Question
JAVA Must convert both from BASE2 to BASE10 and BASE10 to BASE2 and handle errors for both. Use popups to display error conditions. After the
JAVA Must convert both from BASE2 to BASE10 and BASE10 to BASE2 and handle errors for both. Use popups to display error conditions. After the user responds to the popup, clear the bad data and position the curser for new input. Define and throw your own NotInBinaryFormatException to handle the BASE2 -> BASE10. Use NumberFormatException to handle BASE10 -> BASE2. Be sure your error messages appear on the same monitor and over the converter application. Using the code below: import java.awt.event.*;
import java.awt.*;
import javax.swing.*;
publicclass BinaryDecimalConverterextends JFrameimplements ActionListener {
privatestaticfinallongserialVersionUID = 1L;// suppress warnings
// attributes for Decimal conversion
private JButtondecButton;
private JLabeldecLabel;
private JTextFielddecField;
// attributes for Binary conversion
private JButtonbinButton;
private JLabelbinLabel;
private JTextFieldbinField;
// button for clearing
private JButtonclearButton;
// class Constructor
BinaryDecimalConverter() {
// Used to specify GUI component layout
GridBagConstraintslayoutConst =null;
setLayout(new GridBagLayout());
// Set frame's title
setTitle("Binary-Decimal converter");
// Create objects to convert decimal numbers
// Decimal conversion
// Label
decLabel =new JLabel("Decimal number:");
// Create grid and add label
layoutConst =new GridBagConstraints();
// padding space around components
layoutConst.insets =new Insets(10, 10, 10, 10);
layoutConst.gridx = 0;// row 0
layoutConst.gridy = 0;// column 0
add(decLabel,layoutConst);
// Field
// Create field for data entry
decField =new JTextField();
decField.setEditable(true);
decField.setText("0");
decField.setColumns(15);// Initial width of 15 units
// Create grid and add field
layoutConst =new GridBagConstraints();
layoutConst.insets =new Insets(10, 10, 10, 10);
layoutConst.gridx = 1;
layoutConst.gridy = 0;
add(decField,layoutConst);
// Button
// Create button and add action listener
decButton =new JButton("Convert Decimal");
decButton.addActionListener(this);
decButton.setPreferredSize(new Dimension(200, 20));
// Create grid and add button
layoutConst =new GridBagConstraints();
layoutConst.insets =new Insets(10, 10, 10, 10);
layoutConst.gridx = 2;
layoutConst.gridy = 0;
add(decButton,layoutConst);
// End decimal conversion
// Binary conversion
// Label
binLabel =new JLabel("Binary number:");
layoutConst.gridx = 0;
layoutConst.gridy = 1;
add(binLabel,layoutConst);
// Field
binField =new JTextField();
binField.setEditable(true);
binField.setText("0");
binField.setColumns(15);// Initial width of 15 units
layoutConst.gridx = 1;
layoutConst.gridy = 1;
add(binField,layoutConst);
// Button
// Create button and add action listener
binButton =new JButton("Convert Binary");
binButton.addActionListener(this);
binButton.setPreferredSize(new Dimension(200, 20));
layoutConst.gridx = 2;
layoutConst.gridy = 1;
add(binButton,layoutConst);
// End binary conversion
// initializing clear button
clearButton =new JButton("Clear");
clearButton.setPreferredSize(new Dimension(200, 20));
clearButton.addActionListener(this);
layoutConst.gridx = 2;
layoutConst.gridy = 2;
add(clearButton,layoutConst);
}
/*
* Method is automatically called when an event occurs (e.g. mouse click on
* button
*/
@Override
publicvoid actionPerformed(ActionEventevent) {
// finding which button is clicked
if (event.getSource().equals(decButton)) {
// parsing integer value in decField as a binary string
Stringbin = Integer.toBinaryString(Integer.valueOf(decField
.getText()));
binField.setText(bin);
}elseif (event.getSource().equals(binButton)) {
// parsing String from binField from binary String to integer
intnumber = Integer.valueOf(binField.getText(), 2);
// displaying in decField
decField.setText(number +"");
}elseif (event.getSource().equals(clearButton)) {
//clearing text fields
binField.setText("");
decField.setText("");
}
}
publicstaticvoid main(String[]args) {
// Creating a BinaryDecimalConverter and making it visible
BinaryDecimalConvertermyFrame =new BinaryDecimalConverter();
myFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
myFrame.pack();
myFrame.setVisible(true);
}
}
Step by Step Solution
3.39 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
Add error handling for BASE2 to BASE10 conversion using a custom exception NotInBinaryFormatException Add error handling for BASE10 to BASE2 conversion using NumberFormatException Display error messag...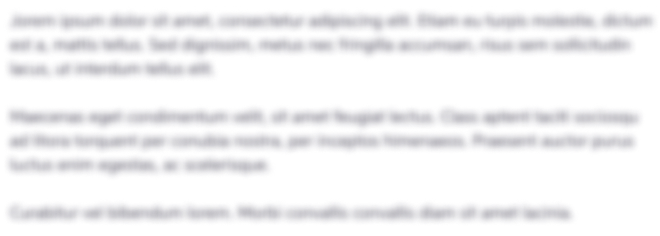
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started