Question
Java: Write a zip code lookup program. Read a data set of 1,000+ zip codes and city names from a file that contains zip codes
Java: Write a zip code lookup program. Read a data set of 1,000+ zip codes and city names from a file that contains zip codes and city names in Iowa in random order. Handle lookups by zip code and also reverse lookups by city name. Use a binary search for both lookups.
Four files are provided with the assignment.
1. ZipLookup.java: class containing main method. No modification needed.
2. Item.java: class to store an item. Use string variable key for zip code and string variable value
for city name. No modification needed.
3. LookupTable.java: core class of this assignment. Complete all methods.
4. iazip.txt: zip codes and city names in Iowa.
Submit one source file (LookupTable.java). In other words, ZipLookup.java, Item.java, or iazip.txt are not submitted
Ziplookup:
import java.io.IOException;
import java.io.FileReader;
import java.util.Scanner;
/* The input file has the format
50001
ACKWORTH
50002
ADAIR
50003
ADEL
50005
ALBION
50006
ALDEN
50007
ALLEMAN
50008
. . .
*/
public class ZipLookup
{
public static void main(String[] args) throws IOException
{
Scanner in = new Scanner(System.in);
System.out.println("Enter the name of the zipcode file: ");
String fileName = in.nextLine();
LookupTable table = new LookupTable();
FileReader reader = new FileReader(fileName);
table.read(new Scanner(reader));
boolean more = true;
while (more)
{
System.out.println("Lookup Z)ip, C)ity name, Q)uit?");
String cmd = in.nextLine();
if (cmd.equalsIgnoreCase("Q"))
more = false;
else if (cmd.equalsIgnoreCase("Z"))
{
System.out.println("Enter Zipcode:");
String n = in.nextLine();
System.out.println("City name: " + table.lookup(n) + " ");
}
else if (cmd.equalsIgnoreCase("C"))
{
System.out.println("Enter city name:");
String n = in.nextLine();
Item.java:
/**
An item with a key and a value.
*/
public class Item implements Comparable
{
private String key;
private String value;
/**
Constructs an Item object.
@param k the key string
@param v the value of the item
*/
public Item(String k, String v)
{
key = k;
value = v;
}
/**
Gets the key.
@return the key
*/
public String getKey()
{
return key;
}
/**
Gets the value.
@return the value
*/
public String getValue()
{
return value;
}
public int compareTo(Item otherObject)
{
Item other = (Item) otherObject;
return key.compareTo(other.key);
}
}
LookupTable.java:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
/**
* Code for HW6
* @author
*/
/**
A table for lookups and reverse lookups
*/
public class LookupTable
{
private ArrayList
private ArrayList
/**
Constructs a LookupTable object.
*/
public LookupTable()
{
byKey = new ArrayList
byValue = new ArrayList
}
/**
Reads key/value pairs.
@param in the scanner for reading the input
*/
public void read(Scanner in)
{
. . .
}
/**
Looks up an item in the table.
@param k the key to find
@return the value with the given key, or null if no
such item was found.
*/
public String lookup(String k)
{
. . .
}
/**
Looks up an item in the table.
@param v the value to find
@return the key with the given value, or null if no
such item was found.
*/
public String reverseLookup(String v)
{
. . .
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
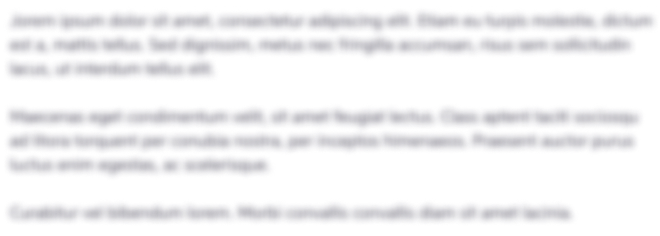
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started