Question
JAVA implemment the following: public static void printLeaves(). This method outputs the leaves of a binary tree from right to left. More specifically, the leaves
JAVA implemment the following:
public static void printLeaves().
This method outputs the leaves of a binary tree from right to left.
More specifically, the leaves should be printed in the reverse order that they would be printed using any of the standard traversals.
If the tree does not have any leaves (an empty tree), simply print: no leaves Note: when you print, use print NOT println
public static NumTree union(NumTree tree1, NumTree tree2)
The method accepts two NumTree as parameters and combines the two trees into a new third tree which is returned.
The new tree's structure should be a union of the structures of the two original trees.
It should have a node in any location where there was a node in either of the original trees (or both).
The nodes of the new tree should store an integer indicating which of the original trees had a node at that position
(1 if just the first tree had the node, 2 if just the second tree had the node, 3 if both trees had the node).
You may define private helper methods as needed. Your method should not change the structure or contents of either of the two trees being compared.
GIVEN___________
ManipulatingTree.java
public class ManipulatingTree{ public static void printLeaves(NumTree tree){ //TO DO } public static NumTree union(NumTree tree1, NumTree tree2){ //TO DO }
}
TreeNode.java
//source code is provided for reference only public class TreeNode implements Comparable{
private int data; private TreeNode left; private TreeNode right;
public TreeNode(int data){ this.data=data; left=right=null; }
public int getData(){ return data; } public TreeNode getLeft(){ return left; } public TreeNode getRight(){ return right; } public void setData(int data){ this.data = data; } public void setLeft(TreeNode left){ this.left = left; } public void setRight(TreeNode right){ this.right = right; } public int compareTo(TreeNode node){ return data-node.getData(); } }
NumTree.java
//For reference only public class NumTree{
private TreeNode root; public NumTree(){ root=null; }
public TreeNode getRoot(){ return root; } public void setRoot(TreeNode root){ this.root=root; } public boolean isEmpty(){ return root==null;} public int size(){ return sizeHelper(root); } private int sizeHelper(TreeNode node){ if(node==null) return 0; else return 1+sizeHelper(node.getLeft())+sizeHelper(node.getRight()); } public boolean search(int data){ return searchHelper(root, data); } private boolean searchHelper(TreeNode node, int data){ if(node==null) return false; if(node.getData()==data) return true; else if(node.getData() return searchHelper(node.getRight(),data); else return searchHelper(node.getLeft(), data); } public void add(int data){ root=addHelper(root, data); } private TreeNode addHelper(TreeNode node, int data){ if(node==null) node=new TreeNode(data); else if(data<=node.getData()) node.setLeft(addHelper(node.getLeft(), data)); else node.setRight(addHelper(node.getRight(), data)); return node; }
public void display(){ displayHelper2(root, 0); }
private void displayHelper(TreeNode t, int level){ if(t==null) return ; displayHelper(t.getRight(), level + 1); for(int k = 0; k < level; k++) System.out.print("\t"); System.out.println(t.getData()); displayHelper(t.getLeft(), level + 1); //recurse left } private void displayHelper2(TreeNode t, int level){ if(t==null) return ; displayHelper2(t.getRight(), level + 1); for(int k = 0; k < level; k++) System.out.print(" "); System.out.println(t.getData()); displayHelper2(t.getLeft(), level + 1); //recurse left } //remove a node from the tree public void delete(int data){ delete(new TreeNode(data)); } public void delete(TreeNode node){ if(root==null) return;// false; //found=false; root=remove(root, node); //return found; } private TreeNode remove(TreeNode tree, TreeNode node){ if(tree==null) return null; if(node.compareTo(tree)<0){ tree.setLeft(remove(tree.getLeft(), node)); }else if(node.compareTo(tree)>0){ tree.setRight(remove(tree.getRight(), node)); }else{ tree = removeNode(tree); //found=true; } return tree; } private TreeNode removeNode(TreeNode tree){ if(tree.getLeft()==null){ return tree.getRight(); }else if(tree.getRight()==null){ return tree.getLeft(); }else{ //if this node has both left and right child; we decided to use the largest node on the left TreeNode maxNodeOnLeft=getMaxNode(tree.getLeft()); tree.setData(maxNodeOnLeft.getData()); tree.setLeft(remove(tree.getLeft(), new TreeNode(maxNodeOnLeft.getData()))); return tree; } } private TreeNode getMaxNode(TreeNode tree){ while(tree.getRight() !=null) tree=tree.getRight(); return tree; } public void buildTree(int[] data){ for(int i=0; i add(data[i]); } public String toString(){ return printHelper(root); } private String printHelper(TreeNode node){ if(node ==null) return ""; else { return printHelper(node.getLeft())+node.getData()+printHelper(node.getRight()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
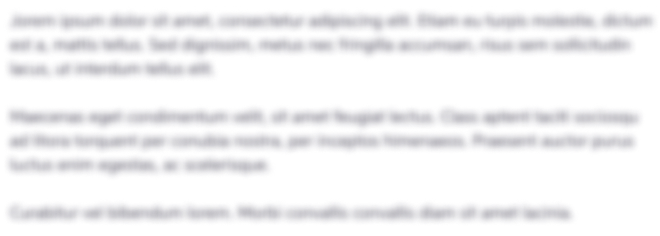
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started