Question
Java Programming. Need to build off existing program which will be located at the bottom. Only the Main and Chatbot class need to be added
Java Programming. Need to build off existing program which will be located at the bottom. Only the Main and Chatbot class need to be added too
You will make a slightly more interactive ChatBot this time. Your program will take input from the user and give it to yourChatBot. From here, it will look through your message, find any keywords that interest it, and print out a response based on the keyword it finds.
Instructions
As you will be reusing code from project three, you should be starting this project with the following three classes created and partially implemented:
- Main.java (class name Main)
- ChatBot.java (class name ChatBot)
- Memory.java (class name Memory)
In the event you need code to start this project with, you can use the following code as a starting point, or at least a point of reference: Project3_ChatBot_Answer.zip
In quick review, the Memory class contains variables that represent important information that a ChatBot would need to know. The ChatBot class contains logic for how a ChatBot can respond to a user (with various 'reply' functions). The ChatBot class has a memory object as an instance variable. Main was originally used to create a single ChatBot and perform a single interaction with it.
For this project, you will update the code to accept a whole sentence from the user in your Main class. Once you read in the sentence from the user, your ChatBot will search the sentence for any one of the following key words:
- hello
- name
- favorite
- quirk
- height
- weight
If you find one of these key words, you'll have the chatbot reply with an appropriate response. The program should respond to one word it finds in the sentence--order doesn't matter, but it should still only respond to one.
In example, if you say "Hello there chatbot!" ...when the chatbot looks through the sentence, it should see the word "hello" and respond to it. Specifically, it will respond with the replyHello() function.
If you instead say, "My name is Jared." then the ChatBot should respond with its own name. Specifically, it will respond with the replyName() function.
If you say two keywords in one sentance, the chatbot is allowed to respond to the whichever word you want--you can choose the precedence. So, if you say, "My name is Tim. Do you have favorite day of the week?" ...it doesn't amtter whether the ChatBot responds to the word "name" and not the word "favorite" or vice versa. However, it must respond to only one of those keywords.
This checking of the users sentence will occur in the interact() function in ChatBot.java.
Your program should loop in main, each time asking the user for a sentence. If the user types "exit" then the loop should end.
You will make the following major changes to Main.java:
- Keep the chatbot you created, but delete the call to your chatbot's interact() function.
- In the main function, after the chatbot you originally created, Create a scanner for input.
- Following that, add the following into the main function:
- A loop that loops until the user enters the exact string "exit"
- Add a call to your chatbot's interact function in the loop. Pass in the user's String sentence as a parameter.
You will make the following major changes to ChatBot.java:
- Set the chatbot's name, favorite hobby, favorite dotw, quirk, height and weight to something meaningful in the ChatBot() constructor. I allowed filler values for these before, but I expect actual values to be given now.
- Add replyHeight() and replyWeight() functions to ChatBot.java. Add a System.out.print call to each, and have the chatbot say something for each. Be sure to use the get functions from the memory object to grab out the height and weight.
- Modify interact(String userMessage) function to:
- look through the user's message to the chatbot to find a keyword. You'll want to review our slides on Strings from Week 7 for how to do this.
- Store the keyword you found in a String variable. From there you can use ifs or a switch block to check if the value of the string equals any of the keywords mentioned earlier. (If you think it would be easier to not store the variable, that's fine as well.)
- If the string you stored equals a keyword, print out that key words's reply function.
- If the string you stored does not contain a keyword, just have the ChatBot respond with the replyAskAboutUser() function.
Goals
- Get experience using and searching through Strings.
- Get experience with loops
- Get experience with Modifying an existing custom class.
Sample Runs
Sample Program Run (user input is underlined)
Say something: What's your name?
My name is Tim!
Say something: Do you have a favorite hobby?
I really like Reading and Mondays!
Say something: Do you work?
What about you? Care to tell me about yourself?
Say something: ...
What about you? Care to tell me about yourself?
Code that needs to be built upon
public class Main { /* * main functions which calls all classes to run program */
public static void main(String[] args) { ChatBot c=new ChatBot(); c.interact(""); }
} public class ChatBot {
private Memory mem; public ChatBot() { /* * Set and call from classes for output */ mem=new Memory(); mem.setName("Andrew"); mem.setFavoriteHobby("building things"); mem.setFavoriteDOTW("Friyay"); mem.setQuirk(" overjoyous"); mem.setHeight(5.5); mem.setWeight(95.65); } /* * interact with user */ public void interact(String userMessage) { replyHello(); replyName(); replyFavoriteThings(); replyQuirk(); replyAskAboutUser(); } /* * shows all output */ private void replyHello() { System.out.println("Hello there!"); } private void replyName() { System.out.println("My name is "+mem.getName()+"!"); } private void replyFavoriteThings() { System.out.println("I absolutely love "+mem.getFavoriteDOTW()+"s and "+mem.getFavoriteHobby()+"!"); } private void replyQuirk() { System.out.println("People say I'm "+mem.getQuirk()+"."); } private void replyAskAboutUser() { System.out.println("What about you? Can you tell me about yourself?"); } } public class Memory { /* * intilize variables */ private String name, favoriteHobby, favoriteDOTW, quirk; private double height, weight; /* * allows for name */ public void setName(String name) { this.name=name; } public void setFavoriteHobby(String favoriteHobby) { /* * allows for fav hobby */ this.favoriteHobby=favoriteHobby; } /* * allows for favorite day of week */ public void setFavoriteDOTW(String favoriteDOTW) { this.favoriteDOTW=favoriteDOTW; } /* * allows for quirk */ public void setQuirk(String quirk) { this.quirk=quirk; } public void setHeight(double height) { /* * allows for height and weight to be set */ this.height=height; } public void setWeight(double weight) { this.weight=weight; } /* * get variables, calls each and pulls variables from each */ public String getName() { return this.name; } public String getFavoriteHobby() { return this.favoriteHobby; } public String getFavoriteDOTW() { return this.favoriteDOTW; } public String getQuirk() { return this.quirk; } public double getHeight() { return this.height; } public double getWeight() { return this.weight; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
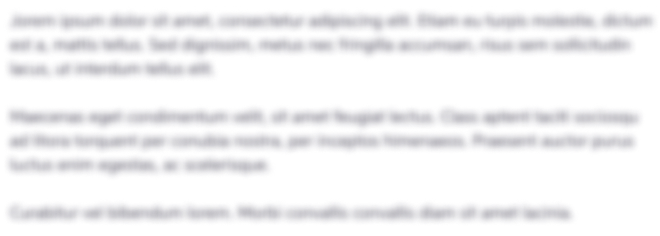
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started