Question
Java BlueJ Programming Lab 3 Solitaire Purpose The purpose of this lab is for you to work with array lists. Problem specification You will model
Java BlueJ Programming
Lab 3 Solitaire
Purpose
The purpose of this lab is for you to work with array lists. Problem specification You will model a particular type of solitaire. The game starts with 45 cards. (They need not be playing cards. Unmarked index cards work just as well.) Randomly divide them into some number of piles of random size. For example, you might start with piles of size 20, 5, 1, 9 and 10. In each round, you take one card from each pile, forming a new pile with these cards. For example, the sample starting configuration would be transformed into piles of size 19, 4, 8, 9 and 5. The solitaire is over when the piles have size 1, 2, 3, 4, 5, 6, 7, 8, and 9, in some order. (It can be shown that you always end up with such a configuration.)
You will write a Solitaire class to implement this behavior.
The SolitaireTester class
You are given a SolitaireTester class that uses Solitaire. SolitaireTester code may not be changed in any way
public class SolitaireTester
{
public static void main(String[] args)
{
Solitaire s = new Solitaire();
System.out.println("Start: " + s.toString());
int rounds = 0;
while (!s.over()) {
s.round();
++rounds;
System.out.println(rounds + ": " + s.toString());
}
System.out.println("Rounds: " + rounds);
}
}
SolitaireTester produces a random starting configuration and prints it. Then keeps applying the solitaire step and prints the result. Stops when the solitaire final configuration is reached and prints the number of rounds.
A run of the program will look something like (many lines omitted for clarity):
Start: [11, 12, 22]
1: [10, 11, 21, 3]
2: [9, 10, 20, 2, 4]
3: [8, 9, 19, 1, 3, 5]
4: [7, 8, 18, 2, 4, 6]
5: [6, 7, 17, 1, 3, 5, 6]
6: [5, 6, 16, 2, 4, 5, 7]
7: [4, 5, 15, 1, 3, 4, 6, 7]
8: [3, 4, 14, 2, 3, 5, 6, 8]
9: [2, 3, 13, 1, 2, 4, 5, 7, 8]
10: [1, 2, 12, 1, 3, 4, 6, 7, 9]
. . .
55: [1, 2, 4, 4, 4, 6, 7, 8, 9]
56: [1, 3, 3, 3, 5, 6, 7, 8, 9]
57: [2, 2, 2, 4, 5, 6, 7, 8, 9]
58: [1, 1, 1, 3, 4, 5, 6, 7, 8, 9]
59: [2, 3, 4, 5, 6, 7, 8, 10]
60: [1, 2, 3, 4, 5, 6, 7, 9, 8]
Rounds: 60
The Solitaire class
Here are an outline and some hints for Solitaire:
Constants: As required.
Exactly and only the following instance variable is required:
private ArrayList
constructor()
Initialize piles to a random number of piles of random size, but exactly 45 cards total. Use Math.random() as you do this. random() returns a double value greater than or equal to 0.0 and less than 1.0. As an example, to set num to a random integer value between 1 and 10 inclusive is:
num = (int) (Math.random() * 10) + 1;
toString()
Return a string representation of a Solitaire object. To do this, simply:
return piles.toString();
over()
Return true if the solitaire is over, false otherwise.
The solitaire is over when the piles have size 1, 2, 3, 4, 5, 6, 7, 8, and 9, in some order. (It can be shown that you always end up with such a configuration.)
round()
In each round, you take one card from each pile, forming a new pile with these cards.
Be very careful here when you remove a pile that reaches a size of 0 cards. Realize that when you remove() an element from an ArrayList elements move down, changing their indexes.
Required
Your program must look like the work of a professional computer programmer. You will lose points if you do not meet ALL of the following requirements:
clear, consistent layout and indentation
use Javadoc documentation comments as you comment every class, every method and anything that is not simple and clear
clear, meaningful variable and method names design simple, clear algorithms
a method must do only a single task, and must be simple and short
use constants to avoid hard-coded magic numbers
Step by Step Solution
There are 3 Steps involved in it
Step: 1
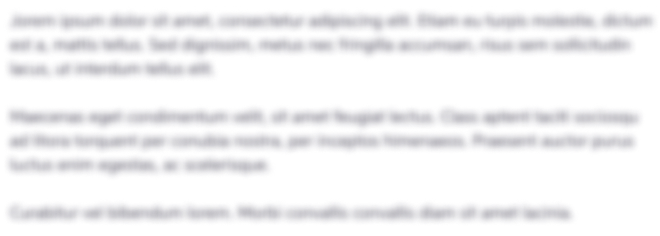
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started