Question
Java Question + Given Code (Below): package edu.wit.cs.comp1000; public class LA5a { /** * Error to output if either denominator is zero */ static final
Java Question + Given Code (Below):
package edu.wit.cs.comp1000;
public class LA5a {
/**
* Error to output if either denominator is zero
*/
static final String E_DEN_ZERO = "Denominator cannot be zero.";
/**
* Error to output if dividing by zero
*/
static final String E_DIV_ZERO = "Cannot divide by zero.";
/**
* Error to output if the operation is invalid
*/
static final String E_OP_INVALID = "Invalid operation.";
/**
* Returns the greatest common divisor (gcd) of two integers
*
* @param num1 integer 1
* @param num2 integer 2
* @return gcd of integers 1 and 2
*/
public static int gcd(int num1, int num2) {
int t;
while (num2 != 0) {
t = num2;
num2 = num1 % num2;
num1 = t;
}
return num1;
}
/**
* Returns the simplified form of a fraction
*
* @param f fraction (numerator=[0], denominator=[1])
* @return simplified fraction (numerator=[0], denominator=[1])
*/
public static int[] simplifyFraction(int[] f) {
final int gcd = gcd(f[0], f[1]);
int[] result = {f[0]/gcd, f[1]/gcd};
if ((result[0]
result[0] = -result[0];
result[1] = -result[1];
}
return result;
}
/**
* Adds two fractions
*
* @param f1 first fraction (numerator=[0], denominator=[1])
* @param f2 second fraction (numerator=[0], denominator=[1])
* @return result of adding parameters (numerator=[0], denominator=[1])
*/
public static int[] addFractions(int[] f1, int[] f2) {
int[] result = new int[2];
result[0] = (f1[0] * f2[1]) + (f2[0] * f1[1]);
result[1] = f1[1] * f2[1];
return simplifyFraction(result);
}
/**
* Subtracts two fractions
*
* @param f1 first fraction (numerator=[0], denominator=[1])
* @param f2 second fraction (numerator=[0], denominator=[1])
* @return result of subtracting parameter f2 from f1 (numerator=[0], denominator=[1])
*/
public static int[] subtractFractions(int[] f1, int[] f2) {
return new int[2]; // TODO: replace with your code
}
/**
* Multiplies two fractions
*
* @param f1 first fraction (numerator=[0], denominator=[1])
* @param f2 second fraction (numerator=[0], denominator=[1])
* @return result of multiplying parameters (numerator=[0], denominator=[1])
*/
public static int[] multiplyFractions(int[] f1, int[] f2) {
return new int[2]; // TODO: replace with your code
}
/**
* Divides two fractions
*
* @param f1 first fraction (numerator=[0], denominator=[1])
* @param f2 second fraction (numerator=[0], denominator=[1])
* @return result of dividing parameter f2 by f1 (numerator=[0], denominator=[1])
*/
public static int[] divideFractions(int[] f1, int[] f2) {
return new int[2]; // TODO: replace with your code
}
public static void main(String[] args) {
// TODO: write your code here
}
}
Problem a (LA5a.java) Write a program that works with fractions. You are first to implement three methods, each to perform a different calculation on a pair of fractions: subtract, multiply, and divide. For each of these methods, you are supplied two fractions as arguments, each a two-dimensional array (the numerator is at index o, the denominator is at index 1), and you are to return a resulting, simplified fraction as a new two-dimensional array (again, with the numerator at index o, and denominator at index 1). You have been provided an add method as an example. You must compute the resulting fraction using fraction-based math (working with numerators and denominators) - do not convert the fractions to double values (like 1.5), do the math, and convert back to a fraction. You have been provided a method to simplify a fraction using the gcd method a previous last lab. As a reminder for fraction arithmetic... did did Once the operation methods are complete and pass the JUnit tests, now focus your attention on the main method. You first need to input the two fractions from the keyboard (numerator then denominator for each; you can assume integers) as well as one of the four valid operations (+, -, *, /) Then validate the inputs: make sure a valid operation was input, make sure neither of the denominators are zero, and make sure that the numerator of the second fraction isn't zero if the operation is division (error messages have been provided for each of these situations). Finally, compute the result of the operation and output the answer. Note that if the denominator of the answer is 1, you should just output the numerator (this includes if the answer is o). Here are two example runs of the program Enter the numerator for the first fraction: 1 Enter the denominator for the first fraction: 2 Enter the numerator for the second fraction -4 Enter the denominator for the second fraction: 8 Enter the operation (+, -,*, /): 1/2-4/80 Enter the numerator for the first fraction: 7 Enter the denominator for the first fraction: 8 Enter the numerator for the second fraction: 1 Enter the denominator for the second fraction: 3 Enter the operation (+, -,*, /): 7/8 1/313/24Step by Step Solution
There are 3 Steps involved in it
Step: 1
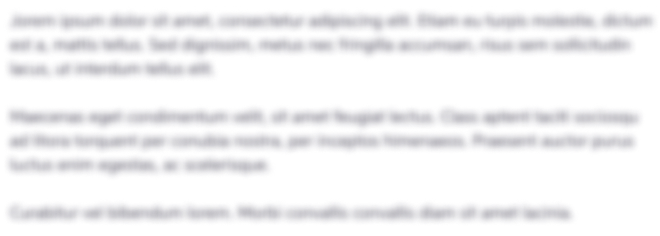
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started