Question
JAVA 3 METHODS STABLE MATCHING - HELPER CLASSES PROVIDED BELOW (Preferences.java & Cost.java) In this problem we will consider a version of the problem for
JAVA 3 METHODS STABLE MATCHING - HELPER CLASSES PROVIDED BELOW (Preferences.java & Cost.java)
In this problem we will consider a version of the problem for professors and students and their fully ordered list of preferences.
The Stable Matching Problem, as discussed in the text, assumes that all men and women have a fully ordered list of preferences. In this problem we will consider a version of the problem for professors and students and their fully ordered list of preferences. Note that ties in preference lists are not allowed. As before we have a set P of n professors and a set S of n students. Assume each professor and each student ranks the members of the opposite group.
Part 1: Implement a Brute Force Solution A brute force solution to this problem involves generating all possible permutations of men and women, and checking whether each one is a stable matching, until a stable matching is found. For this assignment, you are provided with Preferences.java class which includes the necessarry input structures for the problem. Please see the comments in Preferences.java file for details. You are also given Assignment1.java file where you will put your implementation for this part under stableMatchBruteForce() function which returns ArrayList
Part 2: Implement Gale-Shapley Algorithm In order to solve this matching problem more efficiently, you need to implement Gale-Shapley Al- gorithm and give a solution for Professors Optimal Matching. For implementation, we provide you with again with Preferences.java and you will put your implementation to Assignment1.java file under stableMatchGaleShapley(). This function will again return ArrayList, described in previous part. Keep note that your algorithm should run in O(n2) time. Make sure that a student can compare between his current professor and another professor in constant time.
Part 3: Matching with Costs In this part, in addition to the previous part, we will be having costs for the stable matching algo- rithm. What this means is that there are going to be two stable matching combinations (one will be Professors Optimal and one will be Students Optimal). The way we calculate the costs is as follows.
Lets take the example of a professor. Suppose his preference list is {s1,s2,s3,s4,s5}. If the final pair comes to be (p1,s4), then cost of this for professor is going to be 3. (4-1=3). Similarly suppose if the preference list of student 4 was {p3,p2,p1,p4,p5}, then his cost will be 2. Thus the cost of a pair to someone is the difference in rank of his most preferred choice and rank of the person currently assigned to him.
Now, you need to apply the stable matching algorithm for both professors optimal and students optimal. The output will be the stable pairs and their costs. Your output should look like this (p1,s4,3,1) where this result shows index or professor, index of student, cost to professor and cost to student respectively. You should put your implementation to same file(Assignment1.java) under stableMatchCosts() function. For output, you are provided with Cost.java class and the function should return an ArrayList of Costs. Your input to the function will be same as previous part, an instance of Preferences class.
METHODS TO BE IMPLEMENTED:
1) // Part1: Implement a Brute Force Solution public static ArrayList
2) // Part2: Implement Gale-Shapley Algorithm public static ArrayList
3) // Part3: Matching with Costs public static ArrayList
PREFERENCES.JAVA
import java.util.ArrayList;
/** * Class to provide input to Stable Matching algorithms */ public class Preferences { /** Number of professors. */ private int numberOfProfessors;
/** Number of students. */ private int numberOfStudents;
/** A list containing each professor's preference list. */ private ArrayList
/** A list containing each student's preference list. */ private ArrayList
public Preferences(int numberOfProfessors, int numberOfStudents, ArrayList
public int getNumberOfProfessors() { return numberOfProfessors; }
public void setNumberOfProfessors(int numberOfProfessors) { this.numberOfProfessors = numberOfProfessors; }
public int getNumberOfStudents() { return numberOfStudents; }
public void setNumberOfStudents(int numberOfStudents) { this.numberOfStudents = numberOfStudents; }
public ArrayList
public void setProfessors_preference(ArrayList
public ArrayList
public void setStudents_preference(ArrayList
COST.JAVA
/** * Cost object for Assignment 1 - Part 3 * This object helps you to return 4 fields at once. * The meaning of cost is described in the assignment. */ public class Cost { private int indexOfProfessor; private int indexOfStudent; private int costToProfessor; private int costToStuent;
public Cost(int indexOfProfessor, int indexOfStudent, int costToProfessor, int costToStuent) {
this.indexOfProfessor = indexOfProfessor; this.indexOfStudent = indexOfStudent; this.costToProfessor = costToProfessor; this.costToStuent = costToStuent; }
public int getIndexOfProfessor() { return indexOfProfessor; }
public void setIndexOfProfessor(int indexOfProfessor) { this.indexOfProfessor = indexOfProfessor; }
public int getIndexOfStudent() { return indexOfStudent; }
public void setIndexOfStudent(int indexOfStudent) { this.indexOfStudent = indexOfStudent; }
public int getCostToProfessor() { return costToProfessor; }
public void setCostToProfessor(int costToProfessor) { this.costToProfessor = costToProfessor; }
public int getCostToStuent() { return costToStuent; }
public void setCostToStuent(int costToStuent) { this.costToStuent = costToStuent; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
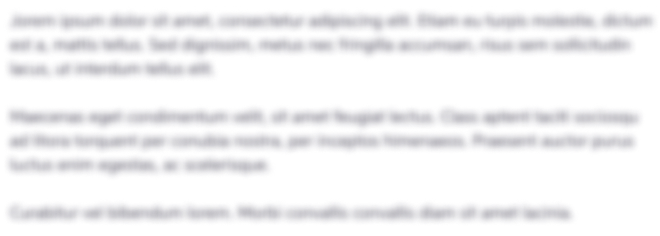
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started