Question
Introduction The aim of this assignment is to have you work with Object Oriented C++ with simple inheritance. Objectives ? Build a Point3D Class ?
Introduction The aim of this assignment is to have you work with Object Oriented C++ with simple inheritance.
Objectives
? Build a Point3D Class
? Build a Vector3D Class that inherits from Point3D
? Demonstrate the classes working
Outcomes:
? Creation and use of classes
? Inheritance
? Pointer use, management and manipulation
Description: You will create two classes for this project. The first will be a basic 3D CartesianCoordinate that stores and X,Y,Z value and has a distance formula function to get the distance between two points.
The second class is a 3D Vector class that will do basic vector math. It will inherit from the Cartesian coordinate since both share a similar base.
Specifications
Point3D.h/.cpp
This class will be a 3D Cartesian-Coordinate
Properties:
? double x,y,z
Method:
? Constructor taking in x,y,z
? Getters and Setters
double GetDistance(Point3D*)
Vector3D.h/.cpp
This class inherits from Point3D since it also contains: X,Y,Z values and requires a distance formula.
Further Properties:
? double size the size of the vector
? double ux,uy,uz the vector components as a unit vector
? double angle angle in radians
Further Methods
? Vector3D(x,y,z) - Constructor that takes in X,Y,Z then calculates size, angle and unit vector
? Vector3D(p1,p2) - Constructor that takes in two Point3Ds and makes a vector from them
? private CalculateSize()
? private CalculateUnitVector()
? public Vector3D* getUnitVector()- create and return the unit vector
? public double dotProduct(Vector3D* other) returns dot product
? public Vector3D* crossProduct(Vector3D* other) returns cross product vector
? public Vector3D* add(Vector3D* other) add two vectors together get a third
? public Vector3D* subtract(Vector3D* other) subtract two vectors together get a third
? public void scale(double value) multiply the vector by the value, dont forget you might need to recalculate angle, size and unit vector components
? Getters and setters
? Anything else you need to make these things work!
Main.cpp
Create a simple program showing off ALL the functionality of your classes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
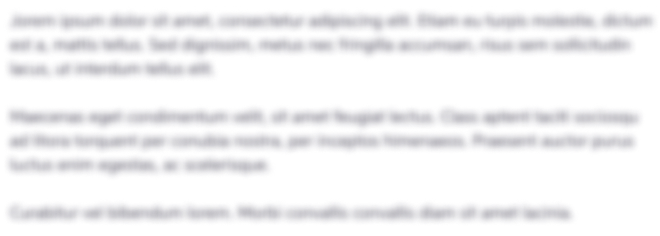
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started