Question
Instructions: When the program starts, the program should read from a textfile at least 16 products with their names, prices, and quantities being purchased (quantities
Instructions:
When the program starts, the program should read from a textfile at least 16 products with their names, prices, and quantities being purchased (quantities initially should equal 0). As the data is read, it should be added into the binary search tree. Arrange the data so that the tree will be reasonably balanced. Use the name of the product as the sorting key for the binary search tree.
After the data has been loaded from the text file, a menu should appear that prompts the user to (1) add a product, (2) edit a product, (3) find and display a product, (4) view all products, (5) delete a product, (6) find and purchase a product, (7) display the current order, (8) clear the current order, or (9) quit the program.
When the program quits, the products in the current program, including those just added and having removed all those removed, are saved to the text file so that the updated collection of products will be loaded when the program runs the next time. The quantities should all be set to 0 when saving the text file.I
Additional requirements (to practice traversals): Your tree of products should have a destructor that uses a post-order traversal strategy. The view all products menu option should use an in-order traversal strategy. When displaying the current order, use a pre-order traversal strategy. When clearing the current order use a level traversal strategyInstructions:
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
In C++, I am trying to make a binary search tree with a menu for a product catalogue. I am supposed to read and write to the textfile I have. The first problem I am having is that it is saying I don't have any data. There is also other errors in my code. Please HELP!!!
This is my productlist.txt:
TShirt 7 0 BlueJeans 14 0 Shorts 20 0 Khaki's 25 0 ButtonDownShirt 30 0 NikeShoes 85 0 AdidasShoes 75 0 Hat 15 0 Underwear 10 0 Socks 5 0 Scarf 6 0 Gloves 3 0 Jacket 45 0 Boots 100 0 Pajamas 30 0 Vest 50 0
Here is my code:
//Binary Search Tree Program
#include
typedef string treeType;
class Products { private: string name; int price; int quantity; public: Products(); Products(string name, int price, int quantity); string getName(); int getprice(); int getquantity(); void setName(string newname); void setprice(int newprice); void setquantity(int newquantity); };
class BinarySearchTree { private: struct tree_node { string key; tree_node* left; tree_node* right; //treeType data; Products data;
}; tree_node* root;
public: BinarySearchTree() { root = NULL; }
bool isEmpty() const { return root == NULL; } void print_inorder(); void inorder(tree_node*); void print_preorder(); void preorder(tree_node*); void print_postorder(); void postorder(tree_node*); void levelOrder(tree_node* n); void insert(Products); void remove(string); void search(string key); void changeprice(string key, int newprice); void edit(string key, string newname, int newprice); void displaytree(tree_node*); };
Products::Products() { }
Products::Products(string newname, int newprice, int newquantity) { name = newname; price = newprice; quantity = newquantity; }
string Products::getName() { return name; }
int Products::getprice() { return price; }
int Products::getquantity() { return quantity; }
void Products::setName(string newname) { name = newname; }
void Products::setprice(int newprice) { price = newprice; }
void Products::setquantity(int newquantity) { quantity = newquantity; }
// Smaller elements go left // larger elements go right void BinarySearchTree::insert(Products p) { tree_node* t = new tree_node; tree_node* parent; t->data = p; t->left = NULL; t->right = NULL; parent = NULL;
// is this a new tree? if (isEmpty()) root = t; else { //Note: ALL insertions are as leaf nodes tree_node* current; current = root; // Find the Node's parent while (current) { parent = current; if (t->data.getName() > current->data.getName()) current = current->right; else current = current->left; }
