Question
Java Assignment I was supposed to have two separate java classes (calculator and main method) but I missed it and did only one. Below are
Java Assignment I was supposed to have two separate java classes (calculator and main method) but I missed it and did only one. Below are the instructions and my code.
For this assignment we're going to make another calculator. This one will be a simple four function (add, subtract, multiply, and divide) calculator but it will have state. Specifically, the calculator will keep track of the result of the most recent operation and use that value as the first operand for the next operation. Your new calculator class should have the following fields and methods. Field private double currentValue
Methods
public static int displayMenu()
public static double getCurrentValue()
public void add(double operand2)
public void subtract(double operand2)
public void multiply(double operand2)
public void divide(double operand2)
public void clear()
The display Menu option should allow the user to pick from the options listed (add, subtract, multiply, divide, clear and quit). If the user enters an invalid option, it should reprompt them. Once a valid option has been entered, the method should return it. The getOperand method displays the prompt to the user, reads in a double value, and returns it. The getCurrentValue method just returns the number stored in the currentValue field. The add, subtract, multiply and divide methods now only need to take one parameter because the first operand will be the currentValue field. Also, these methods do not need to return the result. They should store it in the currentValue field. The clear method will reset the currentValue to zero. DO NOT make the currentValue field static. With currentValue as an instance field, we can eventually write a program that allows users to have multiple calculators running. Note that because currentValue is an instance field, the methods that change it must be instance methods. Methods such as displayMenu and getOperand can remain static because they do not need to access the state of the calculator. Write a main method that creates an instance of your calculator class. Write a loop that displays the current value (initially zero), asks the user what operation they want to perform, computes the result and repeasts until the user chooses to quit. If dividing by 0, it should return "The current value is NaN."
Code Starts here.
import java.util.Scanner;
public class Memory {
public double currentValue=0; //Variable to hold result
public static void main(String[] args) // Main function
{
Scanner choose = new Scanner(System.in);
int choice = 0;
String prompt;
boolean right = false;
double operand2;
do // Loop until user wants to quit
{
System.out.println("The current value is " + currentValue); //Displaying current value
choice = displayMenu(choose); //Displaying the user's menu
if ((choice <= 6) && (choice > 0)) //Checking for a valid option to be selected
{
switch(choice) //Calling the appropriate function
{
case 1: operand2 = getOperand("What is the second number? ");
add(operand2);
break;
case 2: operand2 = getOperand("What is the second number? ");
subtract(operand2);
break;
case 3: operand2 = getOperand("What is the second number? ");
multiply(operand2);
break;
case 4: operand2 = getOperand("What is the second number? ");
divide(operand2);
break;
case 5: case 6: currentValue = 0;
System.out.println("Goodbye!");
break;
}
}
else
{
right = true;
System.out.println("I'm sorry, " + choice + " wasn't one of the options. Please try again.");
}
}while (choice != 6);
}
public static int displayMenu(Scanner choose) // Displaying the user's menu
{
int choice;
System.out.println(" ");
System.out.println("Menu ");
System.out.println("1. Add");
System.out.println("2. Subtract");
System.out.println("3. Multiply");
System.out.println("4. Divide");
System.out.println("5. Clear");
System.out.println("6. Quit");
System.out.println(" ");
System.out.print("What would you like to do? ");
choice = choose.nextInt();
return choice;
}
public static double getOperand(String prompt)
{
Scanner choose = new Scanner(System.in);
System.out.print(prompt);
double option = choose.nextDouble();
return option;
}
public double getCurrentValue()
{
return currentValue;
}
public void add(double operand2)
{
currentValue += operand2;
}
public void subtract(double operand2)
{
currentValue -= operand2;
}
public void multiply(double operand2)
{
currentValue *= operand2;
}
public void divide(double operand2)
{
if (operand2 == 0)
{
System.out.println("The current value is NaN. ");
}
else
{
currentValue /= operand2;
}
}
public void clear()
{
currentValue = 0;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
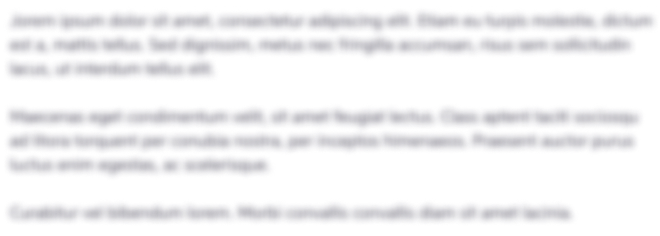
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started