Question
JAVA: you are required to implement a simplied network store-forward simulating program. You are required to set up your system with computers to emulate the
JAVA:
you are required to implement a simplied network store-forward simulating program. You are required to set up your system with computers to emulate the sender, receiver and router, which are connected as shown in the following figure:
The sender will send Y packets (changing Y from 10; 20; 40; 60; 80; 100) via the router to the receiver. The receiver will reply with corresponding acknowledge packet as in the following gure. The router in the middle will randomly drop a packet with a probability (10+X) %, where X is 4. We further assume that the router only randomly drop data packets, not acknowledge packets
You have to:
(a) Implement programs running on the sender, router, and the receiver by using of TCP transmission protocol
Here are the sample programs:
Reciever.java:
import java.io.IOException;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class TcpReceiver {
private static ServerSocket serverSocket;
private static final int PORT = 1240;
public static void main(String[] args)
{
System.out.println("Opening port ");
try
{
serverSocket = new ServerSocket(PORT);
/* Complete here */
}
catch(IOException ioEx)
{
System.out.println(
"Unable to attach to port for receiver!");
System.exit(1);
}
do
{
handleRouter();
}while (true);
}
private static void handleRouter()
{
Socket link = null;
try
{
link = serverSocket.accept();
/* Complete here */
}
catch(IOException ioEx)
{
ioEx.printStackTrace();
}
finally
{
try
{
System.out.println(
" * Closing connection *");
link.close(); //Step 5.
}
catch(IOException ioEx)
{
System.out.println(
"Unable to disconnect!");
System.exit(1);
}
}
}
}
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
Sender.java
//package org.test;
import java.io.*;
import java.net.*;
import java.util.*;
public class TcpSender {
private static InetAddress host;
private static final int PORT = 1241;
public static void main(String[] args)
{
try
{
host = InetAddress.getLocalHost();
}
catch(UnknownHostException uhEx)
{
System.out.println("Host ID not found!");
System.exit(1);
}
accessServer();
}
private static void accessServer()
{
Socket link = null;
try
{
link = new Socket(host,PORT);
/* Complete here */
}
catch(IOException ioEx)
{
ioEx.printStackTrace();
}
finally
{
try
{
System.out.println(" * Closing connection*");
link.close(); //Step 4.
}
catch(IOException ioEx)
{
System.exit(1);
}
}
}
}
%%%%%%%%%%%%%%%%%%%%%%%%%%%
Router.java
//package org.test;
import java.io.*;
import java.net.*;
import java.util.*;
public class TcpRouter
{
private static ServerSocket serverSocket;
private static InetAddress host;
private static final int PORT = 1241;
private static final int PORT2 = 1240;
private static Socket link2 = null;
private static int counter = 0;
private static int dropPacketSize = 0;
public static void main(String[] args)
{
System.out.println("Opening port");
{
try
{
Random roundInteger = new Random();
dropPacketSize = (int) Integer.valueOf(args[0])*14/100;
dropPacketSize += roundInteger.nextInt(2);
/* Complete here */
host = InetAddress.getLocalHost();
/* Complete here */
}
catch(UnknownHostException uhEx)
{
System.out.println("Host ID not found!");
System.exit(1);
}
}
try
{
serverSocket = new ServerSocket(PORT); //Step 1.
link2 = new Socket(host,PORT2);
/* Complete here */
}
catch(IOException ioEx)
{
System.out.println(
"Unable to attach to port for router!");
System.exit(1);
}
handleClient();
}
private static String handleClient()
{
Socket link = null;
try
{
link = serverSocket.accept();
/* Complete here */
}
catch(IOException ioEx)
{
ioEx.printStackTrace();
}
finally
{
try
{
System.out.println(
" * Closing connection*");
link.close(); //Step 5.
}
catch(IOException ioEx)
{
System.out.println(
"Unable to disconnect!");
System.exit(1);
}
}
return null;
}
}
Router Sender Receiver Figure 1: Network Transmission Model (Topology) Router Sender Receiver Figure 1: Network Transmission Model (Topology)Step by Step Solution
There are 3 Steps involved in it
Step: 1
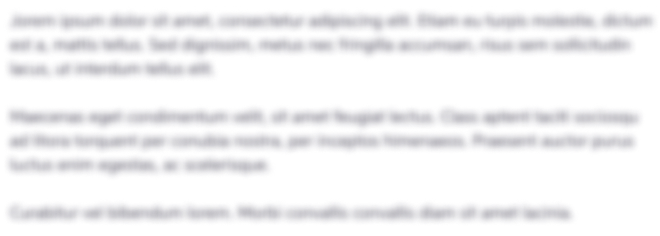
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started