if (t->data.getName() < parent->data.getName()) parent->left = t; else parent->right = t; } }
void BinarySearchTree::remove(string p) { //Locate the element bool found = false; if (isEmpty()) { cout << " This Tree is empty! " << endl; return; }
tree_node* current; tree_node* parent; current = root; parent = root;
while (current != NULL) { if (current->data.getName() == p) { found = true; break; } else { parent = current; if (p > current->data.getName()) current = current->right; else current = current->left; } } if (!found) { cout << " Data not found! " << endl; return; }
// 3 cases : // 1. We're removing a leaf node // 2. We're removing a node with a single child // 3. we're removing a node with 2 children
// Node with single child if ((current->left == NULL && current->right != NULL) || (current->left != NULL && current->right == NULL)) { if (current->left == NULL && current->right != NULL) { if (current->left == current) { parent->left = current->right; delete current; } else { parent->right = current->right; delete current; } } else // left child present, no right child { if (parent->left == current) { parent->left = current->left; delete current; } else { parent->right = current->left; delete current; } } return; }
//We're looking at a leaf node if (current->left == NULL && current->right == NULL) { if (parent->left == current) { parent->left = NULL; } else { parent->right = NULL; } delete current; return; }
//Node with 2 children // replace node with smallest value in right subtree if (current->left != NULL && current->right != NULL) { tree_node* chkr; chkr = current->right; if ((chkr->left == NULL) && (chkr->right == NULL)) { current = chkr; delete chkr; current->right = NULL; } else // right child has children { //if the node's right child has a left child // Move all the way down left to locate smallest element
if ((current->right)->left != NULL) { tree_node* lcurr; tree_node* lcurrp; lcurrp = current->right; lcurr = (current->right)->left; while (lcurr->left != NULL) { lcurrp = lcurr; lcurr = lcurr->left; } current->data = lcurr->data; delete lcurr; lcurrp->left = NULL; } else { tree_node* tmp; tmp = current->right; current->data = tmp->data; current->right = tmp->right; delete tmp; }
} return; }
}
void BinarySearchTree::print_inorder() { inorder(root); }
void BinarySearchTree::inorder(tree_node* p) { if (p != NULL) { if (p->left) inorder(p->left); cout << " " << p->data.getName() << " "; if (p->right) inorder(p->right); } else return; }
void BinarySearchTree::edit(string p, string newname, int newprice) { bool found = false; if (isEmpty()) { cout << " This Tree is empty! " << endl; return; }
tree_node* curr; tree_node* parent; curr = root;
while (curr != NULL) { if (curr->data.getName() == p) { found = true; break; } else { parent = curr; if (p>curr->data.getName()) curr = curr->right; else curr = curr->left; } } if (!found) { cout << " Person not found. " << endl; return; }
//change the phonenumber associated with the node curr->data.setName(newname); cout << "The name has changed succesfully " << endl; }
void BinarySearchTree::print_preorder() { preorder(root); }
void BinarySearchTree::preorder(tree_node* p) { if (p != NULL) { cout << " " << p->data.getName() << " "; if (p->left) preorder(p->left); if (p->right) preorder(p->right); } else return; }
void BinarySearchTree::print_postorder() { postorder(root); }
void BinarySearchTree::postorder(tree_node* p) { if (p != NULL) { if (p->left) postorder(p->left); if (p->right) postorder(p->right); cout << " " << p->data.getName() << " "; } else return; }
// Print the tree level-order assisted by queue void BinarySearchTree::levelOrder(tree_node* n) { // Create a queue queue
// Push the root q.push(n);
while (!q.empty()) { // Dequeue a node from front tree_node* v = q.front(); cout << v->key << endl;
// Enqueue the left children if (v->left != NULL) { q.push(v->left); }
// Enqueue the right children if (v->right != NULL) { q.push(v->right); }
// Pop the visited node q.pop(); } }
