Question
java I am trying to use a dice to determine each turn of a player in a game. Each player in the game is described
java
I am trying to use a dice to determine each turn of a player in a game. Each player in the game is described by a Player class, where a constructor has the dice value of the player
player class has the following code :
player() { dice = 0; }
public int getDice() { return dice; } public void setDice(int s) { diceValue = s; } public static int rollDice() { return dice = ThreadLocalRandom.current().nextInt(1, 7); } public static int playerOrder(player[] a, int numOfPlayers, int i) { a[i].setDice(FlipDice()); System.out.println("Player " + (i+1) +" rolled " + a[i].getDice()); return a[i].getDice(); }
so here the number of player[] is defined in the driver for an amount of players that was determined by a user (2-4 players).
In the driver, there is the following code:
//makes player objects where the number of players is determined by the user (can be from 2-4)
player[] users = new player[numOfPlayers];
for (int i = 0; i < users.length; i++) { users[i] = new player();
}
//here is code that needs to be filled: determine the order (so use the playerOrder method)
//fill code here, something like:
for (int b = 0; b< users.length; b++) player.playerOrder(users, i,numOfPlayers);
//then check that each player has a different dice value.
//fill code here
----
So the task is to create a java program, that rolls a dice and determines the order in which players turns will be. so if there are 4 players, a dice from 1-6 will roll a unique value for each of the 4 players. If when rolling the dice has the same dice values, the code must re-roll for there to be unique values among all 4 players. Please output updates to the user as, "player 1 rolled 6, player 2 rolled 5 ...", "there was a tie between rolls... re-rolling..." etc. The code above is some of the things that I did, they are a guide, but feel free to re-write things to make more sense. Also leave simple comments explaining the code as it will be helpful.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
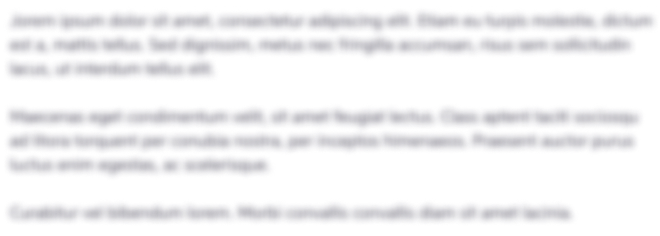
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started