Question
JAVA LANGUAGE You will begin with the attached Animal class, which is to be used as your Super Class (once you create your Java Project
JAVA LANGUAGE
You will begin with the attached Animal class, which is to be used as your Super Class (once you create your Java Project in Eclipse, you can save the Animal.java file to the project's src folder). Extend the animal class to create three particular animal classes, ex. Eagle, Wolf, Whale. Each class will be a subclass of the Animal class. Each class should:
Use the super class's constructor to initialize the Animal class's data fields.
Create at least two new statistics fields that are applicable to that animal (ex. a Wolf has a coloration and an age.)
Create getter and setter methods for each new data field.
Create at least two new methods relevant to that animal (ex. a Wolf can jump and howl.)
Override the Animal class's toString() method to create a string that is greatersuitable for this unique animal.
You should also create a Zoo class (you may name it something different) to exhibit how all of your animal classes work. Create at least two situations of every of your animals and check the various methods.
As stated above, you must also use the Javadoc style remarks to file your code. In Eclipse, you can type /** in the line above a method or class, and Eclipse will generate the imperative remark block. All you want to do then is fill it in. Check the Animal class below :
public class Animal { private boolean canSwim; private boolean canWalk; private boolean canFly; private int numberLegs; private String habitat; /** * Creates a new Animal that walks, lives in the wild with 4 legs. */ public Animal() { this.canSwim = true; this.canWalk = false; this.canFly = false; this.numberlegs = 4; this.habitat = "Wild"; } /** * Creates a new Animal. * * @parameter s Whether or not the animal can swim. * @parameter w Whether or not the animal can walk. * @parameter f Whether or not the animal can fly. * @parameter n The animal's number of legs. * @parameter h The animal's habitat. */ public Animal(boolean s, boolean w, boolean f, int n, String h) { this.canSwim = s; this.canWalk = w; this.canFly = f; this.numberLegs = n; this.habitat = h; } /** * Eats a given food. * * @parameter food The food to eat. */ public void eat(String food) { System.out.println("Num num, just ate " + food); } /** * Sets whether or not the animal can swim. * * @parameter s Whether or not the animal can swim. */ public void setCanSwim(boolean S) { this.canSwim = s; } /** * Sets whether or not the animal can walk. * * @parameter w Whether or not the animal can walk. */ public void setCanWalk(boolean w) { this.canWalk = w; } /** * Sets whether or not the animal can fly. * * @parameter f Whether or not the animal can fly. */ public void setCanFly(boolean f) { this.canFly = f; }
/** * Sets the animal's number of legs. * * @parameter n The animal's number of legs. */ public void setNumberLegs(int n) { this.numberLegs = n; }
/** * Sets the animal's habitat. * * @parameter h The animal's habitat. */ public void setHabitat(String h) { this.habitat = h; } /** * Returns whether or not the animal can swim. * * @return True if the animal can swim, otherwise false. */ public boolean getCanSwim() { return this.canSwim; } /** * Returns whether or not the animal can walk. * * @return True if the animal can walk, otherwise false. */ public boolean getCanWalk() { return this.canWalk; } /** * Returns whether or not the animal can fly. * * @return True if the animal can fly, otherwise false. */ public boolean getCanFly() { return this.canFly; }
/** * Gets the number of legs the animal has. * * @return The animal's number of legs. */ public int getNumberLegs() { return this.numberLegs; }
/** * Gets the animal's habitat. * * @return The animal's habitat. */ public String getHabitat() { return this.habitat; } /** * Dipslay's the animal's information. */ public String toString() { return "This animal " + (this.canSwim ? "can swim, " : "can't swim, ") + (this.canWalk ? "can walk, " : "can't walk, ") + (this.canFly ? "can fly, " : "can't fly, ") + "has " + this.numberLegs + " leg(s), and lives in the " + this.habitat; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
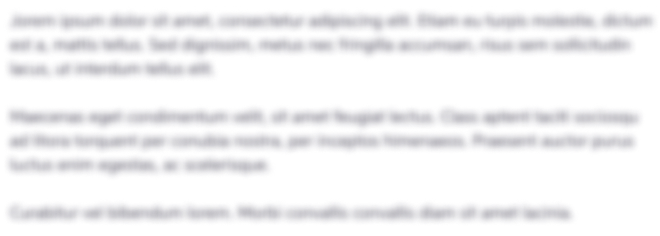
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started