Question
Java War Card Game (based on http://www.bicyclecards.com/how-to-play/war/): The game starts with two players who get half the deck of cards (each player obtaining 26 cards).
Java War Card Game (based on http://www.bicyclecards.com/how-to-play/war/): The game starts with two players who get half the deck of cards (each player obtaining 26 cards). Both Players flip over the top card from their pile and the player who flips the card with a higher value or rank wins and gets both cards. The winning player adds both cards to their own pile of cards and if both players flip over cards with the same value or rank, then a War starts. During a War, both players put four cards into a seperate pile and flip over the top card. The player who flips over the highest card wins the War pile. If the players both turn over the same card during a War, both play War again. The game then resumes as normal (flipping one card at a time). And the game ends when one player runs out of cards, the winner should have all the cards at the end of the game Classes must contain:
And the outputs should be:
I have succesfully implemented the code (see below)
Cards.java
public class Card{
//Initialize instance variables: private String suit; private String rank; private int value;
//Initialize constructor public Card(){ }
//Another constructor that creates a 'Card' object with the attributes based on user input: public Card(String suit, String rank){ this.suit = suit; this.rank = rank; //The following if-statements below will determine the value of the card based on the card's rank: if(rank.equals("2")){ value = 2; } else if(rank.equals("3")){ value = 3; } else if(rank.equals("4")){ value = 4; } else if(rank.equals("5")){ value = 5; } else if(rank.equals("6")){ value = 6; } else if(rank.equals("7")){ value = 7; } else if(rank.equals("8")){ value = 8; } else if(rank.equals("9")){ value = 9; } else if(rank.equals("10")){ value = 10; } else if(rank.equals("Jack")){ value = 11; } else if(rank.equals("Queen")){ value = 12; } else if(rank.equals("King")){ value = 13; } else if(rank.equals("Ace")){ value = 14; } //^ }
//Initialize getter methods: public String getSuit(){ return suit; } public String getRank(){ return rank; } public int getValue(){ return value; } //^
//Initialize setter methods: public void setSuit(String suit){ this.suit = suit; } public void setRank(String rank){ this.rank = rank; } public void setValue(int value){ this.value = value; } //^
public String toString(){ //toString is a method used to print out a Card object's attributes return rank + " " + suit; }
public Card copy(Card card){ //copy is a method which copies the attributes of a Card object to another created Card object Card temp = new Card (card.getSuit(), card.getRank()); return temp; }
public int isGreater(Card card){ //isGreater is a method which compares the value of 2 different Card objects. int temp; if (value > card.getValue()){ //If the current Card's value is greater than the Card being compared then temp is set to 1 temp = 1; } else if (value
Deck.java
import java.util.ArrayList; //Imports Array Lists import java.util.Random; //Imports the Randomizer public class Deck{
Random random = new Random(); //Initialize instance variables: private ArrayList
//Initialize constructor: public Deck(){ //Initialize the string to blank values to avoid initialization error messages: String suit = ""; String rank = ""; //The lines below are a combination of if-else statements and nested for-loops which completes a Deck by assigning their corresponding suites and ranks for(int x = 0; x 1 && y
public Card getFromShuffledDeck(int x){ //getFromShuffled is a method used when the user draws a Card from the shuffled deck if(!Shuffled.isEmpty()){ return Shuffled.get(x); } return null; }
public void shuffle(){ //shuffle is a method that takes all Card objects from the Normal deck and shuffles the card in a random order ArrayList
WarDemo.java
import java.util.Scanner; //Imports the Scanner import java.util.ArrayList; //Imports Array Lists public class WarDemo{ public static void main(String[] args){ Scanner scan = new Scanner(System.in); //Creates a new Scanner called 'scan' //Initialize String variables: String player1; String player2; String option; String roundwinner = ""; String gamewinner = ""; //Initialize integer variables: int temp; int play = 1; //Initialize Boolean variables: boolean winner; while (play != 0){ //This while-loop will run as long as the user decides to play (play value = 1) winner = false; //For the beginning of each game, winner values are set to false Deck deck = new Deck(); //Creates a new Deck object called deck deck.shuffle(); //Calls the method shuffle for the deck //The lines below create 3 Array Lists of type Card for both players' Deck and the War Deck ArrayList
warDeckTransfer(Deck1, Deck2, warDeck); //warDeckTransfer is a method which adds 4 cards of both players to the warDeck temp = warDeck.get(3).isGreater(warDeck.get(7));
