Question
JAVA: Please help me change this DoubleLinkedList into a Circular Double LinkedList. import java.util.*; public class DoubleLinkedList { private static class Node { private E
JAVA:
Please help me change this DoubleLinkedList into a Circular Double LinkedList.
import java.util.*;
public class DoubleLinkedList
private static class Node
private E data; private Node
public Node(E data, Node
public Node(E data) { this(data, null,null); } }
private Node
private int size = 0;
private void addFirst(E item) { head = new Node
private E removeFirst() { Node
private E removeAfter(Node
public E get(int index) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException(Integer.toString(index)); } Node
public E set(int index, E newValue) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException(Integer.toString(index)); } Node
public void add(int index, E item) { if (index < 0 || index > size) { throw new IndexOutOfBoundsException(Integer.toString(index)); } if (index == 0) { addFirst(item); } else { Node
/** * Append the specified item to the end of the list * @param item The item to be appended * @returns true (as specified by the Collection interface) */ public boolean add(E item) { add(size, item); return true; }
// Insert solution to programming exercise 1, section 5, chapter 2 here
/** * Query the size of the list * @return The number of objects in the list */ int size() { return size; }
/** * Obtain a string representation of the list * @return A String representation of the list */ @Override public String toString() { StringBuilder sb = new StringBuilder("["); Node p = head; if (p != null) { while (p.next != null) { sb.append(p.data.toString()); sb.append(", "); p = p.next; } sb.append(p.data.toString()); } sb.append("]"); return sb.toString(); }
public static void main(String [] args) { DoubleLinkedList
//list.addAfter(list.getNode(2), "Peter"); //list.removeFirst(); list.removeAfter(list.getNode(3)); //System.out.println("The list is: " + list.size() + " long!"); //System.out.println(list); System.out.println(list); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
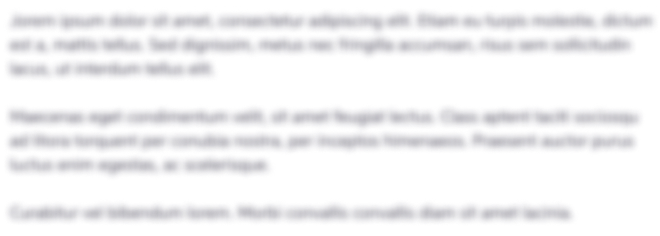
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started