Question
JAVA Given the Company and FullTimeEmployee classes and the Employee interface, write a Java program that reads in a file of full time employees with
JAVA Given the Company and FullTimeEmployee classes and the Employee interface, write a Java program that reads in a file of full time employees with each line of input containing the name of the employee (String) and gross Salary (double) and stores them in an array list. Then using the arraylist of stored employees, find and print the best paid employee (name) and his/her pay. If there is more than one such, print any one best paid employee information.
--------------------------------------------------------------------------------- import java.util.*; // for the Scanner class
import java.io.*; // for the FileNotFoundException class see Section 2.3
public class Company {
public static void main (String[ ] args) throws FileNotFoundException { new Company().run(); } // method main /** * Determines and prints out the best paid of the full-time employees * scanned in from a specified file. * */ public void run() throws FileNotFoundException // see Section 2.3 { final String INPUT_PROMPT = "Please enter the path for the file of employees: "; final String BEST_PAID_MESSAGE = " The best-paid employee (and gross pay) is "; final String NO_INPUT_MESSAGE = " Error: There were no employees scanned in."; String fileName; System.out.print (INPUT_PROMPT); fileName = new Scanner (System.in).nextLine(); Scanner sc = new Scanner (new File (fileName)); FullTimeEmployee bestPaid = findBestPaid (sc); if (bestPaid == null) System.out.println (NO_INPUT_MESSAGE); else System.out.println (BEST_PAID_MESSAGE + bestPaid.toString()); } // method run
/** * Returns the best paid of all the full-time employees scanned in. * * @param sc the Scanner object used to scan in the employees. * * @return the best paid of all the full-time employees scanned in, * or null there were no employees scanned in. * */ public FullTimeEmployee findBestPaid (Scanner sc) { FullTimeEmployee full, bestPaid = new FullTimeEmployee(); while (sc.hasNext()) { full = getNextEmployee (sc); if (full.getGrossPay() > bestPaid.getGrossPay()) bestPaid = full; } //while if (bestPaid.getGrossPay() == 0.00) return null; return bestPaid; } // method findBestPaid
/** * Returns the next full-time employee from the file scanned by a specified Scanner * object. * * @param sc the Scanner object over the file. * * @return the next full-time employee scanned in from sc. * */ protected /*private*/ FullTimeEmployee getNextEmployee (Scanner sc) { Scanner lineScanner = new Scanner (sc.nextLine()); String name = lineScanner.next(); double grossPay = lineScanner.nextDouble();
return new FullTimeEmployee (name, grossPay); } // method getNextEmployee
} // class Company
--------------------------------------------------------------------------------- import java.text.DecimalFormat;
public interface Employee {
final static DecimalFormat MONEY = new DecimalFormat (" $0.00"); // a class constant used in formatting a value in dollars and cents
/** * Returns this Employee objects name. * * @return this Employee objects name. * */ String getName();
/** * Returns this Employee objects gross pay. * * @return this Employee objects gross pay. * */ double getGrossPay();
/** * Returns a String representation of this Employee object with the name * followed by a space followed by a dollar sign followed by the gross * weekly pay, with two fractional digits (rounded). * * @return a String representation of this Employee object. * */ String toString();
} // interface Employee
--------------------------------------------------------------------------------- public class FullTimeEmployee implements Employee { protected /*private*/ String name; protected /*private*/ double grossPay; public FullTimeEmployee() { final String EMPTY_STRING = ""; name = EMPTY_STRING; grossPay = 0.00; } // default constructor
/** * Initializes this FullTimeEmployee object's name and gross pay from a * a specified name and gross pay. * * @param name - the specified name. * @param grossPay - the specified gross pay. * */ public FullTimeEmployee (String name, double grossPay) { this.name = name; this.grossPay = grossPay; } // 2-parameter constructor
/** * Returns the name of this FullTimeEmployee object. * * @return the name of this FullTimeEmployee object. * */ public String getName() { return name; } // method getName
/** * Returns the gross pay of this FullTimeEmployee object. * * @return the gross pay of this FullTimeEmployee object. * */ public double getGrossPay() { return grossPay; } // method getGrossPay
/** * Returns a String representation of this FullTimeEmployee object with the * name followed by a space followed by a dollar sign followed by the gross * weekly pay, with two fractional digits (rounded), followed by " FULL TIME". * * @return a String representation of this FullTimeEmployee object. * */ public String toString() { final String EMPLOYMENT_STATUS = " FULL TIME";
return name + MONEY.format (grossPay) + EMPLOYMENT_STATUS; // the format method returns a String representation of grossPay. } // method toString
} // class FullTimeEmployee ---------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
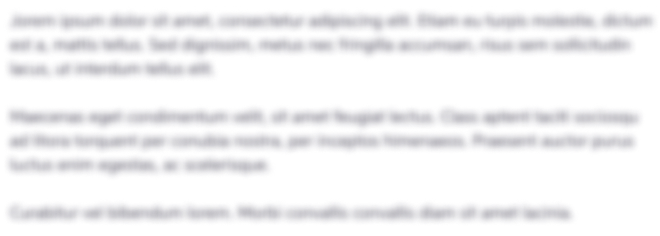
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started