Question
Java Modify the Sorting lab program to allow for a range of sort. The left index is inclusive and the right index is exclusive. 1.
Java
Modify the Sorting lab program to allow for a range of sort. The left index is inclusive and the right index is exclusive.
1.
public static void bubbleSort(int[] array, int left, int right) {
// your code here
}
2.
public static void insertionSort(int[] array, int left, int right) {
// your code here
}
3.
public static void selectionSort(int[] array, int left, int right) {
// your code here
}
Files in the same directory
import java.util.Scanner;
public class SortTester {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
System.out.println("Please enter a space separated list of numbers to sort or type QUIT to exit:");
String numbers = keyboard.nextLine();
while (!numbers.equalsIgnoreCase("quit")) {
int[] array = makeArray(numbers);
System.out.println("Sorted array using Bubble Sort algorithm:");
Sort.bubbleSort(array);
println(array);
array = makeArray(numbers);
System.out.println("Sorted array using Insertion Sort algorithm:");
Sort.insertionSort(array);
println(array);
array = makeArray(numbers);
System.out.println("Sorted array using Selection Sort algorithm:");
Sort.selectionSort(array);
println(array);
System.out.println("Please enter a space separated list of numbers to sort or type QUIT to exit:");
numbers = keyboard.nextLine();
}
keyboard.close();
}
public static int[] makeArray(String str) {
if (!str.trim().isEmpty()) {
String[] strings = str.trim().split("[ ]+");
int[] result = new int[strings.length];
for (int i = 0; i < result.length; i++)
result[i] = Integer.parseInt(strings[i]);
return result;
}
else {
return new int[0];
}
}
public static boolean equals(int[] array1, int[] array2) {
boolean equal = true;
if (array1.length != array2.length)
equal = false;
int i = 0;
while (equal && i < array1.length) {
equal = (array1[i] == array2[i]);
}
return equal;
}
public static void println(int[] array) {
for (int i = 0; i < array.length; i++) {
if (i == array.length - 1)
System.out.println(array[i]);
else
System.out.print(array[i] + " ");
}
}
}
Standard input 4 3 2 1ENTER quitENTER | Files in the same directory SortTester.java |
Please enter a space separated list of numbers to sort or type QUIT to exit: Sorting first half of the array using Bubble Sort algorithm: 3 4 2 1 Sorting second half of the array using Bubble Sort algorithm: 4 3 1 2 Sorting the array using Bubble Sort algorithm: 1 2 3 4 Sorting first half of the array using Insertion Sort algorithm: 3 4 2 1 Sorting second half of the array using Insertion Sort algorithm: 4 3 1 2 Sorting the array using Insertion Sort algorithm: 1 2 3 4 Sorting first half of the array using Selection Sort algorithm: 3 4 2 1 Sorting second half of the array using Selection Sort algorithm: 4 3 1 2 Sorting the array using Selection Sort algorithm: 1 2 3 4 Please enter a space separated list of numbers to sort or type QUIT to exit:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
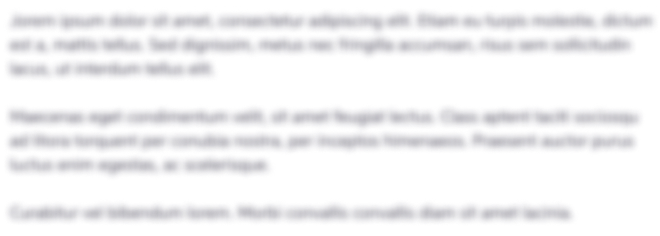
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started