//The lines below analyze which of the two players wins the War, the winner gains all cards from the War Deck: if(temp == 1){ //When player 1 wins.. while (warDeck.size() != 0) { Deck1.add(warDeck.get(0)); warDeck.remove(0); roundwinner = player1; } } else if (temp == 2){ //When player 2 wins.. while (warDeck.size() != 0){ Deck2.add(warDeck.get(0)); warDeck.remove(0); roundwinner = player2; } } //^ else{ //Otherwise, another War begins shift(warDeck); //Uses the method shift which move all the cards to the right temp = warDeck.get(3).isGreater(warDeck.get(7));
//The lines below analyze which of the two players wins the second War, the winner gains all cards from the War Deck: if (temp == 1){ //When player 1 wins.. while (warDeck.size() != 0){ Deck1.add(warDeck.get(0)); warDeck.remove(0); roundwinner = player1; } } else if (temp == 2){ //When player 2 wins.. while (warDeck.size() != 0){ Deck2.add(warDeck.get(0)); warDeck.remove(0); roundwinner = player2; } } //^ } System.out.println(roundwinner); endwar(); } if (Deck1.size() == 52){ //When Deck 1 has 52 cards, player 1 wins, loop terminates gamewinner = player1; winner = true; } if (Deck2.size() == 52){ //When Deck 2 has 52 cards, player 2 wins, loop terminates gamewinner = player2; winner = true; } }//Loop ends when there is a winner (winner = true)
//The lines below print out the expected results, and asks if the user wants to play again: System.out.println(gamewinner + " WINS! Congratulations"); System.out.print("Play again (y)? "); //The lines below scan for the user's response then proceeds to different options based on the response option = scan.nextLine().toLowerCase(); option = "" + option.charAt(0); while (!(option.equals("y") || option.equals("n"))){ //This while-loop continuously runs until the user has provided a valid response System.out.print("Invalid option. Please enter y or n: "); option = scan.nextLine().toLowerCase(); option = "" + option.charAt(0); } if (option.equals("y")){ //When the user decides to keep playing.. play = 1; System.out.println(); } else{ //When the user decides to terminate the game.. play = 0; } }//Loop ends when user decides to stop playing (play = 0) System.out.print("Thanks for playing! "); }
public static void war(){ //war is a method that prints out the War message System.out.println("************************************************WAR*******************************************"); }
public static void endwar(){ //endwar is a method that prints out the End War message System.out.println("************************************************END WAR***************************************"); }
public static void shift(ArrayList
However, I still need some help with formatting my output properly (see the required outputs), VS mine outputs here:
As you can see, the text alignment is way off. I think this can be done by using print formatting but I don't know how to implement it
Card Class You need to first create a Card Class. A Card object is made up of a suit (which are Hearts, Diamonds, Clubs, and Spades). Each suit has the same set of ranked cards: 2, 3, 4, 5, 6, 7, 8,9, 10, Jack, Queen, King, Ace. And each card has a value (eg, you could make Ace-14, King= 13, Queen= 12, Jack-11, then the numbered values e.g., "2" has a value of 2, "8" has a value of 8. The suit of the card doesn't matter in this game (that is only the rank and value of a card matter but you need to print out the suit in your output) The order of best to worst cards: Ace, King, Queen, Jack, 10, 9, 8, 7, 6, 5, 4, 3, 2. Nothing beats an Ace and a 2 doesn't beat anything Deck Class You will then create a Class called Deck. This class will store the Cards as an ArrayList (52 cards in total made up of 4 suites of cards) - a "deck" of cards. You will also need to have methods related to a deck of cards a way to create and populate the ArrayList with all the different Cards, get and set methods, and in particular a shuffle method. The shuffle method will reorder the cards in the ArrayList into a random order There are many ways that you can shuffle a deck of cards. You can search for an algorithm for shuffling (make sure you reference any source you sue) or you can use a simple approach of generating two random indices in the deck and swapping the cards several times (e.g., 52). Demo Class (play the game) In your Demo class, you will ask two players for their names, create a deck of cards and shuffle them. Then you will "deal" the cards into two hands (give each player their own pile of cards) so that each player has 26 cards (that is each player will have their own ArrayList with their pile of cards). Then play the game. This is an automated game, meaning once you start the game, the cards in each players hand (pile) will automatically keep flipping until a winner is found. Note, your display must be like the above. You can use the printf function to help you format strings so that the columns line up. See this source to help you format your printfStep by Step Solution
There are 3 Steps involved in it
Step: 1
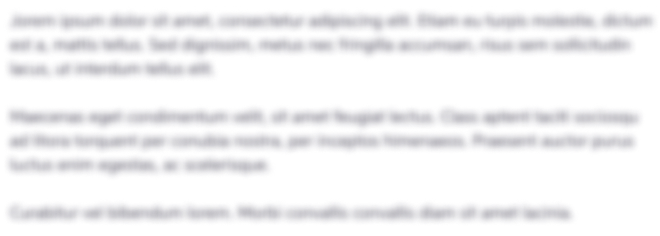
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started