Question
Incorporate all feedback received on Program #1 into the program. Create a new class called Peg. Peg objects should be able to be named and
Incorporate all feedback received on Program #1 into the program.
Create a new class called Peg. Peg objects should be able to be named and utilize a stack-like structure (like vector) to store disks. All class attributes should be private.
Your Peg class should have a constructor (that initializes a peg with a name and a certain number of disks) and a destructor. It should have an accessor and a modifier for each attribute. It should have methods to add a disk to a peg, return the number of disks on a peg, output the list of disks on a peg to the display, return the value of the top disk on a peg, and remove a disk from a peg. Use asserts as appropriate to enforce proper behavior.
All previous references to the PegType struct should be removed from HanoiPegClass. Use the Peg Class accessors and mutators instead. The Hanoi program (main(), hanoi(), and moveDisk()) should not utilize strings or vectors.
The overall Hanoi program should work the same as it did for Program #1: output the starting and ending conditions of all 3 pegs, output each move, and output the total number of moves required to move a stack of 7 disks.
PLEASE HELP ME FIGURE OUT WHY I HAVE ERRORS! THANK YOU !
THIS IS MY PEG.H FILE
// Joyce Martinez
#pragma once #include
class Peg { private: int numDisk; void loadDisk(int numDisk); vector
public: Peg(string newName, int numDisk);
//Accessors string getName() const; int getSize() const;
//Mutators void setName(string newName);
//Other public methods void printPeg() const; void addDisk(Peg &endPeg, Peg &startPeg); void removeDisk(); void lastDisk();
~Peg(); };
THIS IS MY PEG.CPP FILE
#include "stdafx.h" #include "Peg.h" #include
using namespace std;
void Peg::loadDisk(int numDisk) { int i;
assert(numDisk > 0); for (i = numDisks; i > 0; i--) { Peg.push_back(i); }
return i; }
// Constructors Peg::Peg(string newName, int numDisk) { setName(newName); loadDisk(numDisk); }
//Accessors string Peg::getName() const { return pegName; }
int Peg::getSize() const { return Peg.size(); }
//Mutators void Peg::setName(string newName) { pegName = newName; }
// Other public methods
void Peg::printPeg() const { for (unsigned int i = 0; i < Peg.getSize(); i++) { cout << Peg[i] << endl; } }
void Peg::addDisk(Peg &endPeg, Peg &startPeg) { (endPeg.getSize).push_back(startPeg.getSize).back(); }
void Peg::removeDisk() { Peg.pop_back(); }
void Peg::lastDisk() { Peg.back(); }
//Deconstructor
Peg::~Peg() { cout << "I am a deconstructor" << endl; }
// HanoiClass.cpp : Defines the entry point for the console application. // Joyce Martinez
#include "stdafx.h"
#include "Peg.h" #include
using namespace std;
int main() { int numDisk = 7;
Peg peg1("Peg 1", numDisk); Peg peg2("Peg 2", 0); Peg peg3 ("Peg 3", 0);
cout << "-------------------------------" << endl; cout << " Towers of Hanoi " << endl; cout << "-------------------------------" << endl << endl;
loadDisk(peg1.diskStack, numDisk); // Load Peg 1 with 7 disks
cout << " peg1 has " << peg1.diskStack.size() << " discs"; cout << " peg2 has 0" << " discs"; cout << " peg3 has 0" << " discs" << endl;
cout << "Starting Condition: " << endl << endl; cout << "Peg 1: " << endl; printPeg(peg1.diskStack);
cout << "Peg 2: " << endl; printPeg(peg2);
cout << "Peg 3: " << endl; printPeg(peg3);
int total = hanoi(peg1, peg2, peg3, numDisk);
cout << " End Condition: " << endl;
cout << "peg1 has " << peg1.getSize() << " discs"; cout << " peg2 has " << peg2.getSize() << " discs"; cout << " peg3 has " << peg3.getSize() << " discs" << endl;
cout << "Peg 1: " << endl; printPeg(peg1);
cout << "Peg 2: " << endl; printPeg(peg2);
cout << "Peg 3: " << endl; printPeg(peg3;
cout << " A stack of " << numDisk << " can be transferred in " << total << " moves!" << endl << endl;
system("pause"); return 0; }
int hanoi(Peg &startPeg, Peg &swapPeg, Peg &endPeg, int numDisk) { int totalMoves(0); if (numDisk > 0) // the peg has to have disks on it { totalMoves = hanoi(startPeg, endPeg, swapPeg, numDisk - 1); moveDisk(startPeg, endPeg); totalMoves++; totalMoves += hanoi(swapPeg, startPeg, endPeg, numDisk - 1); // count total moves } return totalMoves; }
void moveDisk(Peg &startPeg, Peg &endPeg) { assert(startPeg.getSize() > 0); // makes sure that there is something on the peg
if (endPeg.getSize() > 0) { assert(startPeg.lastDisk() < endPeg.lastDisk()); // make sure that the start peg has less than goal peg } int n = startPeg.lastDisk(); startPeg.addDisk();
cout << " Move Disk " << n << " from peg" << startPeg.setName << " to peg" << endPeg.setName << endl; endPeg.addDisk(n); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
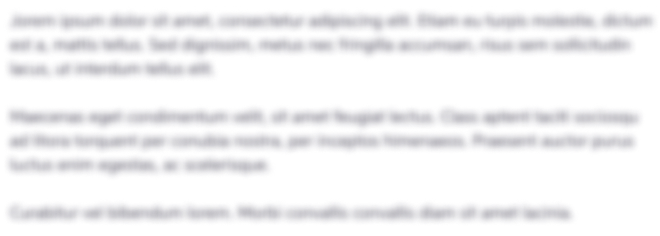
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started