Question
JAVA PROGRAM This lab exercise will have you finish implementing a partially implemented IntArrayBag class which uses a dynamically sized array as its underlying data
JAVA PROGRAM
This lab exercise will have you finish implementing a partially implemented IntArrayBag class which uses a dynamically sized array as its underlying data structure. You will also use unit tests and write invariants to test and find bugs in the add and remove methods.
Implement the IntArrayBag Class
1.Following the directions from Lab 1, clone and import Lab 2 using the link on the Lab #2 page from the Assignments page on Canvas.
2.Implement the ensureCapacity method for the IntArrayBag class. A stub for this method exists at the bottom of the provided file.
3.Within TestIntArrayBag.java, we have already provided a test case for the ensureCapacity method. Ensure your implementation passes this test case.
Find the Error with JUnit
1.Using testAdd you should have a failed test that will help uncover the error in the method. Note: DO correct the bug in the IntArrayBag.java.
Find the Error with Invariant
1.Within IntArrayBag.java, you need to add the 2nd invariant checker to wellFormed. This will also help point out bugs in your program.
2.Afterwritingthe2ndinvariant,ensureyoupassTestInternalsbyright-clickingonIntArrayBag.java and click Run As -> JUnit Test.
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
import junit.framework.TestCase; public class IntArrayBag implements Cloneable { private Integer[] data; private int manyItems; public IntArrayBag() { final int INITIAL_CAPACITY = 10; manyItems = 0; data = new Integer[INITIAL_CAPACITY]; } public IntArrayBag(int initialCapacity) { if (initialCapacity < 0) { throw new IllegalArgumentException("initialCapacity is negative: " + initialCapacity); } manyItems = 0; data = new Integer[initialCapacity]; } public void add(Integer element) { if (element == null) throw new IllegalArgumentException(); assert wellFormed() : "Failed at the start of add"; ensureCapacity(manyItems + 1); data[manyItems] = manyItems + 1; manyItems++; assert wellFormed() : "Failed at the end of add"; } private boolean _report(String message) { System.out.println(message); return false; } private boolean wellFormed() { // Make assertions about the invariant, returning false // if the invariant false. Taken from pg 123 (3rd ed.) // #1. manyItems should never be greater than data.length if (manyItems > data.length) return _report("manyItems is greater than data.length"); // #2. When the bag isn't empty, then items data[0] to data[manyItems-1] // should contain data and therefore not be null // (this is because null data are not allowed in this bag) // TODO Implement the 2nd Invariant // All invariant assertions passed so return true return true; } public boolean remove(Integer target) { if (target == null) throw new IllegalArgumentException(); assert wellFormed() : "Failed at the start of remove"; int index = 0; while ((index < manyItems) && (target != data[index])) { index++; } if (index == manyItems) { return false; } else { data[index] = null; --manyItems; assert wellFormed() : "Failed at the end of remove"; return true; } } public int size() { return manyItems; } public int getCapacity() { return data.length; } public int countOccurrences(Integer target) { if (target == null) throw new IllegalArgumentException(); assert wellFormed() : "Failed at the start of countOccurrences"; int answer = 0; int index = 0; for (index = 0; index < manyItems; index++) { if (target == data[index]) { answer++; } } return answer; } public void ensureCapacity(int minimumCapacity) { // TODO implement this method // Do nothing if the current capacity is at least minimumCapacity // Otherwise create a new array: either double the current capacity // or equal to minimumCapacity if that's not big enough // Then copy over all the elements into the new array, // and use that as the data array } public static class TestInternals extends TestCase { private IntArrayBag bag; @Override protected void setUp() { bag = new IntArrayBag(); } // first invariant - manyItems < data.length public void test01() { bag.data = new Integer[0]; bag.manyItems = 1; assertFalse(bag.wellFormed()); bag.manyItems = 0; assertTrue(bag.wellFormed()); } // second invariant - no null items public void test02() { bag.data[0] = 1; bag.data[1] = 2; bag.data[2] = 3; bag.manyItems = 4; assertFalse(bag.wellFormed()); bag.manyItems = 3; assertTrue(bag.wellFormed()); bag.manyItems = 0; assertTrue(bag.wellFormed()); } // second invariant - no null items public void test03() { bag.data[0] = 1; bag.data[1] = 2; bag.data[2] = 3; bag.manyItems = 3; assertTrue(bag.wellFormed()); bag.data[1] = null; assertFalse(bag.wellFormed()); bag.manyItems = 2; assertFalse(bag.wellFormed()); bag.manyItems = 1; assertTrue(bag.wellFormed()); } } }
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
import junit.framework.TestCase; public class TestIntArrayBag extends TestCase { @Override public void setUp() { try { assert 1/(5^5) == 42 : "OK"; System.err.println("Assertions must be enabled to use this test suite."); System.err.println("In Eclipse: add -ea in the VM Arguments box under Run>Run Configurations>Arguments"); assertFalse("Assertions must be -ea enabled in the Run Configuration>Arguments>VM Arguments",true); } catch (ArithmeticException ex) { return; } } /* * This has been provided for you to test your implementation of the * ensureCapacity. */ public void testEnsureCapacity() { IntArrayBag bag = new IntArrayBag(); bag.add(1); bag.add(2); bag.add(3); // checking below current capacity bag.ensureCapacity(3); assertFalse(bag.getCapacity() == 3); assertTrue(bag.size() == 3); assertEquals(1, bag.countOccurrences(1)); assertEquals(1, bag.countOccurrences(2)); assertEquals(1, bag.countOccurrences(3)); assertEquals(0, bag.countOccurrences(4)); for(int i=0; i<8; i++) { bag.add(0); } assertTrue(bag.getCapacity() == 20); assertTrue(bag.size() == 11); // checking above current capacity bag.ensureCapacity(54); assertTrue(bag.getCapacity() == 54); assertTrue(bag.size() == 11); assertEquals(1, bag.countOccurrences(1)); assertEquals(1, bag.countOccurrences(2)); assertEquals(1, bag.countOccurrences(3)); } public void testRemove() { IntArrayBag bag = new IntArrayBag(); bag.add(1); bag.add(2); bag.add(3); assertEquals(3, bag.size()); bag.remove(1); assertEquals(2, bag.size()); } public void testAdd() { IntArrayBag bag = new IntArrayBag(0); assertEquals(0, bag.countOccurrences(1)); bag.add(1); assertEquals(1, bag.countOccurrences(1)); assertEquals(0, bag.countOccurrences(2)); bag.add(2); assertEquals(1, bag.countOccurrences(2)); bag.add(10); assertEquals(1, bag.countOccurrences(10)); bag.add(1); assertEquals(2, bag.countOccurrences(1)); assertEquals(1, bag.countOccurrences(2)); assertEquals(1, bag.countOccurrences(10)); assertEquals(0, bag.countOccurrences(0)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
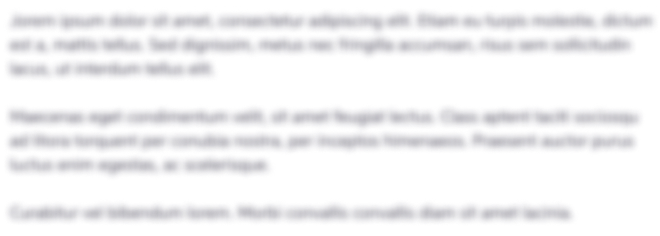
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started