Question
Java Program None of the classes given to you are complete. Your assignment is to complete the four classes and create a fith class so
Java Program
None of the classes given to you are complete. Your assignment is to complete the four classes and create a fith class so that an instance of MountainScene appears as a picture containing four different moutain elements. Here is a list of the requirements of the assignment :
- Your picture should have 4 types of elements. 3 of them must be a cable car on a cable running across the picture, a snow man with a hat and arms, and a tree with ornaments. The fourth one is up to you.
- Create a class for each type of element. The class should at least contain a constructor and a private draw method. Don't change the signature of the constructor. Keep it as public ClassName(int x, int y, double scale, GWindow window) to mean create an object of type ClassName at location (x,y) in the GWindow window with size given by the default size times scale (e.g. you can set the radius of the snow man head by default to 20. Then a scale of 1.5 would draw a snow man with a head of radius 20*1.5 = 30). Don't do the drawing within the constructor. Instead call the private method draw. We will see the advantage of using a draw method in our next homework assignment. Read through the Tree class. It contains code to give you a better idea of what to do.
- Complete the class MountainScene to create a picture with all of the elements in a graphics window. In the picture, each type of element should appear several times at different locations with different sizes (except for the cable car that you can only draw once).
- The graphics elements should show some complexity. A single oval is not a good drawing for a snow man.
- The program should be correctly documented (use the same format as in the sample (javadoc comments /** and */)).
To create the graphics elements, draw them first on a piece of graph paper. It will make it easy for you to locate each point of your drawing with respect to some reference point (x,y).
If you want to create your own colors, use the Color constructor. It reads Color(r,g,b) where r,g and b are integers between 0 and 255. The color is a mixture of red, green and blue as specified by r, g and b. For example
Color myColor = new Color(100,150,210); // a blueish color
==========================================================================
import uwcse.graphics.*; // access the graphics utilities in the uw library import java.awt.Color; // access the Color class /** *Create a cable car in a graphics window
* @author your name here */ public class CableCar { // Your instance fields go here /** * Create a cable car at location (x,y) in the GWindow window. * @param x the x coordinate of the center of the cable car * @param y the y coordinate of the center of the cable car * @scale the factor that multiplies all default dimensions for this cable car * (e.g. if the default size is 80, the size of this cable car is * scale * 80) * @window the graphics window this cable car belongs to */ public CableCar(int x, int y, double scale, GWindow window) { // initialize the instance fields // The details of the drawing are in a private method this.draw(); } /** Draw a cable car at location (x,y) */ private void draw() { } }
========================================================================
import uwcse.graphics.*; // access the graphics utilities in the uw library import java.awt.Color; // access the Color class /** ** A MountainScene displays snow men, trees (with ornaments), a cable car and a * fourth element of your choice in a graphics window *
* * @author Your name here */ public class MountainScene { /** The graphics window that displays the picture */ private GWindow window; /** * Create an image of a mountain scene */ public MountainScene() { // The graphics window // The window is by default 500 wide and 400 high this.window = new GWindow("Mountain scene"); this.window.setExitOnClose(); // so that a click on the close box of the // window terminates the application // Background (cyan here) Rectangle bgnd = new Rectangle(0, 0, window.getWindowWidth(), window .getWindowHeight(), Color.cyan, true); this.window.add(bgnd); // Create the scene elements // e.g. a tree in the lower left area 1.5 times the normal size new Tree(100, 300, 1.5, this.window); } /** * Entry point of the program */ public static void main(String[] args) { new MountainScene(); } }
==========================================================================
import uwcse.graphics.*; // access the graphics utilities in the uw library import java.awt.Color; // access the Color class /** *Create a snow man in a graphics window
* @author your name here */ public class SnowMan { // Your instance fields go here /** * Create a snow man in at location (x,y) in the GWindow window. * @param x the x coordinate of the center of the head of the snow man * @param y the y coordinate of the center of the head of the snow man * @scale the factor that multiplies all default dimensions for this snow man * (e.g. if the default head radius is 20, the head radius of this snow man is * scale * 20) * @window the graphics window this snow man belongs to */ public SnowMan(int x, int y, double scale, GWindow window) { // initialize the instance fields // Put the details of the drawing in a private method this.draw(); } /** Draw in the graphics window a snow man at location (x,y) */ private void draw() { } }
======================================================================
import uwcse.graphics.*; // access the graphics utilities in the uw library import java.awt.Color; // access the Color class /** *Create a tree with ornaments in a graphics window
* @author your name here */ public class Tree { // Instance fields // The graphics window this tree belongs to private GWindow window; // The location of this tree // (precisely (as done in the draw method), (x,y) is // the upper left corner of the tree trunk) private int x; private int y; // The scale used to draw this tree private double scale; /** * Create a tree * @param x the x coordinate of the tree location (upper left corner of the tree trunk) * @param y the y coordinate of the tree location * @param window the graphics window this Tree belongs to * @param scale the scale of the drawing (all default dimensions are multiplied * by scale) */ public Tree(int x, int y, double scale, GWindow window) { // Initialize the instance fields (the use of this is required // since the instance fields have the same name as the // parameters of the constructor) this.window = window; this.scale = scale; this.x = x; this.y = y; // the details of the drawing are in written in the private method draw() this.draw(); } /** * draw a pine tree */ private void draw() { // trunk of the tree: a brown rectangle // (int) converts to an int 20*this scale (etc...), which is a double // For instance, (int)23.8 is 23 // This is necessary since the Rectangle constructor takes integers Rectangle trunk = new Rectangle(this.x,this.y,(int)(20*this.scale),(int)(60*this.scale), Color.black,true); // Foliage (improve the drawing!) // a green triangle Triangle foliage = new Triangle(this.x-(int)(30*this.scale),this.y+(int)(30*this.scale), this.x+(int)(10*this.scale),this.y-(int)(10*this.scale), this.x+(int)(50*this.scale),this.y+(int)(30*this.scale), Color.green,true); this.window.add(trunk); this.window.add(foliage); // Improve the drawing of the foliage and add the ornaments... } }
============================================================================
Please I'll give thumbs up. thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
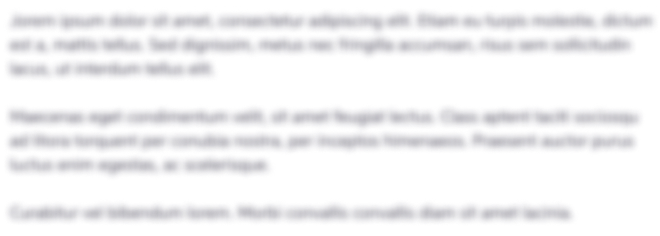
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started