Question
Input is from a file called num1.in Input consists of 4 doubles representing the radii of 4 circles. You are to make an array of
Input is from a file called num1.in Input consists of 4 doubles representing the radii of 4 circles. You are to make an array of circles and then print out the radius followed by the circumference, one per line. So the output looks like this:
3.5 21.991148575128552 0.8 5.026548245743669 2.15 13.50884841043611 0.7 4.39822971502571
Circle.java
public class Circle { //attribute private double radius; //constructors public Circle() {radius=0.0; } public Circle(double r) {radius=r; } //accessors public double getRadius() { return radius; } //mutators public void setRadius(double r) { radius = r; } //methods public double circumference() { return 2*Math.PI*radius; } public double area() { return Math.PI*radius* radius; } }
Lab5Num2.java
public class Lab5Num2 {
public static void main(String[] args) { //declare input file. DO this exactly as you did in netBeans. Try/Catch. Don't forget imports //create (declare) an array that can hold Circle objects //input 4 Circle objects into the array //DO THE REST AFTER YOU HAVE CREATED THE ARRAY //output the radius and circumference of each member of the array starting with the LAST ONE } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
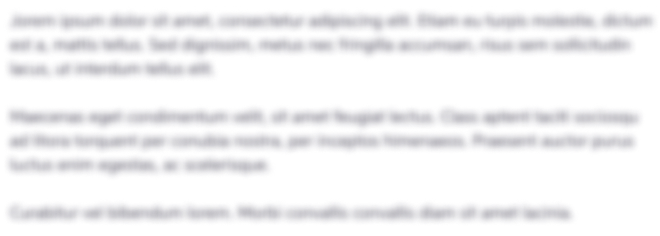
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started