Question
Java coding. No hardcoding and only helper functions allowed package questions; import java.util.ArrayList; import other_classes.Reply; import other_classes.User; public class Review { private ArrayList replies; //
Java coding. No hardcoding and only helper functions allowed
package questions;
import java.util.ArrayList;
import other_classes.Reply; import other_classes.User;
public class Review { private ArrayList
/** * 8 marks * This method should return an ArrayList which contains the unique User objects * which have posted a Reply within the Review * * @return an ArrayList of User objects who have posted within the Review * * Note that there should be no duplicates within the returned ArrayList * */ public ArrayList
Testing:
package testclients;
import java.util.ArrayList;
import other_classes.Data; import other_classes.Reply; import other_classes.User; import questions.Review;
public class ReviewClient {
public static void main(String[] args) {
// This file can be used to test your Review.java solutions
// First lets create a Review Object (for more information read Data.java) Review r1 = Data.review1(); // Checking totalReplies: System.out.println("--- Testing totalReplies ---"); System.out.println(r1.totalReplies(Data.getUser(4))); // Has replies System.out.println(r1.totalReplies(Data.getUser(5))); // Has no replies System.out.println(r1.totalReplies(Data.getUser(1))); // Has 1 reply // Checking findRepliesBy System.out.println("--- Testing findRepliesBy ---"); // This user has posts for(Reply r : r1.findRepliesBy(Data.getUser(1))){ System.out.println(r); } // This user has no posts for(Reply r : r1.findRepliesBy(Data.getUser(0))){ System.out.println(r); } // Checking findReplies System.out.println("--- Testing findReplies ---"); // This user has posts for(Reply r : r1.findReplies("")){ System.out.println(r); } // This user has no posts for(Reply r : r1.findReplies("")){ System.out.println(r); } System.out.println("--- Testing uniqueUsers ---"); for(User u : r1.getUniqueUsers()){ System.out.println(u); } // Checking cleanReplies: r1.cleanReplies("No change"); // This should not effect anything r1.cleanReplies("bad"); // This should remove the word "bad" from all Replies
// Checking clearReplies: ArrayList
} }
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- Required Output ReviewClient.java
Below is the required output when executing ReviewClient.java:
--- Testing totalReplies ---
4
0
1
--- Testing findRepliesBy ---
Kiera Moses: This was a bit rude
Lincoln Walter: I thought this movie was bad
--- Testing findReplies ---
Amaya Lyons: Thank you for taking the time to write a review of the movie.
Lincoln Walter: I thought this movie was bad
Kiera Moses: This was a bit rude
Amaya Lyons: We appreciate your honest review of the film.
Amaya Lyons: Thank you for your review.
Jacquelyn Mendez: Great review!
Gerald Garner: Nice to see that there were no insults
Troy Powell: I hope this gets cancelled
Emmett House: All I can say is bad bad bad
Amaya Lyons: This was not a bad review
Amaya Lyons: Thank you for taking the time to write a review of the movie.
Lincoln Walter: I thought this movie was bad
Kiera Moses: This was a bit rude
Amaya Lyons: We appreciate your honest review of the film.
Amaya Lyons: Thank you for your review.
Jacquelyn Mendez: Great review!
Gerald Garner: Nice to see that there were no insults
Troy Powell: I hope this gets cancelled
Emmett House: All I can say is bad bad bad
Amaya Lyons: This was not a bad review
--- Testing uniqueUsers ---
User: Amaya Lyons
User: Lincoln Walter
User: Kiera Moses
User: Jacquelyn Mendez
User: Gerald Garner
User: Troy Powell
User: Emmett House
--- Testing printReview, clearReplies and cleanReplies ---
Okay movie 6/10
Amaya Lyons: Thank you for taking the time to write a review of the movie.
Lincoln Walter: I thought this movie was
Amaya Lyons: We appreciate your honest review of the film.
Amaya Lyons: Thank you for your review.
Jacquelyn Mendez: Great review!
Emmett House: All I can say is
Amaya Lyons: This was not a review
Step by Step Solution
There are 3 Steps involved in it
Step: 1
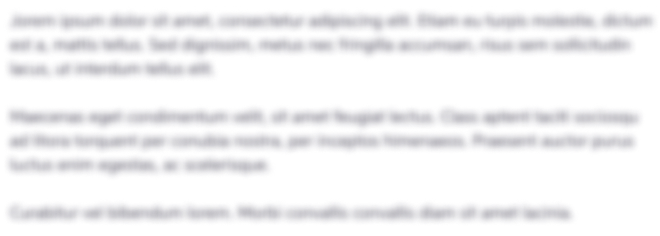
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started