Question
***JAVA PROGRAMMING*** Write a Menu Driven program * for the student to enter information and enroll fro m the list of available courses 1. Ask
***JAVA PROGRAMMING*** Write a Menu Driven program * for the student to enter information and enroll fro m the list of available courses 1. Ask student for first name, last name, id, address and instantiate a student object. 2. Create a menu from the sections returned from the DataStore class (an array of section objects) 3. Prompt the student to enroll in sections available from the menu 4. Handle the custom exceptions and let the student know if they try to enroll in a duplicate section or if they have enrolled in too many 5. Provide a "Quit" option in your menu 6. When the user chooses "Quit", automatically print their information and schedule.
---ADDRESS CLASS---
public class Address extends Object { //Attributes private String street; private String city; private String state; private String zip; //Default constructor public Address(){ } //Designated constructor public Address(String street, String city, String state, String zip) { this.street = street; this.city = city; this.state = state; this.zip = zip; } //Constructor public Address(String state, String zip){ this("", "",state, zip); } //Getter for street public String getStreet() { return street; } //Setter for street public void setStreet(String street) { this.street = street; } //Getter for city public String getCity() { return city; } //Setter for city public void setCity(String city) { this.city = city; } //Getter for state public String getState() { return state; } //Setter for state public void setState(String state) { this.state = state; } //Getter for zip public String getZip() { return zip; } //Setter for zip public void setZip(String zip) { this.zip = zip; } //toString method @Override public String toString() { return "Address: " + street + " " + city + ", " + state + " " + zip; } }
---PERSON CLASS---
public class Person { //Attributes private String firstName; private String lastName; private Address address; //Default constructor public Person(){ this("", "", new Address()); } //Designated constructor public Person(String firstName, String lastName, Address address) { this.firstName = firstName; this.lastName = lastName; this.address = address; } //Constructor public Person(String firstName, String lastName){ this(firstName, lastName, new Address()); } //Getter and Setter methods public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Address getAddress() { return address; } public void setAddress(Address address) { this.address = address; } //toString Method @Override public String toString() { return firstName + " " + lastName + " "; } }
---STUDENT CLASS---
import java.util.Arrays; public class Student extends Person{ //Attributes String id; CourseSection [] sections = new CourseSection [5]; private int numberEnrolled = 0; //Default constructor public Student(){ } //Designated constructor public Student(String id, String firstName, String lastName, Address address){ super(firstName, lastName, address); this.id = id; for (int i = 0; i < sections.length; i++){ sections [i] = new CourseSection(); } } //Getter and setter for ID public String getId() { return id; } public void setId(String id) { this.id = id; } //Method to add a course public void addCourse(CourseSection section)throws MaximumCoursesExceeded, DuplicateCourseException{ try{ for (int i = 0; i < numberEnrolled; i++){ if (sections[i].getSubject().equals(section.getSubject()) && sections[i].getNumber().equals(section.getNumber())) { throw new DuplicateCourseException(); } } sections[numberEnrolled] = section; numberEnrolled++; } catch (ArrayIndexOutOfBoundsException e){ throw new MaximumCoursesExceeded(); } } //toString method @Override public String toString() { return "Student:" + super.toString() + " " + "Student ID: " + id + " "; } } ---COURSE CLASS---
public class Course { //Attributes String subject; String number; String name; //Default constructor public Course(){ } //Designated constructor public Course(String subject, String number, String name) { this.subject = subject; this.number = number; this.name = name; } //Constructor public Course(String subject, String name){ this(subject, "", name); } //Getter and setter methods public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } public String getNumber() { return number; } public void setNumber(String number) { this.number = number; } public String getName() { return name; } public void setName(String name) { this.name = name; } //toString method @Override public String toString() { return " " + subject + " " + number + " " + name + " "; } }
---COURSESECTION CLASS---
public class CourseSection extends Course { //Attributes String id; String days; String startTime; String building; String room; //Default Constructor public CourseSection() { } //Constructor with all attributes public CourseSection(String subject, String number, String name, String id, String days, String startTime, String building, String room){ super(subject, number, name); this.id = id; this.days = days; this.startTime = startTime; this.building = building; this.room = room; } public CourseSection(Course c, String id, String days, String startTime, String building, String room){ super(c.getSubject(), c.getNumber(), c.getName()); this.id = id; this.days = days; this.startTime = startTime; this.building = building; this.room = room; } // Getters and Setters public String getId() { return id; } public void setId(String id) { this.id = id; } public String getDays() { return days; } public void setDays(String days) { this.days = days; } public String getStartTime() { return startTime; } public void setStartTime(String startTime) { this.startTime = startTime; } public String getBuilding() { return building; } public void setBuilding(String building) { this.building = building; } public String getRoom() { return room; } public void setRoom(String room) { this.room = room; } @Override public String toString() { return " CourseSection: " + super.toString() + id + " " + days + " " + startTime + " " + building + " " + room + " " + " "; } }
---DataStore Class---
import java.io.File; import java.io.FileNotFoundException; import java.nio.file.Paths; import java.util.Scanner; public class DataStore { //Static attribute private static DataStore singleton = null; //Private default constructor private DataStore(){ } //Private section array attribute private static CourseSection[] sections = new CourseSection[15]; private static File sectionFile = Paths.get(".", "resources", "Sections.txt").normalize().toFile(); public static DataStore getInstance() { if (singleton == null) { singleton = new DataStore(); Scanner fs; //Try/catch try { fs = new Scanner(sectionFile); for (int i = 0; i < sections.length; i++) { sections[i] = new CourseSection(fs.next(), fs.next(), fs.next(), fs.next(), fs.next(), fs.next(), fs.next(), fs.next()); } } catch (FileNotFoundException e) { System.out.print(e.getMessage()); } } return singleton; } //Getter public static CourseSection[] getSections() { return sections; } }
***HERE IS THE SECTIONS.TXT FILE***
CSC 151 Beginning_Java FJT01 MW 8AM AT 226 CSC 151 Beginning_Java FJT02 MW 10AM AT 226 CSC 151 Beginning_Java FJT03 MW 12PM AT 226 CSC 251 Advanced_Java FJT01 MW 8AM AT 226 CSC 251 Advanced_Java FJT02 MW 10AM AT 226 CSC 251 Advanced_Java FJT03 MW 12PM AT 226 WEB 151 Beginning_Mobile FJT01 TTH 8AM AT 129 WEB 151 Beginning_Mobile FJT02 TTH 10AM AT 129 WEB 115 Beginning_Web FJT01 TTH 12PM AT 219 WEB 115 Beginning_Web FJT02 TTH 2PM AT 219 CSC 139 Beginning_Visual_Basic FJT01 F 10AM AT 226 CSC 139 Beginning_Visual_Basic FJT02 MW 2PM AT 226 WEB 251 Advanced_Mobile FJT01 TTH 2PM AT 129 WEB 251 Advanced_Mobile FJT02 MW 6PM AT 129 ENG 111 English_Composition FJT01 M 6PM AT 254
Step by Step Solution
There are 3 Steps involved in it
Step: 1
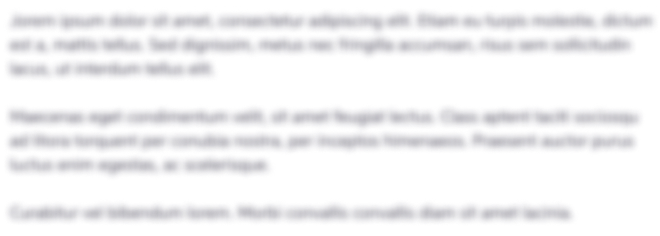
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started