Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java Programming Question. Add a new method to ArrayTools called copy . Make this method accept two String arrays. Copy the content from the first
Java Programming Question.
Add a new method to ArrayTools called copy. Make this method accept two String arrays. Copy the content from the first array into the second array. If the second array isn't big enough, show an error message.
Given:
import java.util.Scanner; public class Demo2 { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); System.out.println("How many strings?"); int size = keyboard.nextInt(); keyboard.nextLine(); String[] arr = new String[size]; for (int subscript = 0; subscript < size; subscript++) { System.out.println("Enter a value:"); arr[subscript] = keyboard.nextLine(); } String[] destination = new String[size]; System.out.println("copy/print test 1"); ArrayTools.copy(arr, destination); ArrayTools.printAll(destination); destination = new String[size + 1]; System.out.println("copy/print test 1"); ArrayTools.copy(arr, destination); ArrayTools.printAll(destination); destination = new String[size - 1]; System.out.println("copy/print test 1"); ArrayTools.copy(arr, destination); ArrayTools.printAll(destination); destination = new String[5]; System.out.println("copy/print test 1"); ArrayTools.copy(arr, destination); ArrayTools.printAll(destination); destination = new String[size * size]; System.out.println("copy/print test 1"); ArrayTools.copy(arr, destination); ArrayTools.printAll(destination); } }
public class ArrayTools { public static int printAll(String[] input) { int x = input.length; for(int i = 0; i < x; ++i) { System.out.println("[" + i + "]: " + input[i]); } return x; } }
Required output:
How many strings? Enter a value: Enter a value: Enter a value: Enter a value: Enter a value: Enter a value: Enter a value: Enter a value: Enter a value: Enter a value: copy/print test 1 [0]: a [1]: b [2]: c [3]: d [4]: e [5]: f [6]: g [7]: h [8]: i [9]: j copy/print test 1 [0]: a [1]: b [2]: c [3]: d [4]: e [5]: f [6]: g [7]: h [8]: i [9]: j [10]: null copy/print test 1 Error: Second array isn't big enough. First array size: 10 Second array size: 9 [0]: null [1]: null [2]: null [3]: null [4]: null [5]: null [6]: null [7]: null [8]: null copy/print test 1 Error: Second array isn't big enough. First array size: 10 Second array size: 5 [0]: null [1]: null [2]: null [3]: null [4]: null copy/print test 1 [0]: a [1]: b [2]: c [3]: d [4]: e [5]: f [6]: g [7]: h [8]: i [9]: j [10]: null [11]: null [12]: null [13]: null [14]: null [15]: null [16]: null [17]: null [18]: null [19]: null [20]: null [21]: null [22]: null [23]: null [24]: null [25]: null [26]: null [27]: null [28]: null [29]: null [30]: null [31]: null [32]: null [33]: null [34]: null [35]: null [36]: null [37]: null [38]: null [39]: null [40]: null [41]: null [42]: null [43]: null [44]: null [45]: null [46]: null [47]: null [48]: null [49]: null [50]: null [51]: null [52]: null [53]: null [54]: null [55]: null [56]: null [57]: null [58]: null [59]: null [60]: null [61]: null [62]: null [63]: null [64]: null [65]: null [66]: null [67]: null [68]: null [69]: null [70]: null [71]: null [72]: null [73]: null [74]: null [75]: null [76]: null [77]: null [78]: null [79]: null [80]: null [81]: null [82]: null [83]: null [84]: null [85]: null [86]: null [87]: null [88]: null [89]: null [90]: null [91]: null [92]: null [93]: null [94]: null [95]: null [96]: null [97]: null [98]: null [99]: null
Step by Step Solution
There are 3 Steps involved in it
Step: 1
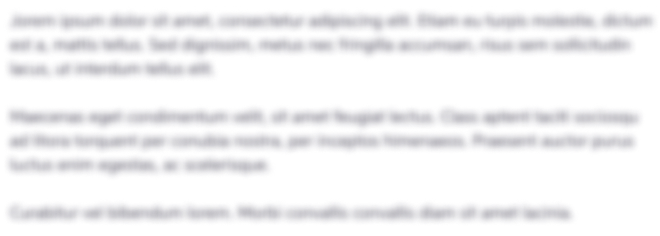
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started