Question
In-Lab Exercise (10 points) Submit the solution files of the Time class operator overloading before the due date/time. Please upload the modified Time.h and Time.cpp
In-Lab Exercise (10 points) Submit the solution files of the Time class operator overloading before the due date/time. Please upload the modified Time.h and Time.cpp to Canvas. In this exercise, you'll implement operator overloading for the Time class that we discussed in class. Download the Time.h and Time.cpp files from Canvas and add the necessary operator functions. A driver program (Lab5.cpp) is also available on Canvas for testing purposes. The following are the three operators that will overload the Time class. Operator +=. This operator forwards a time object by a given number of minutes. Example: nowTime.Set(11,10,P); // At this point nowTime represents the time 11:10 PM. Then the following operation is performed: nowTime += 30; // Now the nowTime should be 11:40 PM. Operator +. This operator adds an integer to a time object and returns the resulting time object: forwards the left operand time object by the number of minutes provided by the right operand (i.e the integer). Note that the reversal of these operators' orders will be syntactically incorrect. The following shows an example of using this operator. nowTime.Set(11,10,P); // At this point nowTime represents the time 11:10 PM. Then the following operation is performed: Time anotherTime = nowTime + 30; // Now the anotherTime should be 11:40 PM. // IMPORTANT: nowTime is still 11:10 PM. // Note: Time anotherTime = 30 + nowTime; is incorrect syntax. Operator =. This operator assigns an integer to a time object. Let t be a Time object and x be an integer between 1 and 12, then "t = x;" should set t to x:00 AM. If t is not between 1 and 12, then the assignment should set the time to 12:00 AM. The following shows an example of using this operator. Time mealTime(4,0,'P'); mealTime = 8; // Will set mealTime to 8 AM. mealTime = 13; // Will set mealTime to 12 AM. Add your code to time.cpp and time.h. Submit the modified Time.cpp and Time.h.
--------------------------------------------------------
Time.h
#pragma once #includeusing namespace std; class Time { public: // Constructors Time(); Time(unsigned hours, unsigned minutes, char am_pm); // Functions to get the values of member unsigned getHours() const; // Return the hour number unsigned getMinutes() const; // Return the minute number char getAMorPM() const; // Return the AM PM value // Set, Display as discussed in class void Set(unsigned hours, unsigned minutes, char am_pm);//Set the time void Display() const; //Display the time // A convenient forward function void Forward(int minutesToForward); //Forward the time private: unsigned myHours, myMinutes; char myAMorPM; };
--------------------------------------------------------
Time.cpp
#include "Time.h" #include#include using namespace std; // Set the objectfs data values. void Time::Set(unsigned hours, unsigned minutes, char am_pm) { if (hours >= 1 && hours <= 12 && minutes >= 0 && minutes <= 59 && (am_pm == 'A' || am_pm == 'P')) { myHours = hours; myMinutes = minutes; myAMorPM = am_pm; } else cerr << "*** Can't set these values *** "; } // Display the time represented by the object void Time::Display() const { string minStr = to_string(myMinutes); if (myMinutes == 0) { minStr = "00"; } cout << myHours << ':' << minStr << ' ' << myAMorPM << ".M." << endl; } unsigned Time::getHours() const { return myHours; } unsigned Time::getMinutes() const { return myMinutes; } char Time::getAMorPM() const { return myAMorPM; } // Forward the time by a given number of minutes void Time::Forward(int minutesToForward) { int totalMinutes = myMinutes + minutesToForward; myMinutes = totalMinutes % 60; int hoursAdded = totalMinutes / 60; int nowHours = myHours + hoursAdded; int flipAmPm = ((nowHours / 12) - (myHours / 12)) % 2; if (flipAmPm == 1) { if (myAMorPM == 'A') { myAMorPM = 'P'; } else { myAMorPM = 'A'; } } myHours = nowHours % 12; if (myHours == 0) { myHours = 12; } } Time::Time() { myHours = 12; myMinutes = 0; myAMorPM = 'A'; } Time::Time(unsigned hours, unsigned minutes, char am_pm) { if (hours >= 1 && hours <= 12 && minutes >= 0 && minutes <= 59 && (am_pm == 'A' || am_pm == 'P')) { myHours = hours; myMinutes = minutes; myAMorPM = am_pm; } else { cerr << "*** Can't set these values *** "; Set(12, 0, 'A'); } }
------------------------------------------------------
Driver Code
#include
--------------------------------------------------
What am i supposed to do/ implement?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
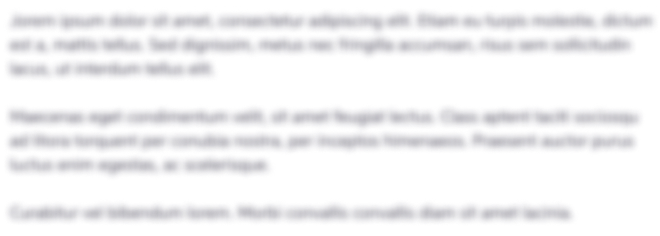
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started