void BinarySearchTree::search(string key) { bool found = false; if (isEmpty()) { cout << " This Tree is empty! " << endl; return; }
tree_node* curr; tree_node* parent; curr = root;
while (curr != NULL) { if (curr->data.getName() == key) { found = true; cout << "The price for " << key << " is " << curr->data.getprice() << endl; break; } else { parent = curr; if (key>curr->data.getName()) curr = curr->right; else curr = curr->left; } } if (!found) { cout << " Data not found! " << endl; return; } }
void BinarySearchTree::changeprice(string p, int newprice) { bool found = false; if (isEmpty()) { cout << " This Tree is empty! " << endl; return; }
tree_node* curr; tree_node* parent; curr = root;
while (curr != NULL) { if (curr->data.getName() == p) { found = true; break; } else { parent = curr; if (p>curr->data.getName()) curr = curr->right; else curr = curr->left; } } if (!found) { cout << " Name not found. " << endl; return; }
//change the price associated with the node curr->data.setprice(newprice); cout << "Price changed successfully. " << endl; }
void fillTree(BinarySearchTree *b) { ifstream file; file.open("productlist.txt"); if (!file) { cout << " Error opening file. " << endl; } //variables used to load data into the tree string name; int price; int quantity; Products p; while (file >> name >> price>> quantity) { p.setName(name); p.setprice(price); p.setquantity(quantity); cout << p.getName() << p.getprice() << p.getquantity()<< endl; (*b).insert(p); } file.close(); }
void BinarySearchTree::displaytree(tree_node* begin) //this displays the items in the list at a given time. It is used throughout the whole program to keep the user informed { //about what is in the list. tree_node *p; if (begin == NULL) { cout << "List is empty "; return; } p = begin; while (p != NULL) { cout << p->key << ", "; p = p->right; } cout << " "; cout << "--------------------------------------------------- ";
} int main() {
BinarySearchTree b; int ch; string name; string key; key = name; int price; Products tmp; Products tmp1; fillTree(&b); b.print_preorder(); while (1) { cout << endl << endl; cout << " Binary Search Tree Operations " << endl; cout << " ----------------------------- " << endl; cout << " 1. Add a Product " << endl; cout << " 2. Edit a product/Change Price " << endl; cout << " 3. Search for a product " << endl; cout << " 4. View all Products " << endl; cout << " 5. Delete a product " << endl; cout << " 6. Find and Purchase a Product " << endl; cout << " 7. Display current list " << endl; cout << " 8. Clear the current order " << endl; cout << " 9. Exit " << endl; cout << " Enter your choice : "; cin >> ch; switch (ch) { case 1: cout << " Enter name of product to be added: "; cin >> name; cout << endl << " Enter price of the product(Do Not Use the dollar sign): " << endl; cin >> price; tmp.setName(name); tmp.setprice(price); b.insert(tmp); break; case 2: cout << " Enter the name of the product whose price you wish to edit: " << endl; cin >> name; cout << endl << " Enter the new price(Do not use the dollar sign): " << endl; cin >> price; cout << endl; b.changeprice(name, price); break; case 3: cout << " Enter the name of the product you wish to search for: " << endl; cin >> key; b.search(key); break; case 4: cout << endl; cout << " The products consist of: " << endl; cout << fillTree << endl; cout << " -------------------" << endl; b.print_preorder(); break; case 5: cout << " Enter data to be deleted : "; cin >> key; b.remove(key); b.print_preorder(); //b.displaytree(); break; case 6: cout << "Which product would you like to search for, here is the current list: " << endl; //b.displaytree(); cin >> key; cout << "Here is your product with the price: " << endl; b.search(key); break; case 7: cout << "The current order is: " << endl; b.print_preorder(); // b:.displaytree(); case 8: cout << " Clear the current order: " << endl; // b.levelOrder(); case 9: return 0; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
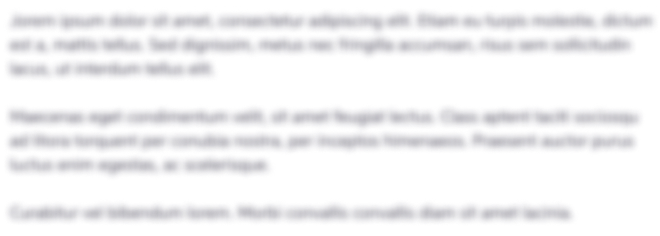
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started