } public int getIntelligence() { return intelligence; } public int getWisdom() { return wisdom; } public int getCharisma() { return charisma; } public Random getRand() { return rand; } public String[] getActionMessages() { return actionMessages; } public String[] getEncouragementMessages() { return encouragementMessages; } public String[] getBadHappeningsMessages() { return badHappeningMessages; } public String[] getSadMessages() { return sadMessages; } //to increment or decrement attribute public int armorClass(int value) { armorClass = checkStat(value, armorClass); return armorClass; } public int hp(int value) { hp = checkStat(value, hp); return hp; } public int strength(int value) { strength = checkStat(value, strength); return strength; } public int dexterity(int value) { dexterity = checkStat(value, dexterity); return dexterity; } public int constitution(int value) { constitution = checkStat(value, constitution); return constitution; } public int intelligence(int value) { intelligence = checkStat(value, intelligence); return intelligence; } public int wisdom(int value) { wisdom = checkStat(value, wisdom); return wisdom; } public int charisma(int value) { charisma = checkStat(value, charisma); return charisma; } //validation to increment and decrement operation private int checkStat(int value, int attribute) { if(attribute + value < 0) { System.out.println("this stat cannot go any lower "); }else { return attribute + value; } return 0; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
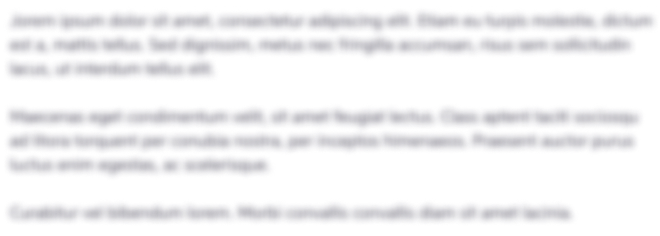
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started