Question
Java Priority Queues. Please help me :(. I don't know why the index keeps starts at index 1 and it adds 1 to the heap.
Java Priority Queues.
Please help me :(. I don't know why the index keeps starts at index 1 and it adds 1 to the heap.
Add a toString() method to class Task, so you can display the instance variables of each task object. This method should display both instance variables in a proper format.
The PriorityQueue task contains a heap, which is essentially an array. The problem in this implementation is that all arrays start at index 0. This implementation incorrectly adds 1 more to the heap and starts at index 1 instead of index 0. Fix this error so the heap starts correctly at index 0.
Add a show() method to the PriorityQueue which will display each of the active tasks on the heap.
Add an option 7 to the PriorityQueueTest, which will call the show() method. Again, this method should only display active tasks on the heap, showing them in proper priority order.
package priorityqueuetest; /** ** Java Program to implement Priority Queue **/ import java.util.Scanner; /** class Task **/ class Task { String job; int priority; /** Constructor **/ public Task(String job, int priority) { this.job = job; this.priority = priority; } } /** Class PriorityQueue **/ class PriorityQueue { private Task[] heap; private int heapSize, capacity; /** Constructor **/ public PriorityQueue( int capacity ) { this.capacity = capacity+1; heap = new Task[this.capacity]; heapSize = 0; } /** function to clear **/ public void clear() { heap = new Task[capacity]; heapSize = 0; } /** function to check if empty **/ public boolean isEmpty() { return heapSize == 0; } /** function to check if full **/ public boolean isFull() { return heapSize == capacity - 1; } /** function to get Size **/ public int size() { return heapSize; } /** function to insert task **/ public void insert( String job, int priority ) { Task newJob = new Task( job, priority ); heap[++heapSize] = newJob; int pos = heapSize; while (( pos != 1 ) && ( newJob.priority > heap[pos/2].priority )) { heap[pos] = heap[pos/2]; pos /=2; } heap[pos] = newJob; } /** function to remove task **/ public Task remove() { int parent, child; Task item, temp; if (isEmpty() ) { System.out.println("Heap is empty"); return null; } item = heap[1]; temp = heap[heapSize--]; parent = 1; child = 2; while ( child <= heapSize ) { if (( child < heapSize ) && ( heap[child].priority < heap[child+1].priority )) { child++; } if ( temp.priority >= heap[child].priority ) { break; } heap[parent] = heap[child]; parent = child; child *= 2; } heap[parent] = temp; return item; } } /** Class PriorityQueueTest **/ public class PriorityQueueTest { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Priority Queue Test "); System.out.println("Enter Desired Size of Priority Queue: "); PriorityQueue pq = new PriorityQueue(scan.nextInt() ); int choice; /* Perform Priority Queue operations */ do { System.out.println( "Priority Queue Operations" ); System.out.println( "1. insert" ); System.out.println( "2. remove" ); System.out.println( "3. check empty" ); System.out.println( "4. check full" ); System.out.println( "5. clear" ); System.out.println( "6. size" ); System.out.println( "8. quit" ); System.out.print( "Choice: " ); choice = scan.nextInt(); switch ( choice ) { case 1 : System.out.println("Enter job name and priority"); pq.insert(scan.next(), scan.nextInt() ); break; case 2 : System.out.println(" Job removed "+ pq.remove()); break; case 3 : System.out.println(" Empty Status : "+ pq.isEmpty() ); break; case 4 : System.out.println(" Full Status : "+ pq.isFull() ); break; case 5 : System.out.println(" Priority Queue Cleared"); pq.clear(); break; case 6 : System.out.println(" Size = "+ pq.size() ); break; case 8: System.out.println( "Goodbye" ); break; default : System.out.println("Wrong Entry "); break; } } while ( choice != 8 ); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
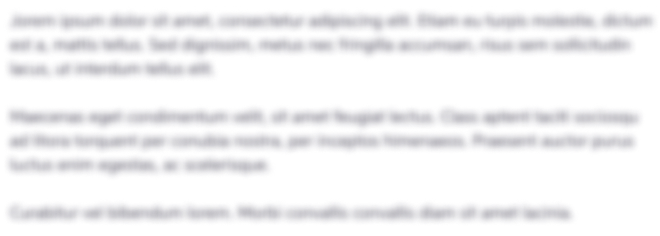
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started